Datalore
Collaborative data science platform for teams
A Complete Guide to Credit Risk Analysis With Python and Datalore AI
This is a guest blog post by Ryan O’Connell, CFA, FRM.
Understanding the relationships between various economic indicators is crucial for navigating the financial landscape. These relationships significantly impact the overall state of the economy, affecting businesses, investors, and individuals alike.
“In this tutorial, we’ll focus on the complex interplay between federal funds rates, 10-year Treasury yields, and corporate bond yields – key indicators that shape investment strategies, economic forecasts, and policy decisions.”
We’ll analyze data spanning over two decades of financial history, leveraging the power of Python, the Federal Reserve Economic Data (FRED) API, and Datalore’s AI-assisted coding capabilities. Python is particularly well-suited for this analysis due to its ability to efficiently pull and process large amounts of financial data through APIs. This approach allows for easy automation and replication of the analysis, offering a significant advantage over traditional spreadsheet methods.
Our goal is to uncover patterns, anomalies, and insights that illuminate the predictive power of yield curve changes on credit spreads. By using Python, we can create a workflow that’s not only powerful but also easily updatable for future analyses in just a few clicks.
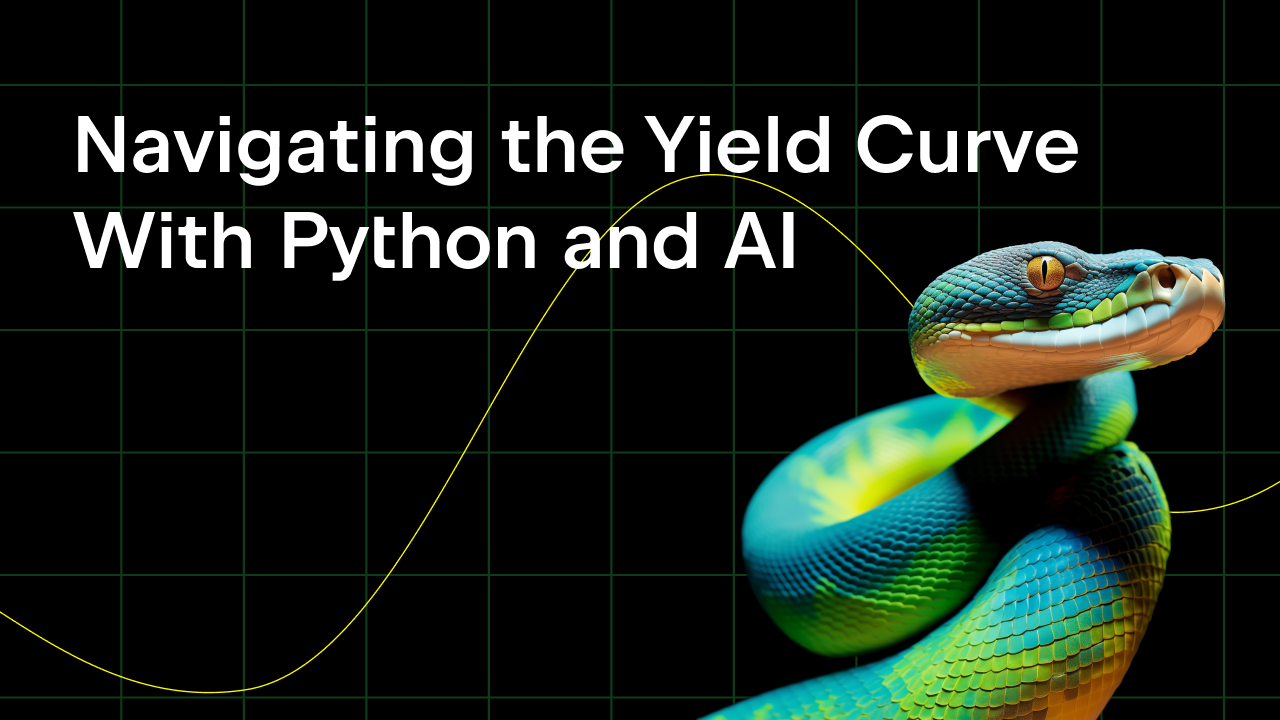
In this tutorial, you’ll learn how to:
- Set up your Python environment in Datalore for financial data analysis.
- Use Datalore’s AI Assistant to generate code for data retrieval and preprocessing.
- Create visualizations to analyze yield curve dynamics and credit spreads.
- Implement statistical analyses to explore the relationship between yield curves and credit risk.
- Leverage AI to help interpret results and generate insights.
Whether you’re a credit risk analyst looking to leverage more of Python within your stack or a curious newcomer to the world of credit risk, this tutorial will provide you with the tools and techniques to conduct your own in-depth analysis using Python and Datalore AI assistance.
Let’s dive in and start coding!
Disclaimer: This article is for informational and educational purposes only and is not intended to serve as personal financial advice.
The Yield Curve: A Financial Crystal Ball?
Before we dive into the data, let’s establish some context. The yield curve, particularly the spread between long-term and short-term interest rates, has long been considered a powerful predictive tool in finance. But why?
- Economic expectations: The yield curve reflects market expectations about future economic conditions. A normal, upward-sloping curve suggests optimism, while an inverted curve often signals pessimism.
- Monetary policy: It provides insights into the market’s view of future monetary policy decisions by central banks.
- Credit risk: The shape of the yield curve can influence lending behaviors and, consequently, credit risk in the economy.
Our analysis focuses on three key components:
- The federal funds rate: the short-term interest rate at which banks lend to each other overnight.
- The 10-year Treasury yield: a benchmark for long-term interest rates in the US.
- Corporate bond yields: representing the cost of borrowing for corporations.
By examining the relationships between these factors, we aim to uncover insights that could help predict changes in credit spreads and, by extension, credit risk in the market.
Setting up your environment and retrieving data
Before we dive into the analysis, you have two options to get started:
- Start a new Datalore notebook to follow along with this tutorial from scratch.
- If you have a Datalore account, you can click Edit copy on this Datalore report. This option allows you to see the full analysis and modify it as you go.
Whichever option you choose, we’ll need to set up our Python environment in Datalore and retrieve the necessary data. We’ll be using the Federal Reserve Economic Data (FRED) API to fetch historical data on federal funds rates, 10-year Treasury yields, and corporate bond yields.
Setting up your FRED API key
To use the FRED API, you’ll need to obtain an API key. Here’s how to set it up in Datalore:
- Go to the FRED API Key documentation and click on Request API Key.
- Register for an account or log in if you already have one.
- Once you have your API key, go to Environment in your Datalore workspace.
- Click on Environment Variables.
- Select + New Variable.
- Set up the variable as follows:
- Variable title: FRED_API_KEY
- Key: FRED_API_KEY
- Value: Your actual FRED API key
Installing required packages
In Datalore, you can easily install required packages. For this tutorial, we’ll need the fredapi package. To install it:
- Go to the Environment tab in Datalore.
- Click on Package Management.
- Search for ”fredapi” and click Install.
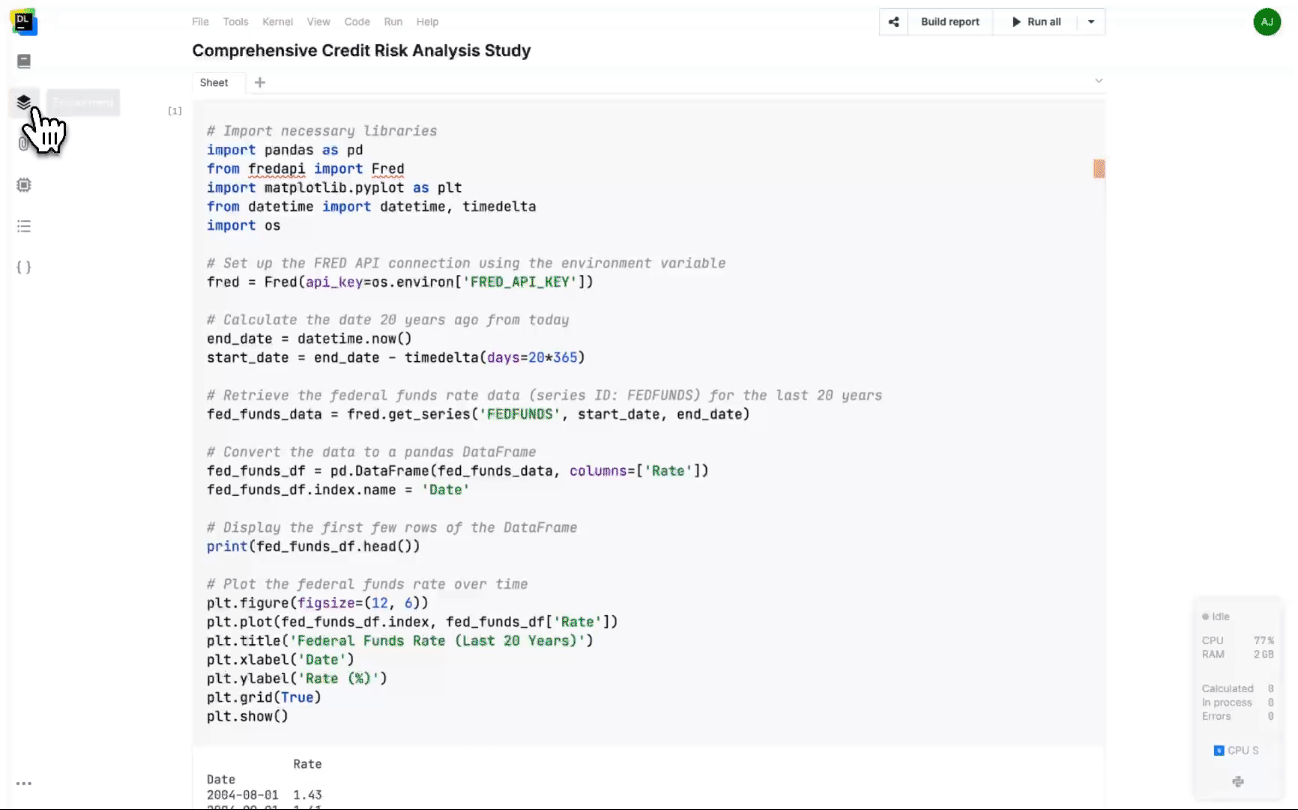
Retrieving fed funds rate data with AI assistance
Now, let’s use Datalore’s AI assistance to help us write code to retrieve and plot 20 years of federal funds rate data.
