An Introduction to Django Views
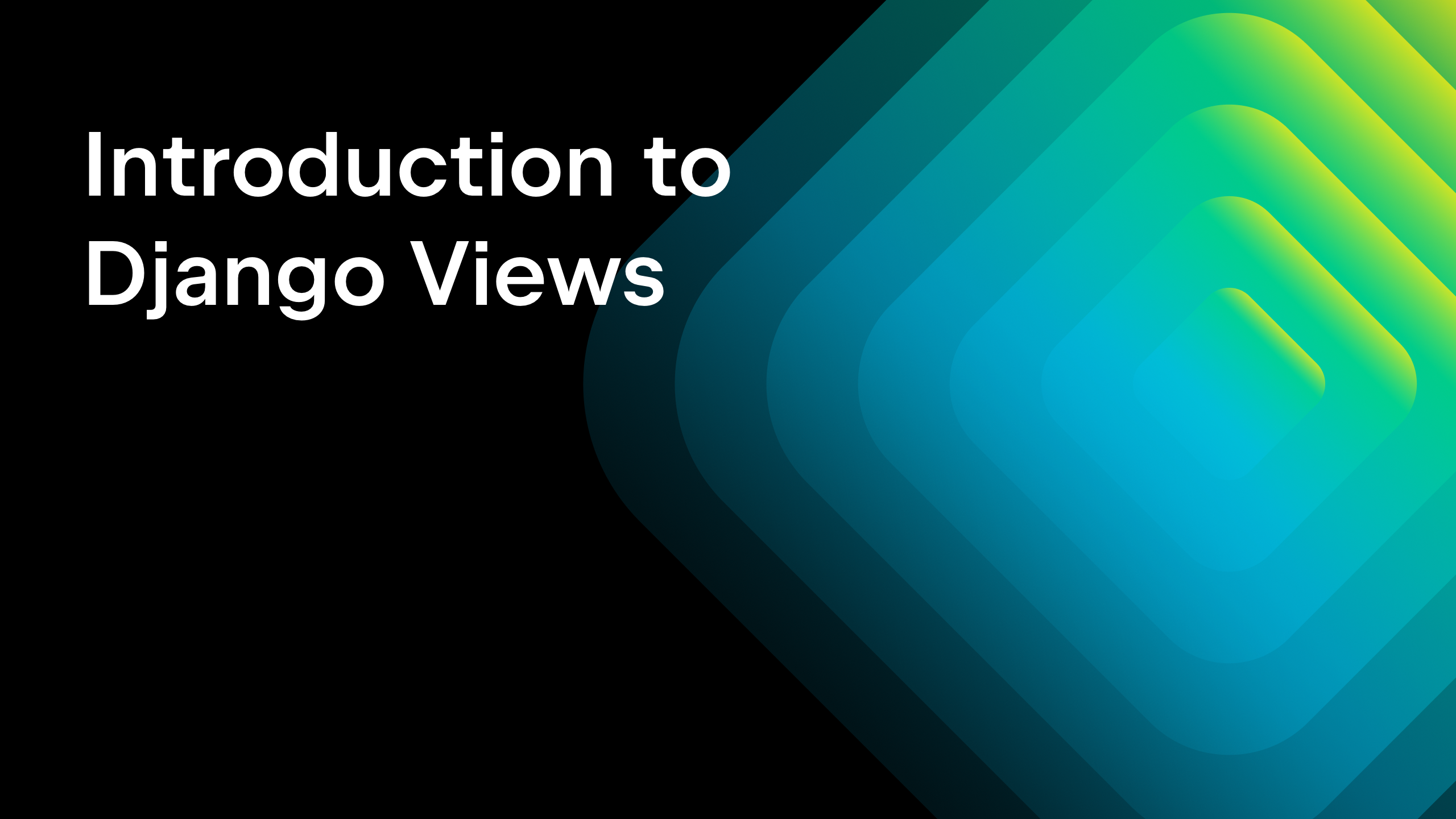
Views are central to Django’s architecture pattern, and having a solid grasp of how to work with them is essential for any developer working with the framework. If you’re new to developing web apps with Django or just need a refresher on views, we’ve got you covered.
