Django vs. FastAPI: Which is the Best Python Web Framework?
Introduction
If you’re new to web development and have only recently heard of “Frameworks” as a method of speeding up your application development, then you’re in the right place. Today, we’ll explore the two most popular web frameworks in the Python ecosystem currently, namely Django and FastAPI.
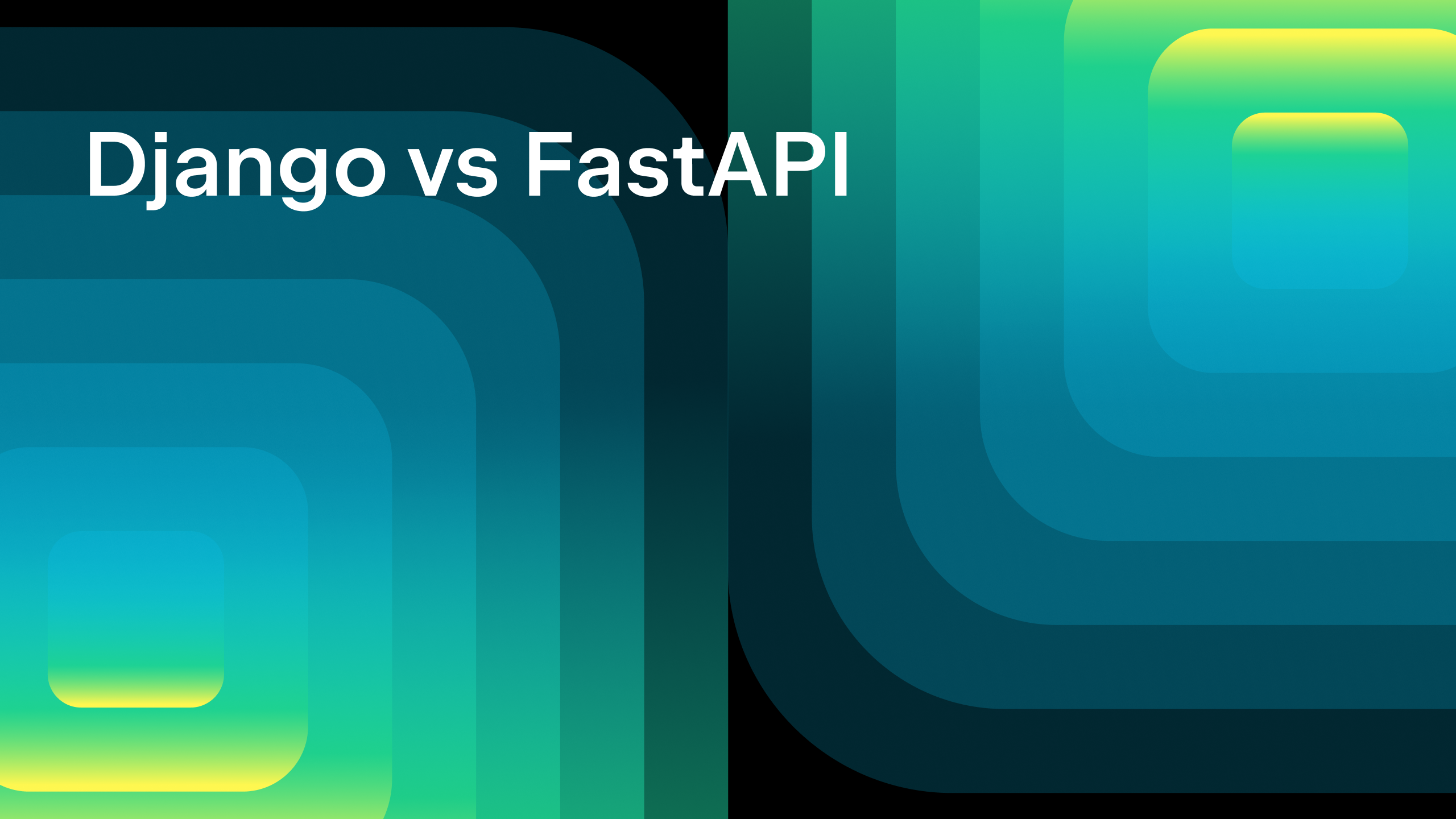
In this blog post, we’ll help you understand which framework is best for you in terms of business requirements, learning curve, complexity, speed, support, and more.