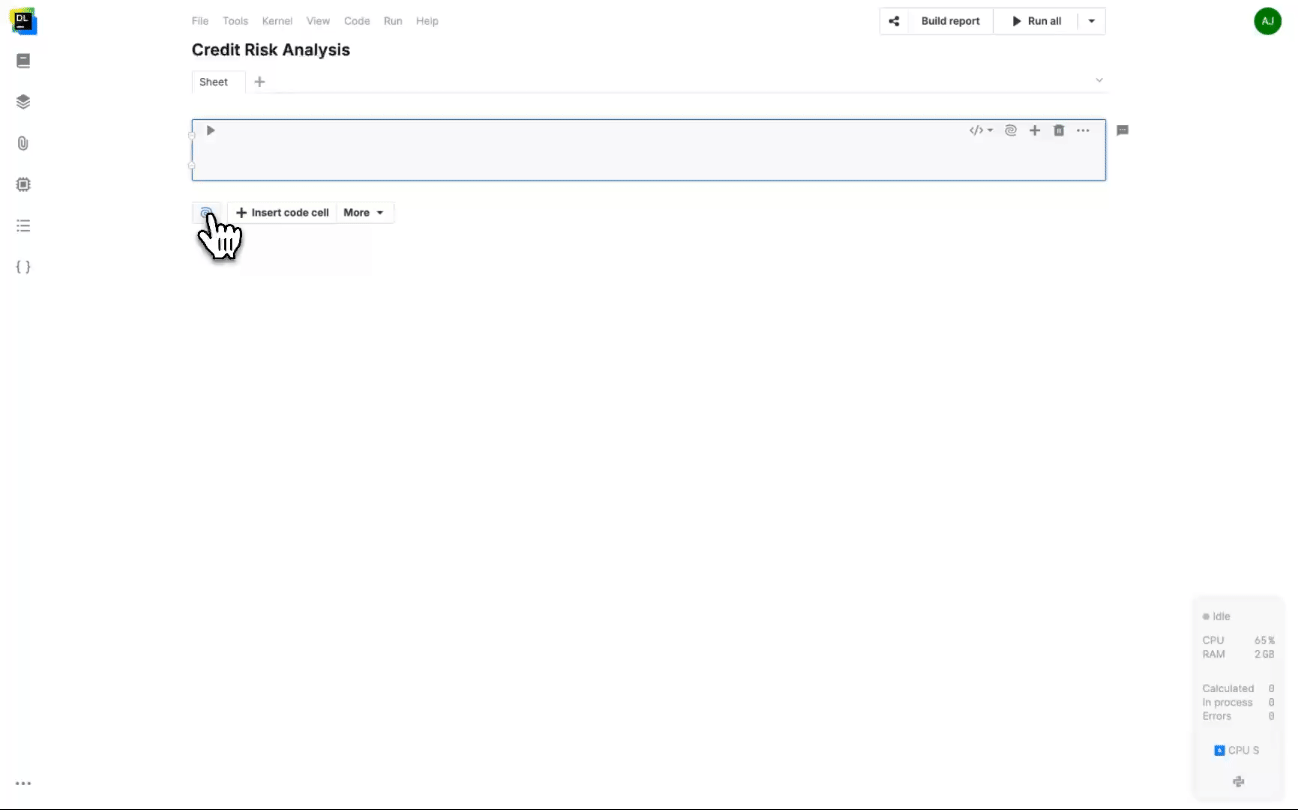
Here’s how you can prompt the AI:
AI Prompt |
Write Python code to retrieve the last 20 years of federal funds rate data using the FRED API. Please include the following steps: 1. Import necessary libraries (fredapi and pandas) 2. Set up the FRED API connection using the FRED_API_KEY environment variable 3. Retrieve the federal funds rate data (series ID: FEDFUNDS) for the last 20 years 4. Convert the data to a pandas DataFrame 5. Display the first few rows of the DataFrame 6. Plot the federal funds rate over time using matplotlib Note: Include comments explaining each step of the process. |
Here’s the code the AI might generate:
# Import necessary libraries import pandas as pd from fredapi import Fred import matplotlib.pyplot as plt from datetime import datetime, timedelta import os # Set up the FRED API connection using the environment variable fred = Fred(api_key=os.environ['FRED_API_KEY']) # Calculate the date 20 years ago from today end_date = datetime.now() start_date = end_date - timedelta(days=20*365) # Retrieve the federal funds rate data (series ID: FEDFUNDS) for the last 20 years fed_funds_data = fred.get_series('FEDFUNDS', start_date, end_date) # Convert the data to a pandas DataFrame fed_funds_df = pd.DataFrame(fed_funds_data, columns=['Rate']) fed_funds_df.index.name = 'Date' # Display the first few rows of the DataFrame print(fed_funds_df.head()) # Plot the federal funds rate over time plt.figure(figsize=(12, 6)) plt.plot(fed_funds_df.index, fed_funds_df['Rate']) plt.title('Federal Funds Rate (Last 20 Years)') plt.xlabel('Date') plt.ylabel('Rate (%)') plt.grid(True) plt.show()
The graph that this code plots can be seen below.
Federal funds rate: navigating two decades of monetary policy
Now that we’ve retrieved and plotted the data, let’s analyze the key trends and events visible in our graph:
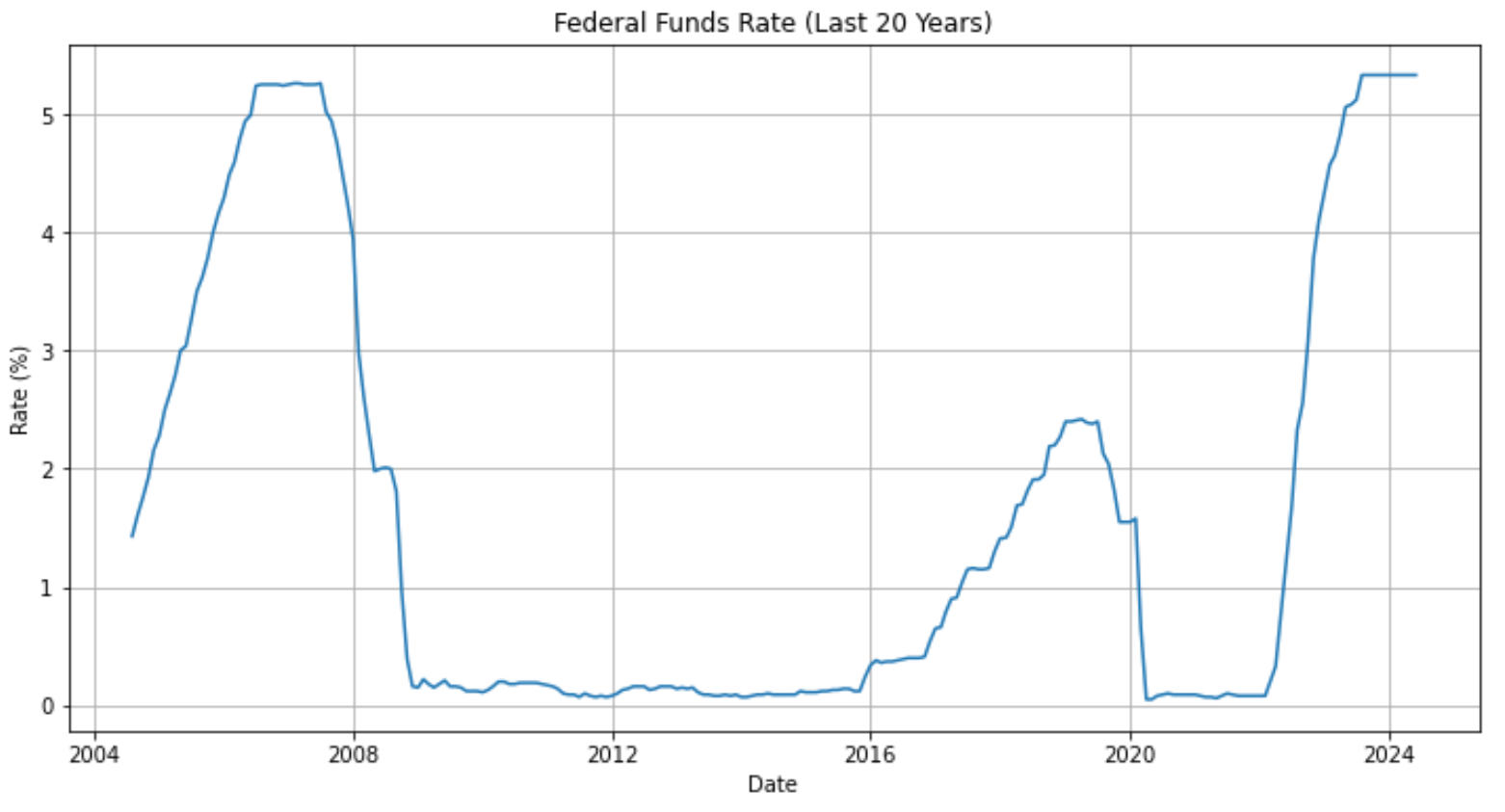
Key observations from our initial data exploration of the federal funds rate:
- Cyclical nature: The federal funds rate exhibits clear cyclical patterns over the 20-year period, reflecting the economic cycles and the Federal Reserve’s monetary policy responses.