Gaining a better understanding of views will help you make faster progress in your Django project. Whether you’re working on an API backend or web UI flows, knowing how to use views is crucial.
Read on to discover what Django views are, their different types, best practices for working with them, and examples of use cases.
What are Django views?
Views are a core component of Django’s MTV (model-template-view) architecture pattern. They essentially act as middlemen between models and templates, processing user requests and returning responses.
You may have come across views in the MVC (model-view-controller) pattern. However, these are slightly different from views in Django and don’t translate exactly. Django views are essentially controllers in MVC, while Django templates roughly align with views in MVC. This makes understanding the nuances of Django views vital, even if you’re familiar with views in an MVC context.
Views are part of the user interface in Django, and they handle the logic and data processing for web requests made to your Django-powered apps and sites. They render your templates into what the user sees when they view your webpage. Each function-based or class-based view takes a user’s request, fetches the data from its models, applies business logic or data processing, and then prepares and returns an HTTP response to a template.
This response can be anything a web browser can display and is typically an HTML webpage. However, Django views can also return images, XML documents, redirects, error pages, and more.
Rendering and passing data to templates
Django provides the render()
shortcut to make template rendering simple from within views. Using render()
helps avoid the boilerplate of loading the template and creating the response manually.
PyCharm offers smart code completion that automatically suggests the render()
function from django.shortcuts
when you start typing it in your views. It also recognizes template names and provides autocompletion for template paths, helping you avoid typos and errors.
The user provides the request, the template name, and a context dictionary, which gives data for the template. Once the necessary data is obtained, the view passes it to the template, where it can be rendered and presented to the user.
from django.shortcuts import render def my_view(request): # Some business logic to obtain data data_to_pass = {'variable1': 'value1', 'variable2': 'value2'} # Pass the data to the template return render(request, 'my_template.html', context=data_to_pass)
In this example, data_to_pass
is a dictionary containing the data you want to send to the template. The render