Django
Django is a high-level web framework for building web applications in Python. It follows the model-view-controller (MVC) architectural pattern, emphasizing reusability, pluggability, and rapid development. Django provides a range of built-in features, such as an object-relational mapping (ORM) system for database interactions, an admin panel for managing content, and a templating engine for designing user interfaces. It aims to simplify the development process by promoting a clean and pragmatic coding style, allowing developers to focus on building robust and scalable web applications.
For a more comprehensive understanding of Django, see this dedicated blog post.
Architecture
Django follows the MVT pattern, which stands for model, view, and template.
Model: A model is defined as the exclusive and authoritative repository of knowledge pertaining to your data. Models, in essence, are Python entities that offer functionalities for administering (creating, reading, updating, and deleting) and querying records within the database. Each attribute from the model represents a database field.
An example from the official Django documentation:
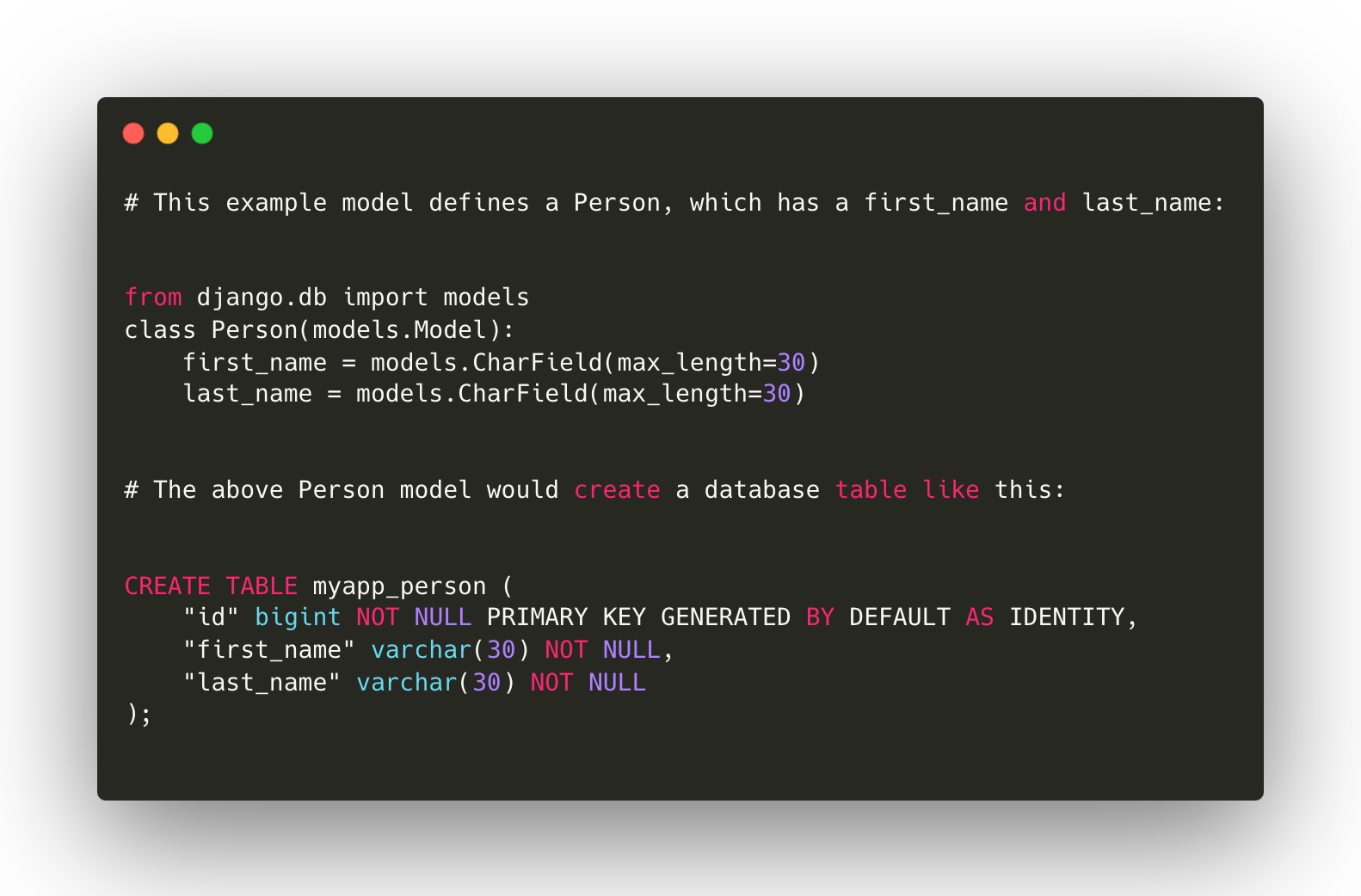
Reference: https://docs.djangoproject.com/en/4.2/topics/db/models/
View: A view serves as a request-handling function that takes an HTTP request, processes it by executing business logic, accesses data models directly or through ORMs, and ultimately sends the resulting HTTP response to be rendered in the user interface via templates.
Template: Django templates help you to design the structure and layout of your website by combining HTML with special tags and variables. These tags and variables are placeholders that Django fills in with actual content when a user views your web page. As such, a Django template makes creating dynamic and personalized web pages easier by allowing you to reuse and customize the overall design.
URLs: While processing requests from every single URL via a single function is possible, it is much more efficient to write a separate view function to handle each resource. A URL mapper is used to redirect HTTP requests to the appropriate view based on the request URL. The URL mapper can also match particular patterns of strings or digits that appear in a URL and pass these to a view function as data.