- Pre-2008 financial crisis: From 2004 to 2006, we observe a steady increase in the rate, peaking at around 5.25% in 2006–2007. This period of rising rates was likely in response to a growing economy and inflationary pressures.
- 2008 financial crisis: The rate drops dramatically starting in 2007 and accelerates in 2008, coinciding with the Global Financial Crisis. The Fed rapidly cut rates to near zero in an attempt to stimulate the economy.
- Extended low-rate environment: From 2009 to 2015, we see an unprecedented period of near-zero interest rates, often referred to as the “zero lower bound” era. This reflects the Fed’s aggressive monetary policy to support economic recovery post-crisis.
- Gradual normalization: Starting in late 2015, there’s a gradual increase in rates as the Fed began to normalize monetary policy in response to improving economic conditions.
- COVID-19 pandemic impact: In early 2020, we observe another sharp drop to near-zero rates, mirroring the Fed’s emergency response to the economic shock of the COVID-19 pandemic.
- Recent rapid increase: From 2022 to 2023, we see a steep and rapid increase in rates, reaching levels not seen since before the 2008 crisis. This reflects the Fed’s aggressive tightening to combat high inflation.
These observations set the stage for our deeper analysis of how federal funds rate changes interact with other key indicators and influence credit risk. Next, we’ll retrieve Treasury yields and corporate bond rates.
Retrieving 10-year Treasury rate data with AI assistance
In this section, we’ll focus on retrieving historical data for 10-year US Treasury yields, a key indicator in financial markets. The 10-year Treasury yield is often used as a benchmark for other interest rates and is crucial for understanding broader economic trends.
We’ll use the FRED API to fetch 20 years of daily data, giving us a comprehensive view of long-term interest rate movements.
Let’s start by prompting the AI to write our data retrieval and visualization code. Here’s the prompt we’ll use:
AI Prompt |
Write Python code to retrieve the last 20 years of 10-year Treasury yields data using the FRED API. Please include the following steps: 1. Import necessary libraries (if not already imported) 2. Use the existing FRED API connection (assuming it’s already set up) 3. Retrieve the 10-year Treasury yields data (series ID: DGS10) for the last 20 years 4. Convert the data to a pandas DataFrame 5. Display the first few rows of the DataFrame 6. Plot the 10-year Treasury yields over time using matplotlib Note: Include comments explaining each step of the process. |
Here’s the code the AI might generate:
# Import necessary libraries (if not already imported) import pandas as pd from fredapi import Fred import matplotlib.pyplot as plt from datetime import datetime, timedelta import os # Use the existing FRED API connection fred = Fred(api_key=os.environ['FRED_API_KEY']) # Calculate the date 20 years ago from today end_date = datetime.now() start_date = end_date - timedelta(days=20*365) # Retrieve the 10-year Treasury yields data (series ID: DGS10) for the last 20 years treasury_10y_data = fred.get_series('DGS10', start_date, end_date) # Convert the data to a pandas DataFrame treasury_10y_df = pd.DataFrame(treasury_10y_data, columns=['Yield']) treasury_10y_df.index.name = 'Date' # Display the first few rows of the DataFrame print(treasury_10y_df.head()) # Plot the 10-year Treasury yields over time plt.figure(figsize=(12, 6)) plt.plot(treasury_10y_df.index, treasury_10y_df['Yield']) plt.title('10-Year Treasury Yields (Last 20 Years)') plt.xlabel('Date') plt.ylabel('Yield (%)') plt.grid(True) plt.show()
Now, let’s take a look at the plot of 20 years of long-term Treasury data.
10-year Treasury yields: two decades of fluctuations
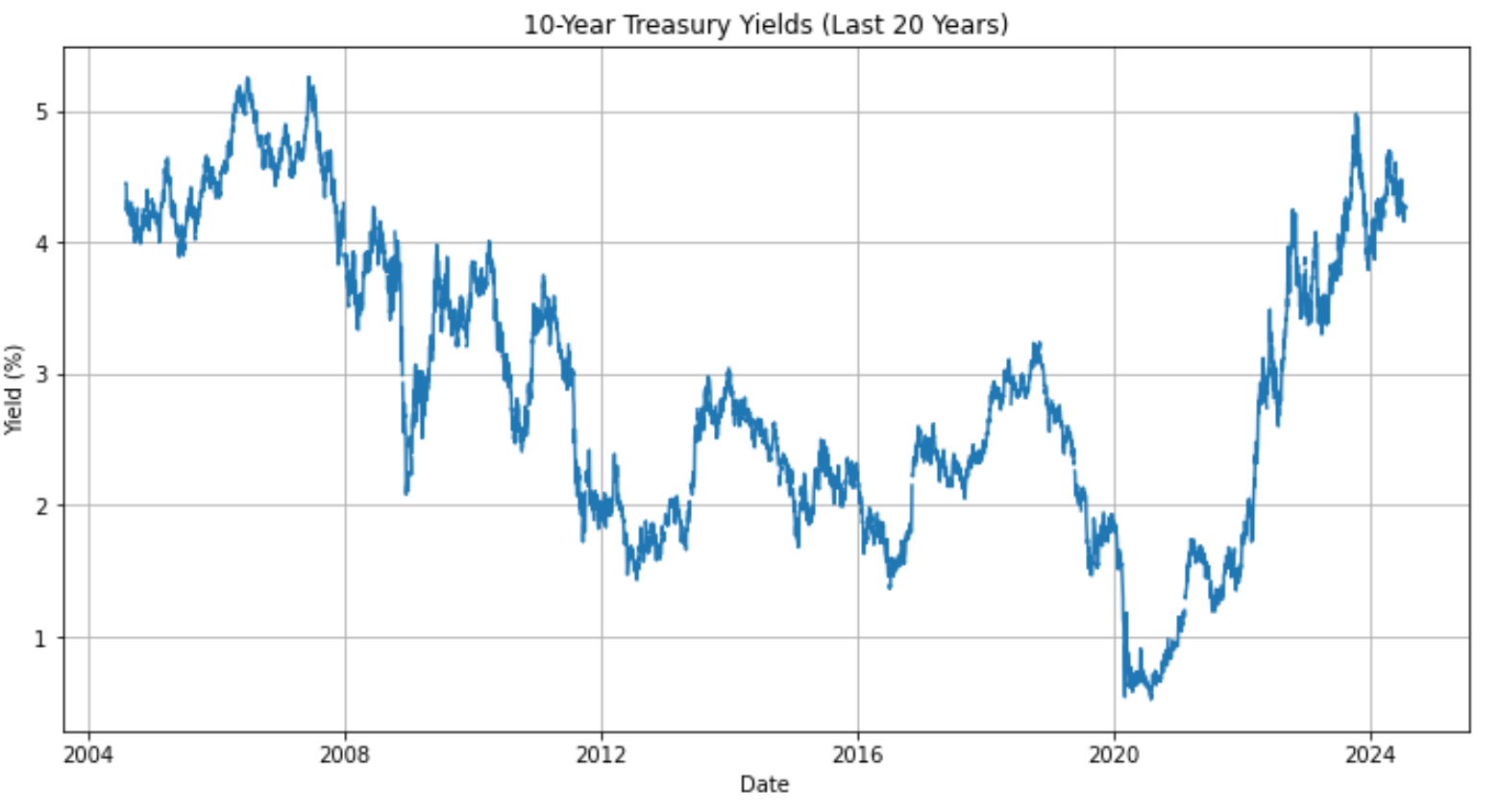
Now that we’ve retrieved and plotted the data, let’s analyze the key trends and events visible in our graph:
- 2008 financial crisis impact: There’s a sharp decline in yields starting in late 2007 and accelerating through 2008, mirroring the flight to safety during the financial crisis, though less dramatic than the federal funds rate drop.