function is then used to render the template (my_template.html
) with the provided context data.
Now, in your template (my_template.html
), you can access and display the data.
<!DOCTYPE html> <html> <head> <title>My Template</title> </head> <body> <h1>{{ variable1 }}</h1> <p>{{ variable2 }}</p> </body> </html>
In the template, you use double curly braces ({{ }}
) to indicate template variables. These will be replaced with the values from the context data passed by the view.
PyCharm offers completion and syntax highlighting for Django template tags, variables, and loops. It also provides in-editor linting for common mistakes. This allows you to focus on building views and handling logic, rather than spending time manually filling in template elements or debugging common errors.
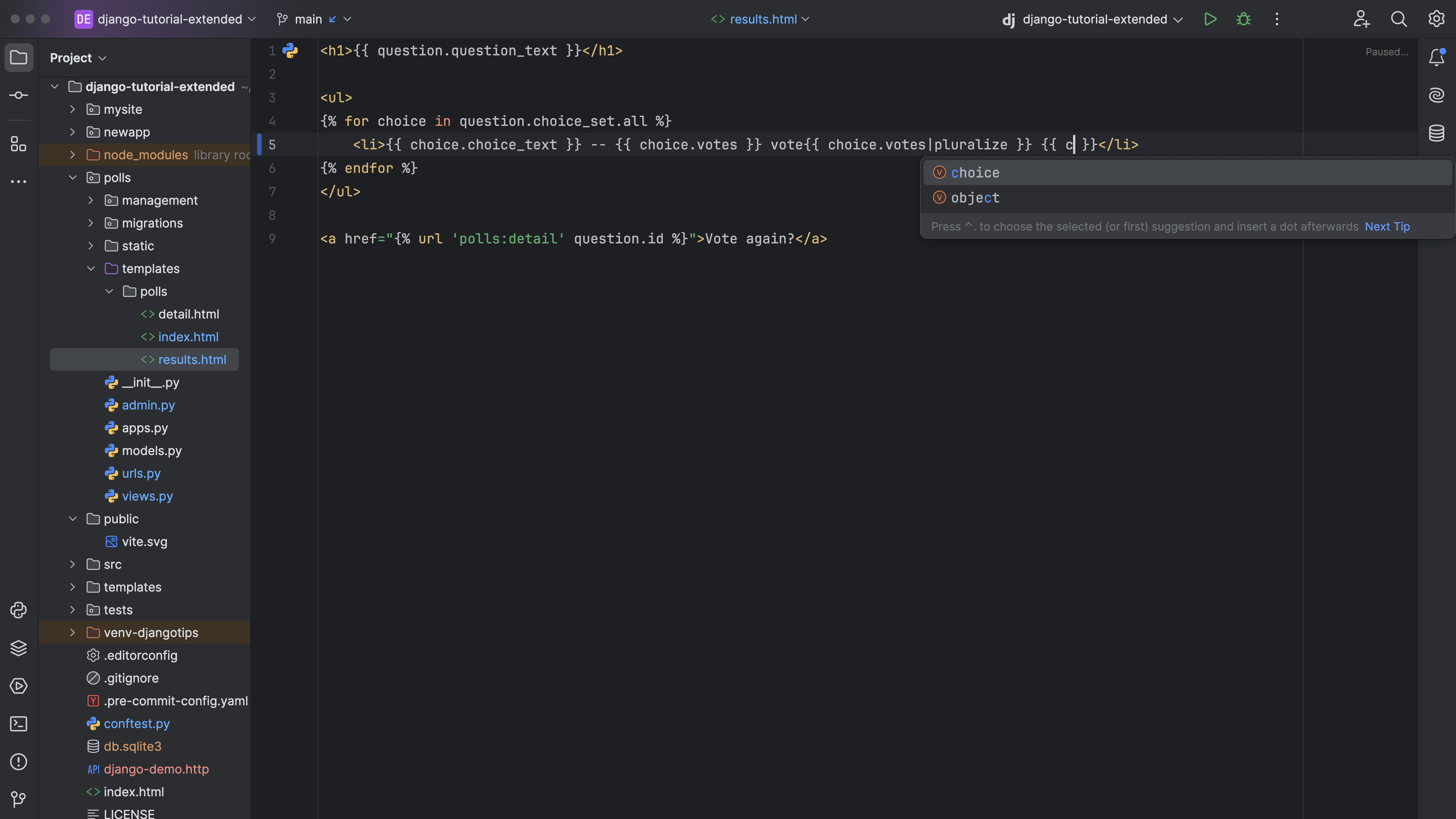
Function-based views
Django has two types of views: function-based views and class-based views.
Function-based views are built using simple Python functions and are generally divided into four basic categories: create, read, update, and delete (CRUD). This is the foundation of any framework in development. They take in an HTTP request and return an HTTP response.
from django.http import HttpResponse def my_view(request): # View logic goes here context = {"message": "Hello world"} return HttpResponse(render(request, "mytemplate.html", context))
This snippet handles the logic of the view, prepares a context dictionary for passing data to a template that is rendered, and returns the final template HTML in a response object.
Function-based views are simple and straightforward. The logic is contained in a single Python function instead of spread across methods in a class, making them most suited to use cases with minimal processing.
PyCharm allows you to automatically generate the def my_view(request)
structure using live templates. Live templates are pre-defined code snippets that can be expanded into boilerplate code. This feature saves you time and ensures a consistent structure for your view definitions.
You can invoke live templates simply by pressing ⌘J, typing Listview
, and pressing the tab key.
Moreover, PyCharm includes a Django Structure tool window, where you can see a list of all the views in your Django project, organized by app. This allows you to quickly locate views, navigate between them, and identify which file each view belongs to.
Class-based views
Django introduced class-based views so users wouldn’t need to write the same code repeatedly. They don’t replace function-based views but instead have certain applications and advantages, especially in cases where complex logic is required.