Benefits of Django
Velocity in action
Django was created to enable rapid application prototyping and facilitate a fast feedback loop.
Packed to perfection
Django provides out-of-the-box support for everything, offering a rich set of built-in features for handling development operations. The most powerful part of Django is the Admin interface, which automatically generates production-ready UIs from database models.
Ironclad confidence
Django offers built-in, fortified security measures, including safeguards against SQL injection, cross-site scripting (XSS), CSRF forgery, and a robust authentication system.
Trusted by titans
It may come as a surprise, but industry giants like Instagram, Spotify, Mozilla, National Geographic, and numerous others place their trust in Django.
Crafting a myriad of apps, from social to commerce and beyond
Django is an excellent choice for building applications like content management systems (CMS), e-commerce platforms, or social media applications. Many developers have also leveraged Django to create multi-tenant applications as well.
Django’s design philosophies
These fundamental philosophies have served as guiding principles throughout the framework’s development process.
Loose coupling
One of the core principles underlying Django’s architecture is maintaining a balance between minimal interdependence and strong internal unity. The different components within the framework should only have knowledge of each other when this is absolutely essential.
Less code
Django applications promote code simplicity by minimizing unnecessary code and eliminating boilerplate whenever possible. Django is designed to harness Python’s dynamic features, including introspection, to their fullest extent.
Quick development
Django enables swift web development by expediting laborious tasks. Django facilitates rapid development through its high-level abstractions and built-in features, streamlining common web development tasks.
“Don’t repeat yourself” (DRY)
Each unique concept or piece of data should exist in a single, exclusive location. Redundancy is undesirable, while normalization is favorable.
To a reasonable extent, the framework should extract as much information as it can from minimal input.