- Post-crisis volatility and recovery: After the initial drop, yields showed significant volatility between 2009 and 2012, followed by a gradual increase until 2018, reflecting the uncertain economic recovery and the effects of changing monetary policies.
- COVID-19 pandemic shock: In early 2020, we observe a dramatic plunge in yields to historic lows below 1%, coinciding with the economic shock of the COVID-19 pandemic and aggressive Fed intervention.
- Recent rapid increase: From 2021 onwards, there’s a sharp upward trend in yields, reaching levels not seen since before the 2008 crisis, aligning with rising inflation concerns and the Fed’s tightening monetary policy.
These observations provide crucial context for understanding how both short-term and longer-term interest rates have behaved in response to economic conditions and policy changes over the past two decades. As we proceed, we’ll explore how these rate movements interact to shape the overall yield curve and influence credit risk in the market.
Retrieving corporate bond yields with AI assistance
Having examined the 10-year Treasury yields, we now turn to corporate bond yields, which provide insights into borrowing costs for corporations and serve as a key component in our credit risk analysis. We’ll use the ICE BofA US Corporate Index Effective Yield as our benchmark, offering a broad measure of US dollar-denominated investment-grade corporate debt performance.
Let’s use Datalore’s AI assistance to generate code that retrieves and visualizes 20 years of daily data from the FRED API. Here’s the prompt:
AI Prompt |
Write Python code to retrieve the last 20 years of corporate bond yield data using the FRED API. Please include the following steps: 1. Use the existing FRED API connection 2. Retrieve the ICE BofA US Corporate Index Effective Yield data (series ID: BAMLC0A0CMEY) for the last 20 years 3. Convert the data to a pandas DataFrame 4. Handle any missing values appropriately 5. Display the first few rows of the DataFrame 6. Plot the corporate bond yields over time using matplotlib Note: Include comments explaining each step of the process. |
My Datalore AI Assistant produced this code:
# Use the existing FRED API connection # Assuming fred is already defined and the API key is set up as an environment variable # Calculate the date 20 years ago from today end_date = datetime.now() start_date = end_date - timedelta(days=20*365) # Retrieve the ICE BofA US Corporate Index Effective Yield data (series ID: BAMLC0A0CMEY) for the last 20 years corporate_yield_data = fred.get_series('BAMLC0A0CMEY', start_date, end_date) # Convert the data to a pandas DataFrame corporate_yield_df = pd.DataFrame(corporate_yield_data, columns=['Yield']) corporate_yield_df.index.name = 'Date' # Handle missing values by forward filling (using the last known value) corporate_yield_df = corporate_yield_df.fillna(method='ffill') # Display the first few rows of the DataFrame print(corporate_yield_df.head()) # Plot the corporate bond yields over time plt.figure(figsize=(12, 6)) plt.plot(corporate_yield_df.index, corporate_yield_df['Yield']) plt.title('ICE BofA US Corporate Index Effective Yield (Last 20 Years)') plt.xlabel('Date') plt.ylabel('Yield (%)') plt.grid(True) plt.show()
In the next section, we will analyze the results of this code block.
Corporate bond yields: two decades of risk and reward
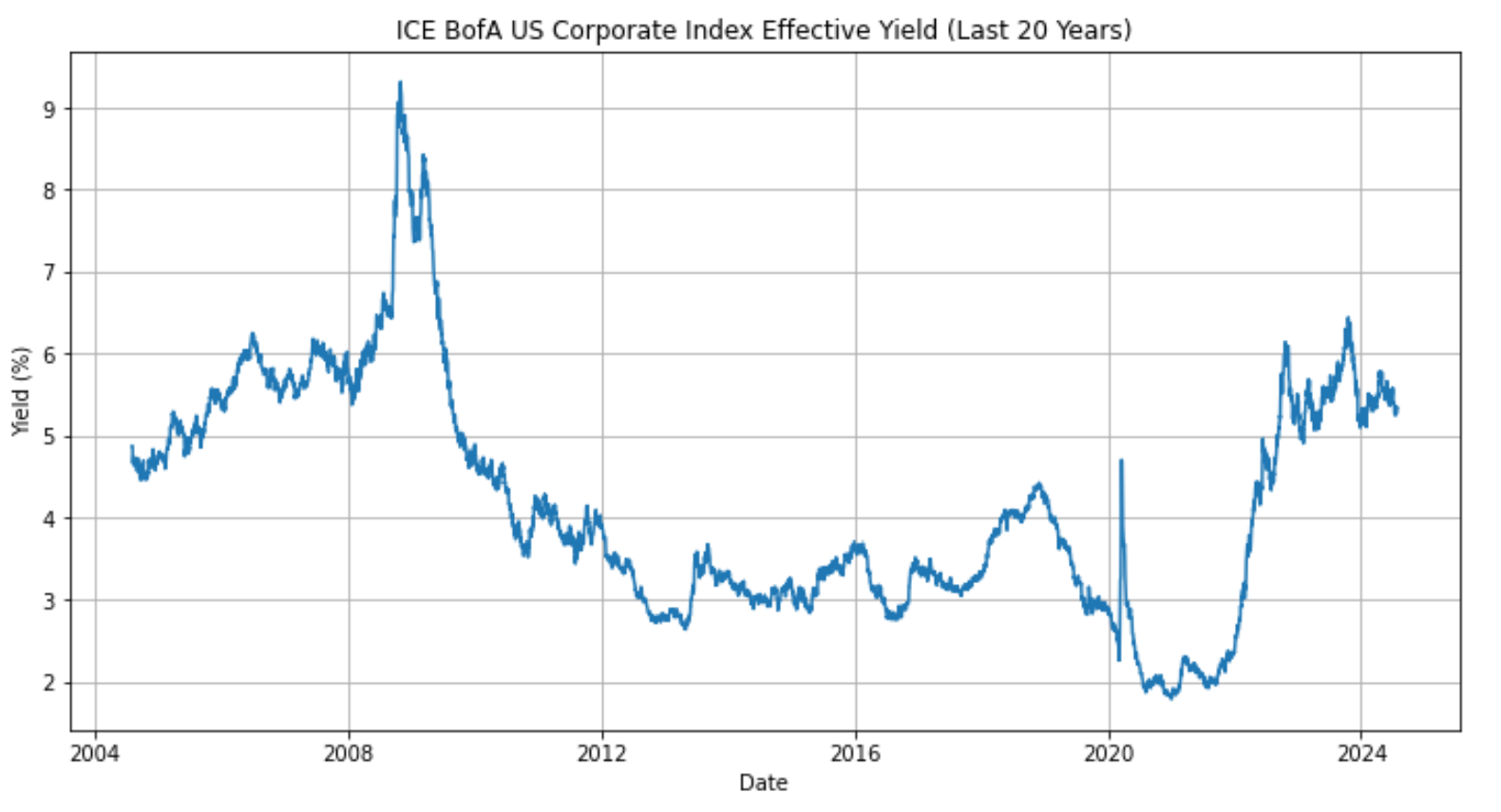
Now that we’ve retrieved and plotted the data, let’s analyze the key trends and events in corporate bond yields over the past 20 years:
- 2008 financial crisis spike: The most dramatic feature of the graph is the sharp spike in yields during the 2008 financial crisis, reaching a peak of over 9%. This reflects the extreme risk aversion and liquidity concerns in the corporate bond market during this period.
- Pre-crisis build-up: Leading up to the 2008 crisis, we observe a gradual increase in yields from 2004 to 2007, possibly indicating growing concerns about credit risk in the years preceding the financial crisis.
- Post-crisis decline and stabilization: Following the 2008 peak, yields declined rapidly and then stabilized, fluctuating between 3% and 4% for much of the period from 2010 to 2019. This reflects the impact of the Fed’s accommodative monetary policy and a general improvement in economic conditions.
- COVID-19 pandemic impact: In early 2020, we see another significant, though less extreme, spike in yields coinciding with the onset of the COVID-19 pandemic. This spike was quickly reversed, likely due to swift central bank intervention.
- Recent upward trend: From 2021 onwards, we observe an upward trend in yields, reaching levels not seen since before the 2008 crisis. This aligns with rising inflation concerns and tightening monetary policy, reflecting increased borrowing costs for corporations.
These observations provide valuable insights into how corporate borrowing costs and perceived credit risk have evolved over the past two decades. In the next section, we’ll explore how these corporate bond yields interact with the federal funds rate and Treasury yields to shape the overall credit risk landscape.
Fed Funds Rate vs. 10-year Treasury yield and spread analysis
After examining the federal funds rate and 10-year Treasury yields individually, we now turn our attention to the relationship between these two crucial indicators. The spread between these rates, often referred to as the yield curve slope, provides valuable insights into economic expectations and potential credit risk.
The yield curve spread is calculated as follows:
Yield Curve Spread = 10-Year Treasury Yield - Federal Funds Rate
A positive spread indicates a “normal” yield curve, generally associated with economic growth expectations. Conversely, a negative spread signals an inverted yield curve, which is often seen as a predictor of economic downturns.