Class-based views in Django provide reusable parent classes that implement various patterns and functionality typically needed by web application views. You can take your views from these parent classes to reduce boilerplate code.
Class-based views offer generic parent classes like:
ListView
DetailView
CreateView
- And many more.
Below are two similar code snippets demonstrating a simple BookListView.
The first shows a basic implementation using the default class-based conventions, while the second illustrates how you can customize the view by specifying additional parameters.
Basic implementation:
from django.views.generic import ListView from .models import Book class BookListView(ListView): model = Book # The template_name is omitted because Django defaults to 'book_list.html' # based on the convention of <model_name>_list.html for ListView.
When BookListView
gets rendered, it automatically queries the Book records and passes them under the variable books when rendering book_list.html
. This means you can create a view to list objects quickly without needing to rewrite the underlying logic.
Customized implementation:
from django.views.generic import ListView from .models import Book class BookListView(ListView): model = Book # You can customize the view further by adding additional attributes or methods def get_queryset(self): # Example of customizing the queryset to filter books return Book.objects.filter(is_available=True)
In the second snippet, we’ve introduced a custom get_queryset()
method, allowing us to filter the records displayed in the view more precisely. This shows how class-based views can be extended beyond their default functionality to meet the needs of your application.
Class-based views also define methods that tie into key parts of the request and response lifecycle, such as:
get()
– logic forGET
requests.post()
– logic forPOST
requests.dispatch()
– determines which method to callget()
orpost()
.
These types of views provide structure while offering customization where needed, making them well-suited to elaborate use cases.
PyCharm offers live templates for class-based views like ListView
, DetailView
, and TemplateView
, allowing you to generate entire view classes in seconds, complete with boilerplate methods and docstrings.
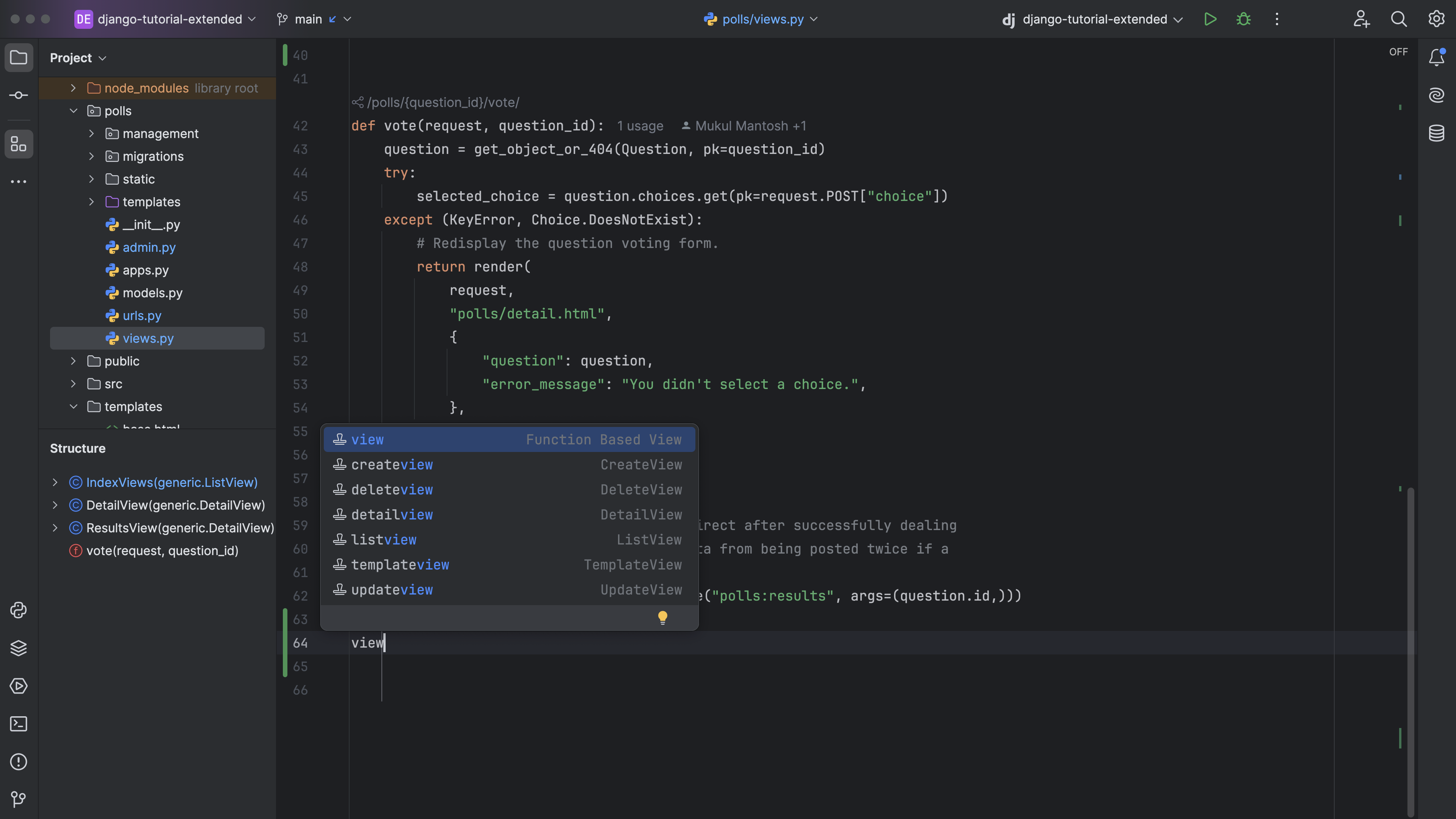
Creating custom class-based views
You can also create your own view classes by subclassing Django’s generic ones and customizing them for your needs.
Some use cases where you might want to make your own classes include:
- Adding business logic, such as complicated calculations.