Explicit is better than implicit
In Python, there’s a basic idea mentioned in PEP 20. It advises that when using Django, a tool for making web apps, we should keep “magic” to a minimum. Magic in programming means making things work automatically without showing how they work. We should only use this kind of magic for simplifying our work. However, we need to ensure that this magic doesn’t make Django confusing for those beginners trying to learn the framwork.
Consistency
Consistency within the framework should be maintained across all levels, encompassing aspects ranging from the low level, such as adhering to a consistent Python coding style, to the high level, including the overall user experience when working with Django.
How to install Django
- First, we are going to create a virtual environment. For our example, we are going to create a Python virtual environment and name it
DjangoEnv
.
Once successfully created, we will activate the virtual environment.
- Run the following command to install the Django
pip install Django
- We are going to create a new Django project and name it
mysite
.
- Once the project is created successfully, move inside the project directory and run the following command:
python manage.py runserver
- Visit http://localhost:8000, where you will see the Django landing page. ?
Finding manually entering commands in the terminal exhausting and looking for a quicker solution? ? Check out our tutorials designed to get you up to speed in no time.
You may want to explore this blog post, which details a smooth method for creating Django projects.
Short on time? Skip the text and dive into quick, engaging videos to kick-start your journey!
FastAPI
FastAPI is one of the most popular Python frameworks available on the market, having amassed over 65,000 GitHub stars. It’s a high-performance framework, and its beauty lies in its exceptional use of type hints.
Type hints utilize Python annotations, a feature introduced in PEP 3107 and PEP 484. They serve the purpose of specifying types for variables, parameters, function arguments, return values, class attributes, and methods.
The creator of FastAPI, Sebastian Ramirez, also stands behind Typer, as well as various CLI-based apps and other open-source projects.
The FastAPI project has experienced significant growth in just a few years, much of which is attributable to its vibrant community. Moreover, it’s famously more straightforward to work with than Django.
FastAPI is backed by two major components:
- Pydantic
- Starlette
Pydantic is a Python library used for data validation and parsing. It is beneficial for data serialization and deserialization, making it easier to work with complex data types in Python. Pydantic allows you to define the structure of your data using Python data classes and then validate and parse incoming data according to that structure.
Pydantic has now unveiled its version 2, completely rewritten in Rust. As stated in the official Pydantic documentation, this update has led to a substantial performance boost, with a 4–50 times speed increase compared to the previous version.
Starlette is a lightweight ASGI (Asynchronous Server Gateway Interface) framework for building web applications and microservices in Python. It provides the foundation for building asynchronous web applications using Python’s asyncio library. Starlette is designed to be minimalistic and modular, allowing developers to pick and choose the components they need for their specific application.
According to the official website, it is production-ready and gives you the following:
- A lightweight, low-complexity HTTP web framework
- WebSocket support
- In-process background tasks
- Startup and shutdown events
- A TestClient built on HTTPX
- Support for CORS, GZip, StaticFiles, and streaming responses
- Session and cookie support
- 100% test coverage
- 100% type-annotated codebase
- A small number of hard dependencies
- Compatibility with asyncio and trio backends
Benefits of FastAPI
FastAPI is designed for building efficient APIs quickly with auto-generated API docs using Swagger or ReDoc.
Moreover, it has additional benefits.
- The major advantage of FastAPI is its async nature, backed by libraries like Starlette and Pydantic.
- FastAPI has garnered the interest of organizations in search of exceptionally efficient API development frameworks due to its emphasis on performance, contemporary design, and developer-friendly features.
- FastAPI provides comprehensive support for asynchronous programming, enabling the creation of highly responsive APIs capable of efficiently managing numerous simultaneous requests.
How to install FastAPI
First, create a new Python Virtual environment. Once created, activate the environment.
Now, go ahead and install FastAPI by running the following command:
pip install fastapi
Then, you need to install Uvicorn. Uvicorn is an ASGI web server implementation for Python.
This is what a bare minimum FastAPI code block looks like, with no need for any complex setup.