Let’s use AI assistance to create a comprehensive line chart visualization that tracks these key elements. Here’s the prompt for the AI:
AI Prompt |
Plot monthly Fed Funds Rate, Corporate Bond Yields, and their spread: 1. Use ‘fed_funds_data’ for Fed Funds Rate 2. Retrieve Corporate Bond Yield data (BAMLC0A0CMEY) from FRED 3. Resample both to monthly frequency and align to common date range 4. Calculate spread (Corporate Bond Yield minus Fed Funds Rate) 5. Remove NaN values 6. Plot all three lines with different colors 7. Include legend, title, axis labels, and red dashed line at y=0 8. Show summary statistics |
Your AI assistant should produce code that looks something like this:
import pandas as pd import matplotlib.pyplot as plt # Resample both series to monthly frequency and align them fed_funds_monthly = fed_funds_data.resample('M').last() treasury_10y_monthly = treasury_10y_data.resample('M').last() # Create a DataFrame with both series, using the common date range start_date = max(fed_funds_monthly.index.min(), treasury_10y_monthly.index.min()) end_date = min(fed_funds_monthly.index.max(), treasury_10y_monthly.index.max()) df = pd.DataFrame({ 'Fed Funds Rate': fed_funds_monthly[start_date:end_date], '10-Year Treasury Yield': treasury_10y_monthly[start_date:end_date] }) # Calculate the spread df['Spread'] = df['10-Year Treasury Yield'] - df['Fed Funds Rate'] # Remove any rows with NaN values df = df.dropna() # Plot the data plt.figure(figsize=(12, 6)) plt.plot(df.index, df['Fed Funds Rate'], label='Fed Funds Rate', color='blue') plt.plot(df.index, df['10-Year Treasury Yield'], label='10-Year Treasury Yield', color='orange') plt.plot(df.index, df['Spread'], label='Spread', color='green') plt.title('Fed Funds Rate vs 10-Year Treasury Yield and Spread') plt.xlabel('Date') plt.ylabel('Rate (%)') plt.legend() plt.grid(True) # Add horizontal line at y=0 plt.axhline(y=0, color='r', linestyle='--') plt.show() # Print some summary statistics print("\nSummary Statistics:") print(df.describe())
In the next section, we will dive into the plotted results of this code to draw some conclusions about the economy over time.
Interpreting yield curve dynamics
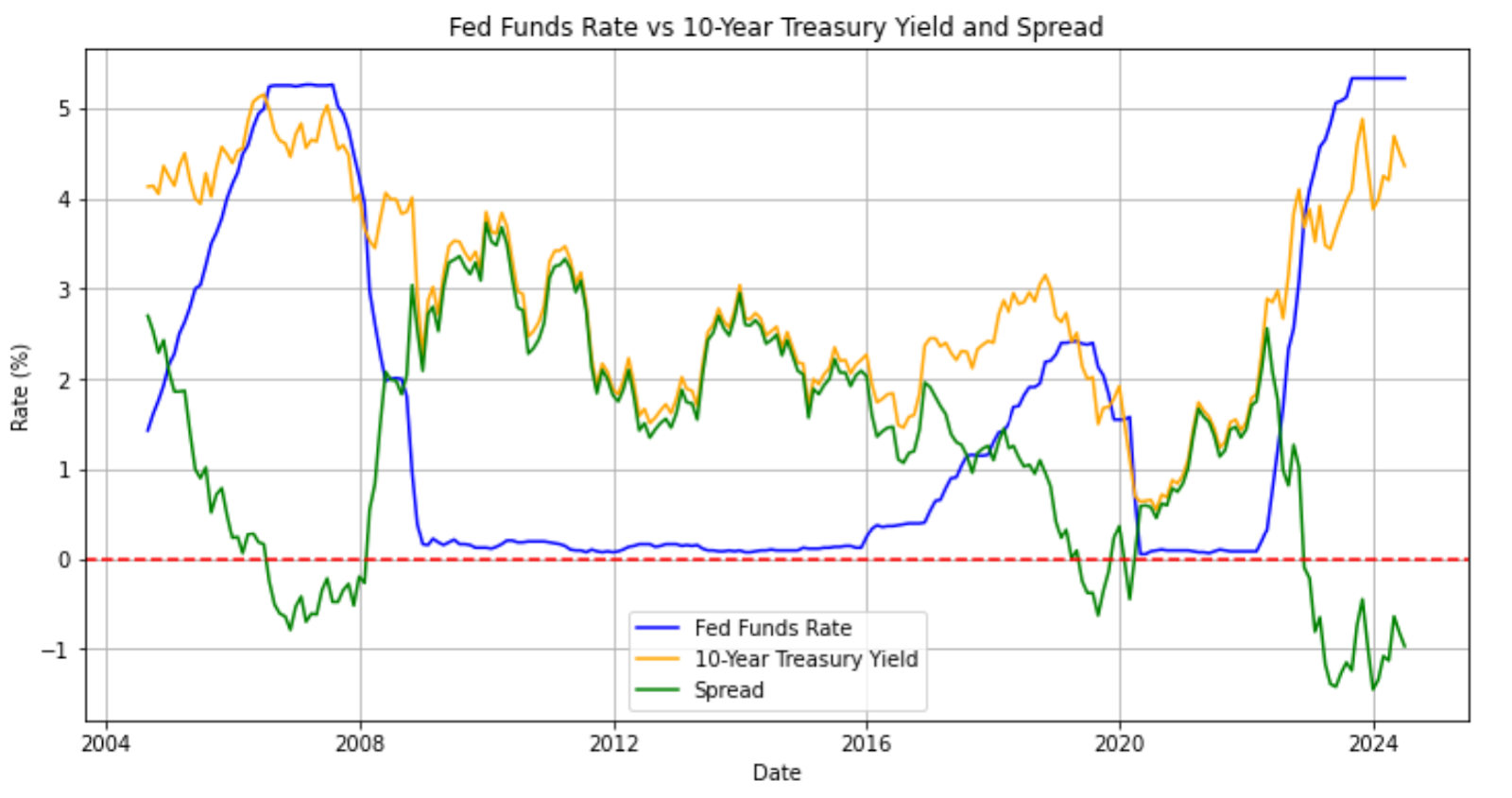
Now that we’ve visualized the data, let’s analyze the key trends and events in yield curve dynamics:
- Pre-2008 inversion: Leading up to the 2008 financial crisis, we observe a period of yield curve inversion (negative spread), with the fed funds rate exceeding the 10-year Treasury yield. This inversion is often seen as a predictor of economic recessions. In this particular case, the yield curve inversion was an accurate leading indicator.
- Post-crisis widening: Following the 2008 crisis, we see a significant widening of the spread as the Fed Funds Rate dropped dramatically while the 10-year Treasury Yield declined more gradually. This wide spread reflects the Fed’s aggressive monetary easing and attempts to stimulate long-term borrowing and investment.
- Extended period of positive spread: From 2009 to 2019, the spread remained consistently positive, with long-term rates higher than short-term rates. This “normal” yield curve is generally associated with expectations of economic growth.
- Recent inversions: We observe two notable periods of inversion in recent years – a brief inversion in 2019 and a more pronounced one starting in 2022. These inversions coincide with economic uncertainties, including trade tensions and inflation concerns.
- Pandemic impact and recovery: The spread widened sharply at the onset of the COVID-19 pandemic in 2020 as the Fed cut rates to near zero. Subsequently, we see a rapid narrowing and eventual inversion as the Fed aggressively raised rates to combat inflation. As of our analysis in 2024, we are currently experiencing an inverted yield curve, with short-term rates higher than long-term rates. Historically, such inversions have often preceded economic recessions, suggesting the possibility of an economic downturn in the coming years. However, it’s important to note that while yield curve inversions have been reliable predictors in the past, economic conditions can vary, and past performance doesn’t guarantee future outcomes.
These yield curve dynamics provide crucial context for understanding market expectations and potential economic turning points. The current inversion underscores the importance of monitoring these indicators closely in the coming months and years.
10-year Treasury yield vs. corporate bond yields analysis
In this section, we’ll explore a crucial relationship in fixed-income markets: the comparison between 10-year Treasury yields and corporate bond yields. This analysis is fundamental to understanding credit risk in the economy.
The spread between these yields, known as the credit spread, is calculated as:
Credit Spread = Corporate Bond Yield - 10-Year Treasury Yield
This spread represents the additional return investors demand for taking on the risk of lending to corporations rather than the US government. A widening spread typically indicates increased perceived risk, while a narrowing spread suggests improving conditions or increased risk appetite.
Let’s use AI assistance to create a visualization that includes:
- The 10-year Treasury yield.
- The corporate bond yield (using the ICE BofA US Corporate Index Effective Yield as a proxy).
- The spread between these rates.