- Mixing multiple generic parents to blend functionality.
- Managing sessions or state across multiple requests.
- Optimizing database access with custom queries.
- Reusing common rendering logic across different areas.
A custom class-based view could look like this:
from django.views.generic import View from django.shortcuts import render from . import models class ProductSalesView(View): def get(self, request): # Custom data processing sales = get_sales_data() return render(request, "sales.html", {"sales": sales}) def post(self, request): # Custom form handling form = SalesSearchForm(request.POST) if form.is_valid(): results = models.Sale.objects.filter(date__gte=form.cleaned_data['start_date']) context = {"results": results} return render(request, "search_results.html", context) # Invalid form handling errors = form.errors return render(request, "sales.html", {"errors": errors})
Here, custom get
and post
handlers enable you to extend the existing ones between requests.
When to use each view type
Function-based and class-based views can both be useful depending on the complexity and needs of the view logic.
The main differences are that class-based views:
- Promote reuse via subclassing and parents inheriting behavior.
- Are ideal for state management between requests.
- Provide more structure and enforced discipline.
You might use them working with:
- Dashboard pages with complex rendering logic.
- Public-facing pages that display dynamic data.
- Admin portals for content management.
- List or detail pages involving database models.
On the other hand, function-based views:
- Are simpler and take less code to create.
- Can be easier for Python developers to grasp.
- Are highly flexible and have fewer constraints.
Their use cases include:
- Prototyping ideas.
- Simple CRUD or database views.
- Landing or marketing pages.
- API endpoints for serving web requests.
In short, function-based views are flexible, straightforward, and are easier to reason about. However, for more complex cases, you’ll need to create more code that you can’t reuse.
Class-based views in Django enforce structure and are reusable, but they can be more challenging to understand and implement, as well as harder to debug.
Views and URLs
As we’ve established, in Django, views are the functions or classes that determine how a template is rendered. Each view links to a specific URL pattern, guiding incoming requests to the right place.
Understanding the relationship between views and URLs is important for managing your application’s flow effectively.
