The Angular Language Server: Understanding IDE Integration Approaches
The Language Server Protocol (LSP) has been a fundamental part of the code editor landscape for years, providing a consistent development experience across different editors. The Angular Language Server leverages this protocol to provide Angular-specific features to compatible editors. However, not all IDEs take the same approach to delivering these capabilities. Let’s look at the major differences between VS Code, NeoVim, and WebStorm and finally answer the age-old question, “Why does WebStorm not just use the Angular Language Server?!”
What is a language server, and how does the LSP work?
Modern code editors need to understand your code to provide features like autocompletion, go to definition, or error detection. Traditionally, each editor needed to implement this understanding for every programming language, leading to duplicated effort and inconsistent experiences across editors.
The Language Server Protocol (LSP) solves this by standardizing how editors communicate with language-specific analysis tools. Here’s how it works:
- Your editor (the client) sends information about your code (typically the file that is currently open in your editor) to a language server.
- The language server analyzes the code and responds with insights.
- Your editor displays these insights as squiggly error lines, autocompletion popups, or other UI elements.
// When you type this in your editor @Component({ template: ` <div *ngFor="let item of items"> {{ item.property }} </div> ` }) class MyComponent { }
Your editor sends the file content to the language server. The server analyzes it and might respond with:
{ "diagnostics": [{ "message": "Property 'property' does not exist on type 'any'", "range": { "start": {"line": 3, "character": 12}, "end": {"line": 3, "character": 20} } }] } // Your editor then shows the error underline
This standardization means that:
- Developers of editors and IDEs can add support for new languages just by implementing the LSP client once.
- Language tool developers can support all LSP-compatible editors with a single server and very small client implementations (JetBrains IDE plugin or VS Code plugin).
- Developers get consistent features across different editors.
The Angular Language Server specifically provides Angular-aware code analysis, offering features like template type checking, component property completion, and Angular-specific refactorings to any editor that supports LSP.
Benefits of the Language Server Protocol
The LSP has established itself as the standard for providing editor intelligence across different development environments. It enables consistent features like type checking, code completion, and navigation across any LSP-compatible editor:
@Component({ template: ` <div *ngFor="let item of items"> {{ item?.deeply?.nested?.property }} </div> ` }) class WhyTypeCheckingMatters { }
While the Angular compiler would catch these template errors during build time, the language server provides immediate feedback in your editor. This means you can spot and fix type errors as you write your code, without waiting for the compilation step.
VS Code: LSP’s golden child
VS Code’s integration with the Angular Language Server is seamless and intuitive. Here are some of the features that you’ll be able to use after installing the necessary plugin:
- Template type checking: Because nobody likes finding out about undefined properties in production.
- Go To Definition: Jump straight to your TypeScript references.
- Autocompletion: For when you can’t remember the syntax of
*ngFor
or@switch
. - Real-time error detection: Catch those typos before they catch you out.
The best part? It’s all standardized. The same language server that powers VS Code can power any editor that speaks LSP.
Neovim: for when Vim users want nice things, too
Remember when Vim users had to type out every import statement manually? Pepperidge Farm remembers. Now, thanks to LSP, you get:
-- Neovim config that actually gives you IDE features require'lspconfig'.angularls.setup{ -- Look ma, real autocomplete! }
Neovim users now enjoy the same powerful features as VS Code users while keeping their beloved modal editing. It’s the best of both worlds – modern IDE features with an outstanding editing experience.
The caveats of language servers
Language servers are great – they provide a consistent developer experience and guarantee correctness. However, the fact that language servers adhere to a standardized protocol means that they do have some limitations. For instance, even though most languages and frameworks have some kind of test support, the LSP is not aware of this concept, and, therefore, tests must be configured manually within the editor. This is also the case when working with debuggers, too.
Additionally, language servers generally look at the codebase on a “file by file” basis, and, more specifically, at the current cursor position. Generally speaking, this works great until it doesn’t… Implementing a multi-file refactoring, for instance, can be difficult (though not impossible). For this reason, extracting some HTML code into a new Angular component is not as straightforward as it might first sound. On top of that, language servers can usually only process one language at a time; therefore, refactoring a CSS class across languages can quickly become very cumbersome in a language server implementation. The Angular Language Server has a very clever trick for handling HTML and TypeScript, but more on that in the next section. CSS, on the other hand, doesn’t enjoy the same level of support.
What’s more, adding further support for parts that don’t have dedicated compiler functionality is difficult to accomplish. One such example would be IntelliSense for the host property of the component decorator.
WebStorm: a slightly different approach
Now, here’s where things get interesting. WebStorm and other JetBrains IDEs take a different approach, and it’s not just about being contrarian. For the last few years, the Angular plugin has been pre-installed with WebStorm. As of now, this plugin does not utilize the Angular Language Server, but a custom-type engine. As part of our new Service-powered type engine (more about this in the future), we made some major changes to our Angular integration. Instead of using the entire Angular Language Server, WebStorm adopted Angular’s TCB (type-check block) engine, an integral part of the Angular Language Server. For more details, you can check out this talk at NG DE, but in short, the Angular Language Server converts the HTML code into TypeScript code and uses the generated TypeScript code to map errors and IntelliSense features back to the cursor position in the original HTML file. The screenshot below visualizes this concept.