Here’s the prompt for the AI:
AI Prompt |
Plot monthly 10-Year Treasury Yield, Corporate Bond Yields, and their spread: 1. Use ‘treasury_10y_data’ for 10-Year Treasury Yield 2. Retrieve Corporate Bond Yield data (BAMLC0A0CMEY) from FRED 3. Resample both to monthly frequency and align to common date range 4. Calculate spread (Corporate Bond Yield minus 10-Year Treasury Yield) 5. Remove NaN values 6. Plot all three lines with different colors 7. Include legend, title, axis labels, and red dashed line at y=0 8. Show summary statistics |
The code output should look like this:
import pandas as pd import matplotlib.pyplot as plt from fredapi import Fred import os from datetime import datetime, timedelta # Use the existing FRED API connection fred = Fred(api_key=os.environ['FRED_API_KEY']) # Calculate the date range end_date = datetime.now() start_date = end_date - timedelta(days=20*365) # Retrieve the corporate bond yield data corporate_yield_data = fred.get_series('BAMLC0A0CMEY', start_date, end_date) # Resample both series to monthly frequency treasury_10y_monthly = treasury_10y_data.resample('M').last() corporate_yield_monthly = corporate_yield_data.resample('M').last() # Create a DataFrame with both series, using the common date range start_date = max(treasury_10y_monthly.index.min(), corporate_yield_monthly.index.min()) end_date = min(treasury_10y_monthly.index.max(), corporate_yield_monthly.index.max()) df = pd.DataFrame({ '10-Year Treasury Yield': treasury_10y_monthly[start_date:end_date], 'Corporate Bond Yield': corporate_yield_monthly[start_date:end_date] }) # Calculate the spread df['Spread'] = df['Corporate Bond Yield'] - df['10-Year Treasury Yield'] # Remove any rows with NaN values df = df.dropna() # Plot the data plt.figure(figsize=(12, 6)) plt.plot(df.index, df['10-Year Treasury Yield'], label='10-Year Treasury Yield', color='blue') plt.plot(df.index, df['Corporate Bond Yield'], label='Corporate Bond Yield', color='orange') plt.plot(df.index, df['Spread'], label='Spread', color='green') plt.title('10-Year Treasury Yield vs Corporate Bond Yield and Spread') plt.xlabel('Date') plt.ylabel('Rate (%)') plt.legend() plt.grid(True) # Add horizontal line at y=0 plt.axhline(y=0, color='r', linestyle='--') plt.show() # Print some summary statistics print("\nSummary Statistics:") print(df.describe())
Now, let’s dive into the results of this analysis in the next section.
Interpreting credit spread dynamics
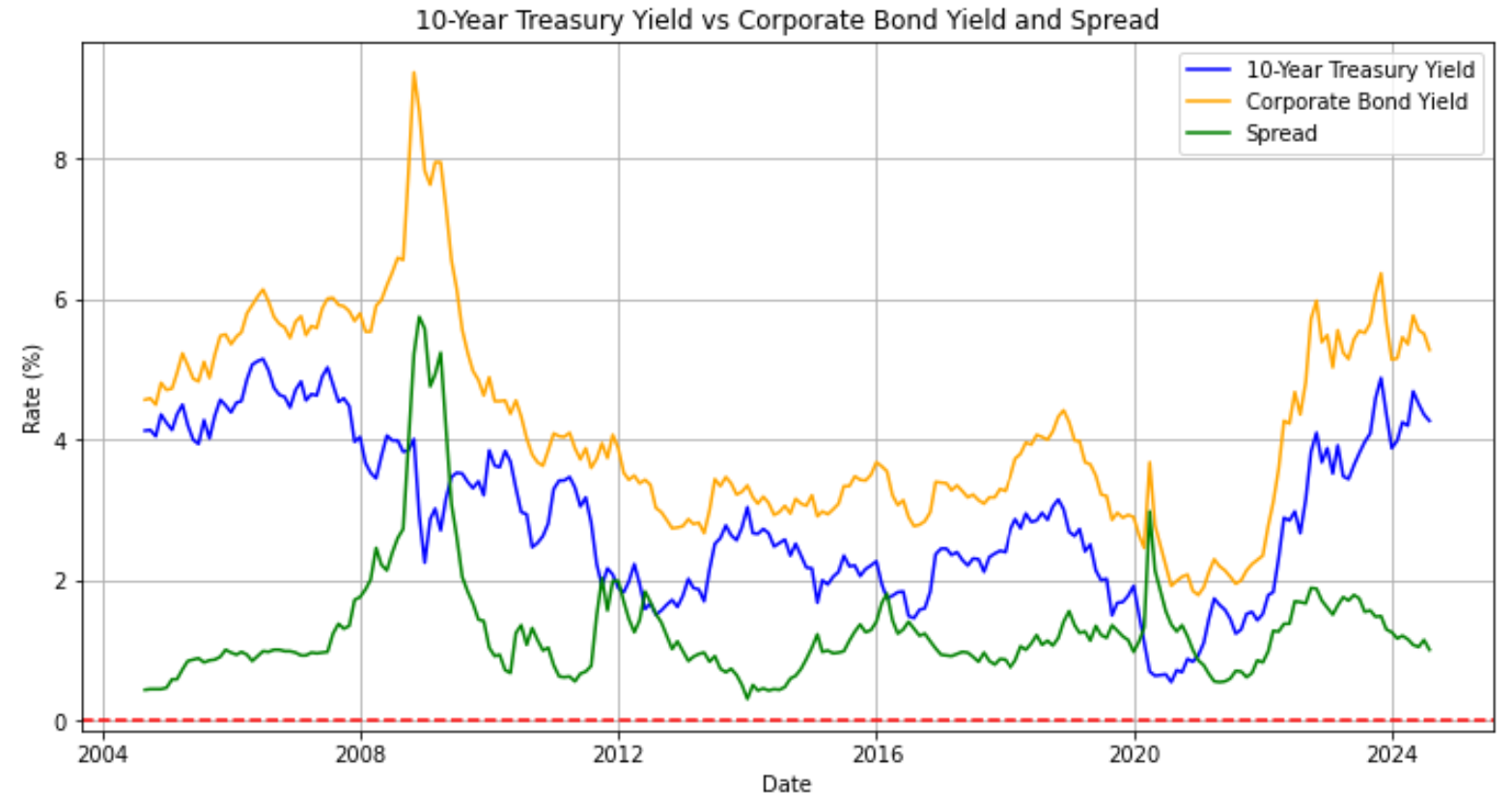
Now that we’ve visualized the data, let’s analyze the key trends in credit spread dynamics:
- 2008 financial crisis impact: The most striking feature of the graph is the dramatic widening of the spread during the 2008 financial crisis. This spike reflects a severe increase in perceived credit risk as investors fled to the safety of government bonds, demanding much higher yields for corporate debt.
- Pre-crisis build-up: In the years leading up to the 2008 crisis, we observe a gradual narrowing of the spread, suggesting increasing investor comfort with corporate credit risk. This trend reversed sharply with the onset of the crisis.
- Post-crisis normalization: Following the crisis, the spread gradually narrowed but largely remained elevated compared to pre-crisis levels for several years, indicating a more cautious approach to credit risk in the aftermath of the financial turmoil.
- COVID-19 pandemic spike: In early 2020, we see another significant, though less extreme, widening of the spread coinciding with the onset of the COVID-19 pandemic. This reflects the sudden increase in perceived corporate credit risk due to economic uncertainties.
- Recent trends: Since the pandemic spike, the spread has narrowed but remains above pre-pandemic levels, suggesting that while credit risk perceptions have improved, investors still maintain a degree of caution in the current economic environment.
These credit spread dynamics provide crucial insights for investors and policymakers. A widening spread often signals increasing economic stress and may precede broader market downturns. Conversely, a narrowing spread typically indicates improving conditions and increased confidence in corporate debt.
However, it’s important to consider credit spreads alongside other economic indicators for a comprehensive view of market conditions and credit risk. In the next section, we’ll explore how these insights can be applied to predictive analysis and risk management strategies.
Congratulations! You’ve made it this far into our tutorial. Want more? Read on to explore the relationship between yield curve changes and credit spreads.
[Advanced] Yield curve changes and credit spreads
In this section, we’ll explore the predictive relationship between yield curve changes and credit spreads. This analysis is crucial for understanding how changes in interest rates might signal future shifts in credit risk.
We’ll focus on two key questions:
- Do changes in the yield curve precede changes in credit spreads?
- Have yield curve inversions historically led to widening credit spreads?
Let’s use AI assistance to create a comprehensive Python script that analyzes these relationships:
AI Prompt |
Create a Python script to analyze yield curve slopes and credit spread changes: 1. Use FRED API for 40 years of: – Federal funds rate – 10-year Treasury yield – Corporate bond yield 2. Calculate: – Yield curve slope – Credit spread – Identify inversions 3. Analyze periods: 1, 7, 14, 30, 60, 90, 180, 270, 365, 547, 730, 1095 days For each: – Compute forward credit spread changes – Calculate correlations and mean changes (normal/inverted periods) – Perform t-tests (normal vs inverted) 4. Store results in DataFrame 5. Plot: – Correlation vs forward period – Mean changes vs forward period 6. Print results |
To check the resulting code, go to Datalore:
Let’s delve deeper into the relationship between yield curve slopes and future changes in credit spreads. Our goal is to understand how yield curve inversions might predict future spikes in credit risk and, by extension, potential economic downturns.
Methodology:
We analyzed the correlation between the yield curve slope (10-year Treasury yield minus fed funds rate) and forward changes in credit spreads (corporate bond yield minus 10-year Treasury yield) for various future time periods, ranging from 1 day to 3 years (1,095 days).