Every view corresponds with a URL pattern defined in your Django app’s urls.py
file. This URL mapping ensures that when a user navigates to a specific address in your application, Django knows exactly which view to invoke.
Let’s take a look at a simple URL configuration:
from django.urls import path from .views import BookListView urlpatterns = [ path('books/', BookListView.as_view(), name='book-list'), ]
In this setup, when a user visits /books/
, the BookListView
kicks in to render the list of books. By clearly mapping URLs to views, you make your codebase easier to read and more organized.
Simplify URL management with PyCharm
Managing and visualizing endpoints in Django can become challenging as your application grows. PyCharm addresses this with its Endpoints tool window, which provides a centralized view of all your app’s URL patterns, linked views, and HTTP methods. This feature allows you to see a list of every endpoint in your project, making it easier to track which views are tied to specific URLs.
Instead of searching through multiple urls.py
files, you can instantly locate and navigate to the corresponding views with just a click. This is especially useful for larger Django projects where URL configurations span multiple files or when working in teams where establishing context quickly is crucial.
Furthermore, the Endpoints tool window lets you visualize all endpoints in a table-like interface. Each row displays the URL path, the HTTP method (GET
, POST
, etc.), and the associated view function or class of a given endpoint.
This feature not only boosts productivity but also improves code navigation, allowing you to spot missing or duplicated URL patterns with ease. This level of visibility is invaluable for debugging routing issues or onboarding new developers to a project.
Check out this video for more information on the Endpoints tool window and how you can benefit from it.
Best practices for using Django views
Here are some guidelines that can help you create well-structured and maintainable views.
Keep views focused
Views should concentrate on handling requests, fetching data, passing data to templates, and controlling flow and redirects. Complicated business logic and complex processing should happen elsewhere, such as in model methods or dedicated service classes.
However, you should be mindful not to overload your models with too much logic, as this can lead to the “fat model” anti-pattern in Django. Django’s documentation on views provides more insights about structuring them properly.
Keep views and templates thin
It’s best to keep both views and templates slim. Views should handle request processing and data retrieval, while templates should focus on presentation with minimal logic.
Complex processing should be done in Python outside the templates to improve maintainability and testing. For more on this, check out the Django templates documentation.
Decouple database queries
Extracting database queries into separate model managers or repositories instead of placing them directly in views can help reduce duplication. Refer to the Django models documentation for guidance on managing database interactions effectively.
Use generic class-based views when possible
Django’s generic class-based views, like DetailView
and ListView
, provide reusability without requiring you to write much code. Opt for using them over reinventing the wheel to make better use of your time. The generic views documentation is an excellent resource for understanding these features.
Function-based views are OK for simple cases
For basic views like serving APIs, a function can be more effective than a class. Reserve complex class-based views for intricate UI flows. The writing views documentation page offers helpful examples.
Structure routes and URLs cleanly
Organize routes and view handlers by grouping them into apps by functionality. This makes it easier to find and navigate the source. Check out the Django URL dispatcher documentation for best practices in structuring your URL configurations.
Next steps
Now that you have a basic understanding of views in Django, you’ll want to dig deeper into the framework and other next steps.
- Brush up on your Django knowledge with our How to Learn Django blog post, which is ideal for beginners or those looking to refresh their expertise.
- Discover how to create and run your first Django project in PyCharm, with our tutorial on crafting a basic to-do application, or explore our complete list of Django project ideas for further inspiration.
- Explore the state of Django to see the latest trends in Django development for further inspiration.
- If you’re still deciding which Python framework to use, our Django vs. Flask and Django vs. FastAPI comparison guides can help.
Django support in PyCharm
PyCharm Professional is the best-in-class IDE for Django development. It allows you to code faster with Django-specific code assistance, project-wide navigation and refactoring, and full support for Django templates. You can connect to your database in a single click and work on TypeScript, JavaScript, and frontend frameworks. PyCharm also supports Flask and FastAPI out of the box.
Create better applications and streamline your code. Get started with PyCharm now for an effortless Django development experience.