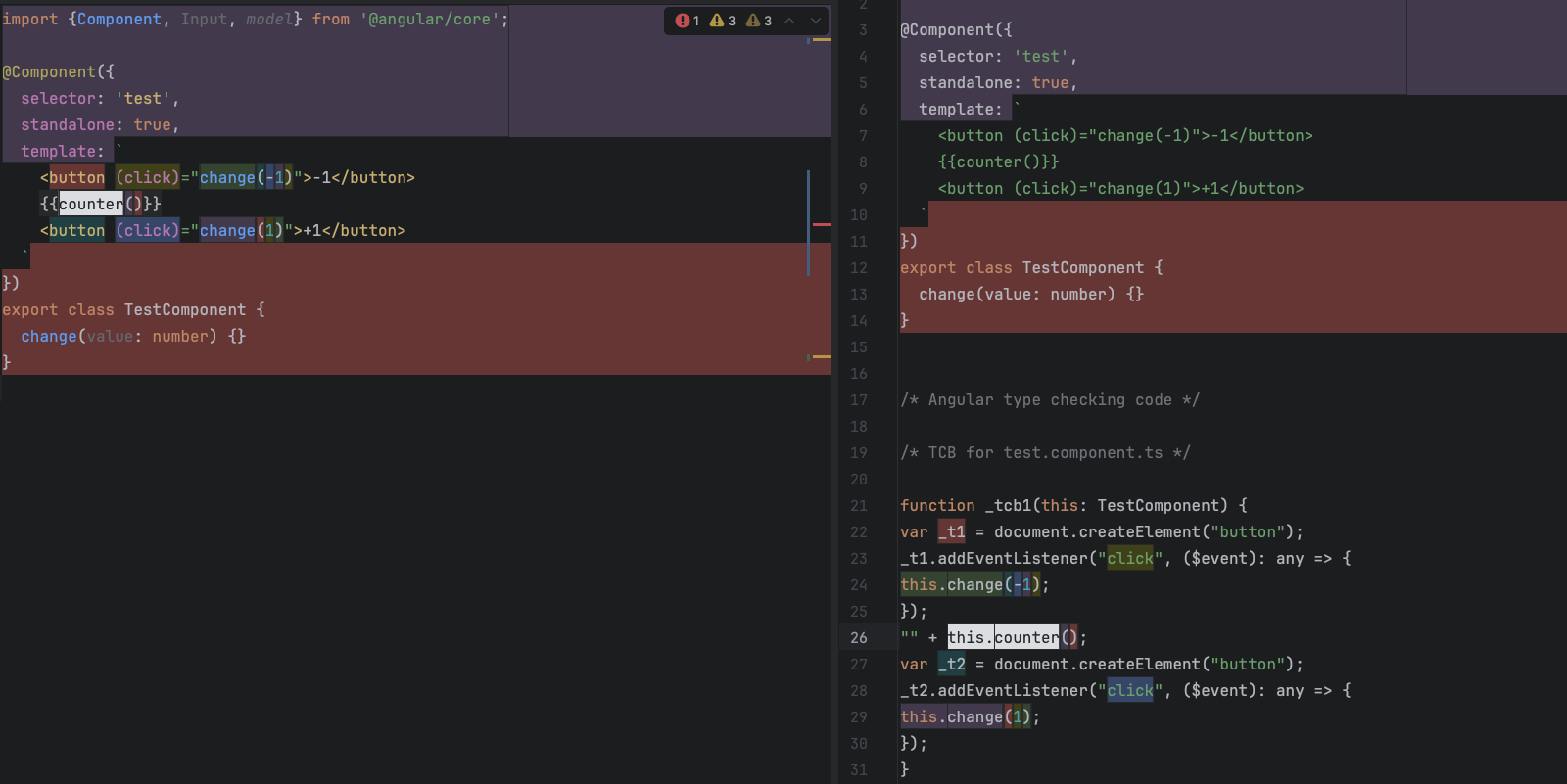
See how this.counter
is used in the template, which gets converted to the function _tcb1
on the right side, but isn’t properly declared in TestComponent
.
You might now be asking, why doesn’t WebStorm just use the Angular Language Server? Well, it turns out template type checking is just one piece of the IDE puzzle. WebStorm’s architecture already handles most of what the Angular Language Server provides:
- Navigation
- Code completion
- Quick-fixes
Furthermore, it offers parts of functionality that our users appreciate and that are not offered by the language server:
- Unit and E2E test integration for Jasmine, Jest, Vitest, Cypress, and Playwright.
- Debugging integration.
- Semantic highlighting of signals (fun fact: the Angular LSP does not provide syntax highlighting, which is part of the VS Code plugin).
- Special refactoring and quick-fix capabilities (e.g. extract Angular Component, create a signal ‘property’, etc.).
- IntelliSense without compiler support, like the host property mentioned above.
- Enhanced search capabilities (e.g. Find Usages for components).
The real-world implications
So what does this mean for you, the developer, just trying to get work done?
VS Code and Neovim users
- Standardized experience across editors
- Regular updates with Angular releases
- Built-in type correctness
WebStorm users
- Built-in integration of broader IDE tools (debugger, test runner, run configurations, etc.)
- Direct TCB integration for template type checking
- Additional navigation, refactoring features, and consistent development experiences across different languages (HTML, CSS, and TypeScript)
Looking forward
The Angular Language Server continues to evolve, making developers’ lives easier. Meanwhile, WebStorm’s specialized approach shows that there’s more than one way to achieve excellent Angular support.