Analysis of correlation between yield curve slope and forward credit spread change
Key observations:
- Short-term correlations (1–90 days): We observe weak negative correlations, suggesting limited predictive power in the immediate future.
- Medium-term correlations (180–547 days): There’s a stronger negative relationship, with the peak negative correlation occurring around the 547-day mark. This indicates that yield curve inversions tend to precede credit spread widening most reliably in this time frame.
- Long-term correlation (1,095 days): Interestingly, the correlation turns positive, suggesting a potential reversal or cyclical nature of the relationship over very long periods.
Implications:
- The strongest predictive power of yield curve inversions for credit spread widening appears to be in the 1–2 year range.
- This aligns with the common observation that recessions often follow yield curve inversions by 12–24 months.
- The positive correlation at 1,095 days warrants further investigation and could relate to long-term economic cycles.
Analysis of mean forward credit spread change
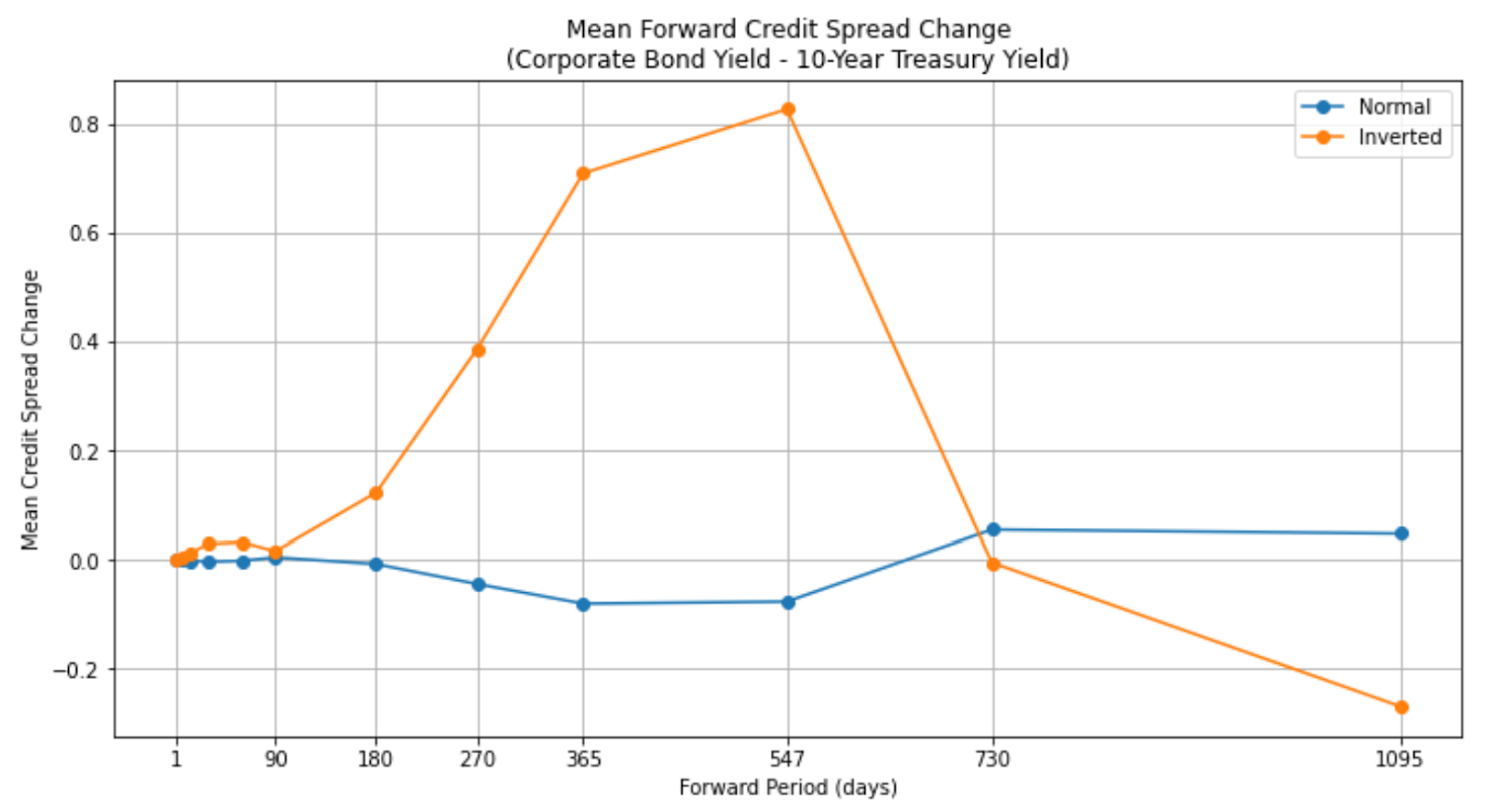
This graph illustrates the average change in credit spreads over various future time periods, comparing normal yield curve periods (blue line) to inverted yield curve periods (orange line).
Key observations:
- Normal yield curve periods (blue line):
- The line remains relatively flat and close to zero across all time periods.
- There’s a slight dip into negative territory for medium-term periods (180–547 days), suggesting a tendency for mild credit improvement during normal economic conditions.
- Long-term periods (730–1,095 days) show a small positive change, indicating a slight widening of credit spreads over extended time frames.
- Inverted yield curve periods (orange line):
- Short-term periods (1–90 days) show small positive changes, indicating slight immediate credit deterioration following inversions.
- Medium-term periods (180–547 days) exhibit large positive changes, peaking around the 547-day mark. This suggests significant credit spread widening in the 1–2 year period following yield curve inversions.
- Long-term periods (730–1,095 days) show a dramatic reversal, with the 1,095-day point dropping into negative territory. This indicates a potential improvement in credit conditions or a cyclical effect over very long time frames.
- Comparison between normal and inverted periods:
- The divergence between the two lines is most pronounced in the medium-term range (180–547 days), highlighting the predictive power of yield curve inversions for credit spread changes in this timeframe.
- The convergence and eventual crossover of the lines in the long term (1,095 days) is particularly interesting, suggesting a potential “mean reversion” effect over extended periods.
Implications:
- Timing of credit risk: The peak in the inverted line around 547 days suggests that the greatest credit risk following a yield curve inversion typically manifests between 1.5 to 2 years after the inversion occurs.
- Magnitude of impact: The large gap between normal and inverted lines in the medium term indicates that yield curve inversions precede significantly larger credit spread widenings compared to normal periods.
- Risk management strategies: Financial institutions and investors might consider implementing more conservative credit policies or increasing hedges against credit risk in the 1–2 year period following yield curve inversions.
- Economic cycle insights: The reversal seen at 1,095 days could indicate the typical length of a credit cycle or the delayed effects of policy interventions following economic stress periods.
- Predictive power: This graph reinforces the idea that yield curve inversions can serve as early warning indicators for increased credit risk, with a lead time of approximately 1–2 years.
While these patterns offer guidance, they should not be treated as infallible predictors. Economic conditions can vary, and past patterns may not always repeat in the same way. Nonetheless, this analysis offers a framework for understanding the relationship between yield curve dynamics and future credit risk, providing a valuable tool for economic forecasting and risk management.
[Advanced] Credit spread analysis with economic events: a 90-day forward look
In this final section, we’ll explore the dynamic relationship between credit spreads and major economic events, focusing on a 90-day forward-looking perspective. This analysis provides crucial insights into how credit risk perceptions evolve over relatively short periods, offering a window into market sentiment and economic conditions.
We’ll use AI assistance to create a comprehensive Python script that combines data manipulation, statistical analysis, and advanced visualization techniques. Our analysis will:
- Calculate 90-day forward changes in credit spreads.
- Identify and visualize outliers in credit spread changes.
- Map these changes to significant economic events from 2001 to 2020.
- Compare credit spread behavior during normal and inverted yield curve periods.
- Conduct statistical tests to validate our observations.
Let’s prompt the AI to help us create this analysis:
AI Prompt |
Create a Python script to analyze and visualize 90-day forward credit spread changes in relation to major economic events: 1. Use existing DataFrame ‘df’ with credit spread data 2. Calculate 90-day forward change in credit spreads: diff(periods=90).shift(-90) 3. Identify outliers using IQR method 4. Create a time series plot: – X-axis: Date (show every 5 years) – Y-axis: 90-day forward change in credit spread – Plot main line for spread changes – Highlight outliers as red scatter points 5. Add vertical lines for economic events (2001-2020): – Enron Bankruptcy, WorldCom Bankruptcy, Lehman Brothers Bankruptcy – European Debt Crisis, 2019 Repo Spike, COVID-19 Pandemic Declaration 6. Customize plot: large fonts, clear labels, grid, legend 7. Print summary statistics: – Number and percentage of outliers – Top 10 positive and negative outliers – Mean spread change for normal and inverted yield curve periods 8. Perform t-test comparing normal and inverted periods Use pandas for data manipulation, matplotlib for plotting, seaborn for styling, and scipy.stats for the t-test. Ensure the script is well-commented and follows Python best practices. |
To check the generated code, go to Datalore:
Let’s dive into the results of this code and get a unique look at the history of the United States through the lens of credit spread changes.