To run the application, type the following command:
uvicorn main:app –reload
Django vs. FastAPI: A Comparison
Django
- If you’d like to have all the necessary features already there in one place, without the need to set anything up manually, then you should definitely consider Django. DjangoAdmin, ORM, and built-in authentication are all available out of the box.
- Django can be very useful when working with large complex projects that have tons of complex functionalities, for example, building multi-tenant apps, internalization (i18n) support, caching, etc.
- When it comes to maturity, Django is over 18 years old and has a robust framework with extensive community support.
- There’s a steep learning curve compared to FastAPI, as Django has many features, which can take time to master and might be unnecessary depending on your use case.
FastAPI
- FastAPI’s features align well with the principles and requirements of microservices architecture, providing the necessary tools and capabilities to build scalable, efficient, and loosely coupled microservices.
- When you’re tasked with creating applications that need to support real-time features, such as WebSockets, FastAPIs become a valuable choice. FastAPIs offer efficient and quick ways to develop web applications, making them well-suited for handling real-time functionality and delivering responsive user experiences.
- You can boost your application development speeds by generating auto-docs using Swagger/Redoc using Python type hints and Pydantic.
- FastAPI is relatively easy to learn when compared to Django.
Popularity
Django is one of the most popular and widely used Python backend frameworks, having been in service for nearly two decades. There’s no doubt that it is capable of handling all kinds of complexity, including building complex applications, caching, multiple databases, and authenticating users, while also offering out-of-the-box support for DjangoAdmin.
As per the Python Developer Survey 2022, Flask, Django, and FastAPI maintain their positions as the leading three Python web frameworks. FastAPI, which was initially launched in late 2018, has experienced year-over-year growth of 4%.
Per the StackOverflow Developer Survey 2023, Flask and Django continue to be consistently popular choices among developers, while FastAPI is steadily increasing its market share, reaching 7.42% in 2023.
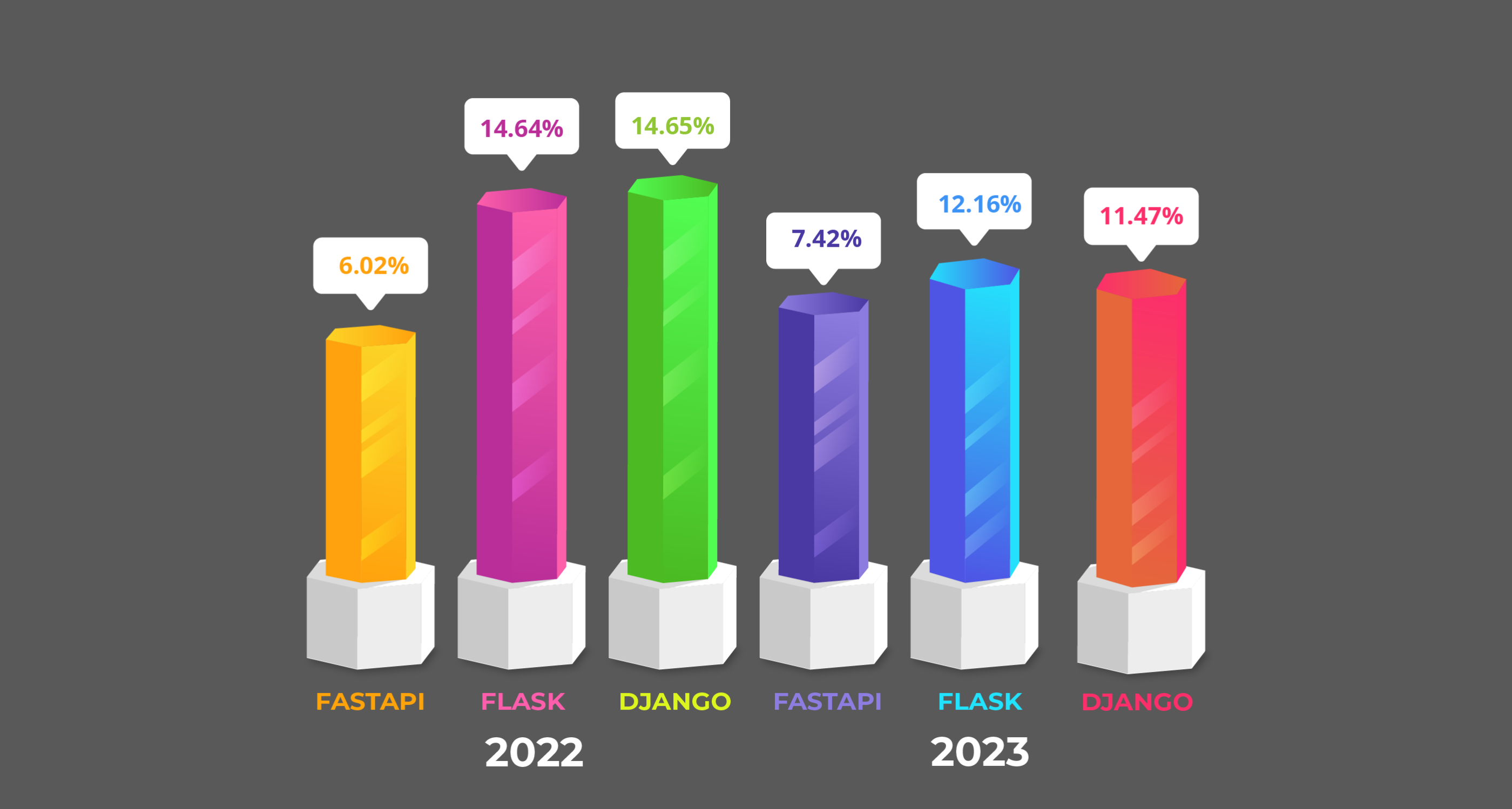
The Django community is really active and keeps on striving to improve the framework, providing updates, new features, and more. Not only that, the framework has received around 74,000 stars on GitHub, which clearly indicates how popular it is.