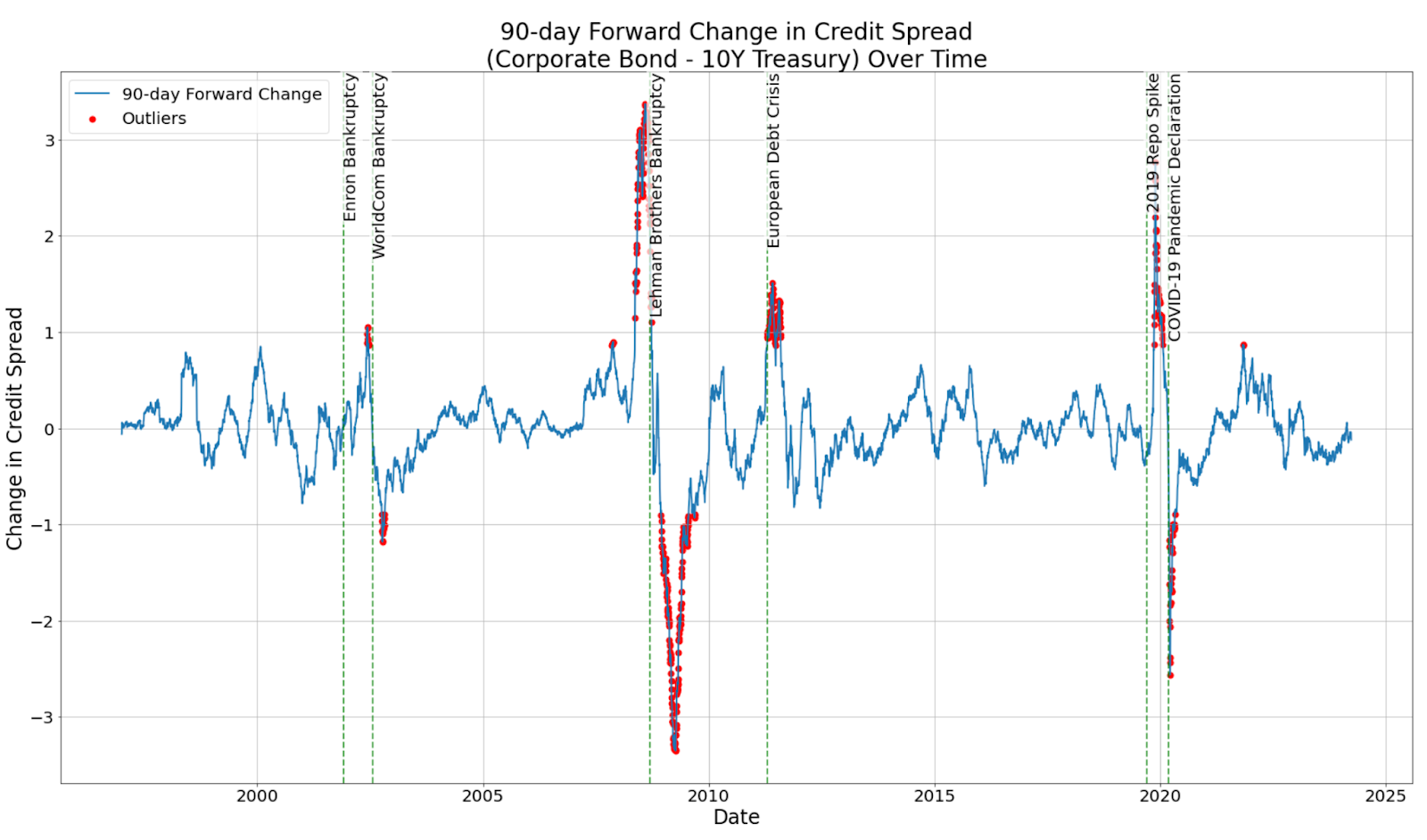
Now that we’ve visualized the data and generated our statistics, let’s analyze the key trends and events:
- Volatility clusters: The graph reveals distinct periods of high volatility in credit spread changes, particularly around major economic events. This clustering of volatility suggests that credit risk perceptions can change rapidly and dramatically during times of economic uncertainty.
- Asymmetric outliers: We observe that the most extreme outliers (red dots) tend to be on the positive side, indicating sudden, large increases in credit spreads. This asymmetry suggests that credit risk can spike more sharply than it tends to decrease, reflecting the market’s sensitivity to negative news.
- Major economic events:
- Dot-com bubble (2000–2002): We see increased volatility and some outliers, reflecting the tech market crash and subsequent economic uncertainty.
- 2008 financial crisis: The most dramatic spike in our dataset occurs around the Lehman Brothers bankruptcy, with extreme positive outliers indicating a severe credit crunch.
- European debt crisis (2011): Another cluster of outliers is visible, showing how international events can impact US credit markets.
- COVID-19 pandemic (2020): A sharp spike in credit spread changes, rivaling the 2008 crisis in magnitude, demonstrates the immediate and severe impact of the pandemic on credit risk perceptions.
- Recovery patterns: Following each major spike, we observe periods of negative changes in credit spreads, indicating a gradual return to lower credit risk perceptions. However, the recovery patterns vary in duration and stability.
- Recent trends: In the years leading up to 2020, we see relatively stable credit spread changes with fewer outliers, possibly reflecting the prolonged period of low interest rates and economic growth. The COVID-19 pandemic disrupts this stability dramatically.
- Frequency of outliers: Outliers appear to be more frequent and extreme during recognized periods of economic stress, serving as a visual indicator of market turmoil.
Limitations:
While this 90-day forward view provides valuable insights, it doesn’t capture longer-term trends or very short-term fluctuations. The causes behind these changes can be complex and multifaceted, often requiring additional context to fully interpret.
This analysis offers a nuanced view of credit risk dynamics over the past two decades. It highlights the market’s sensitivity to major economic events and provides a valuable tool for anticipating potential shifts in credit risk perceptions.
Conclusion: applying your new credit risk analysis skills
Congratulations on completing this comprehensive tutorial on credit risk analysis using Python and AI-assisted coding in Datalore! Let’s recap what you’ve learned and how you can apply these skills in your own financial analyses:
Key skills acquired
- Data retrieval and preprocessing: You’ve learned how to use the FRED API to fetch economic data and prepare it for analysis using pandas.
- Visualization techniques: You’ve created various plots to visualize federal funds rates, Treasury yields, and corporate bond yields, enhancing your data presentation skills.
- Yield curve analysis: You’ve explored how to calculate and interpret yield curve slopes and inversions, crucial indicators for economic forecasting.
- Credit spread calculation: You’ve computed and analyzed credit spreads, gaining insights into market risk perception.
- Predictive analysis: You’ve conducted forward-looking analyses to understand the relationship between yield curve changes and future credit spread movements.
- Event impact assessment: You’ve examined how major economic events affect credit spreads, developing skills in contextual data interpretation.
- AI-assisted coding: Throughout the tutorial, you’ve leveraged AI to generate code, demonstrating how to use this powerful tool to streamline your workflow.
Applying your skills
- Custom analyses: Use the code templates provided to analyze different time periods or other economic indicators that interest you.
- Risk management: Apply the yield curve inversion and credit spread analysis techniques to assess potential risks in your investment portfolio.
- Economic forecasting: Utilize the predictive analysis methods to create your own forecasts for credit risk and economic conditions.
- Data-driven decision making: Incorporate these data analysis techniques into your financial decision-making processes, whether for personal investments or professional applications.
- Continuous learning: As you’ve seen, economic conditions evolve. Use the skills you’ve learned to stay updated on market trends and continually refine your analysis techniques.
Next steps
- Expand your dataset: Try incorporating additional economic indicators or international data to broaden your analysis.
- Enhance your models: Experiment with more advanced statistical methods or machine learning techniques to improve predictive power.
- Automate your analyses: Create scripts that automatically update your analyses as new data becomes available with Datalore’s Scheduled runs feature.
- Collaborate and share: Use Datalore’s collaboration features to work with others and share your insights.
While these tools and techniques are powerful, they should be used in conjunction with a nuanced understanding of economic principles and current market conditions. Stay curious, keep practicing, and continue to refine your credit risk analysis skills!
If you’d like to edit the full code of this tutorial in Datalore, including interactive elements and additional resources, click the button below:
Subscribe to Datalore News and Updates
- The Yield Curve: A Financial Crystal Ball?
- Setting up your environment and retrieving data
- Setting up your FRED API key
- Installing required packages
- Retrieving fed funds rate data with AI assistance
- Federal funds rate: navigating two decades of monetary policy
- Retrieving 10-year Treasury rate data with AI assistance
- 10-year Treasury yields: two decades of fluctuations
- Retrieving corporate bond yields with AI assistance
- Corporate bond yields: two decades of risk and reward
- Fed Funds Rate vs. 10-year Treasury yield and spread analysis
- Interpreting yield curve dynamics
- 10-year Treasury yield vs. corporate bond yields analysis
- Interpreting credit spread dynamics
- [Advanced] Yield curve changes and credit spreads
- [Advanced] Credit spread analysis with economic events: a 90-day forward look
- Conclusion: applying your new credit risk analysis skills