FastAPI, while relatively new when compared to Django, boasts significant potential for constructing high-performance APIs, handling asynchronous requests, and automatically generating documentation. This feature is particularly appreciated by developers, as it reduces the time they need to invest in documenting APIs, marking a substantial advancement.
Final thoughts
Django is a web framework equipped with an integrated admin interface, ORM, templates, authentication, and many more features, making it well-suited for extensive projects like content management systems, social media platforms, multi-tenant apps, and e-commerce websites. On the other hand, FastAPI is a contemporary API framework tailored to asynchronous programming, making it an excellent choice for data-rich APIs, machine-learning applications, and building microservices.
Django may not be the optimal framework for every situation. While it serves as an excellent framework for building extensive projects, it might be deemed excessive for smaller endeavors. Its robust, monolithic architecture could pose challenges for developers seeking more customizable and faster applications, especially for shorter scripts. Django excels in providing reliability, efficiency, architectural integrity, and security, which makes it particularly suitable for developing larger-scale applications.
FastAPI has a more limited library ecosystem compared to Django, and being relatively new at just five years old, it’s undergoing significant changes. Depending on your particular needs, there might be a necessity to develop extensions on your own.
At times, selecting the right framework can be quite challenging, yet it’s crucial to ensure that the chosen framework aligns with your precise business objectives before proceeding.
PyCharm Supports Django and FastAPI
No matter which framework is your primary one, you can have all the tools you need for web development in one IDE. PyCharm offers built-in support for Django, FastAPI, and Flask, and goes the extra mile by providing excellent support for frontend frameworks like React, Angular, and Vue.js.
Let’s explore the IDE playground and discover its features together.
Open PyCharm and click on New Project.
Click on Django and provide a project name.
You can effortlessly generate virtual environments or link up with existing ones. After completion, simply click on Create.
PyCharm will effortlessly configure everything for you. Just click on the play icon, and the server will initiate without the need for any manual commands.
Hooray! The server is already up and running.
The setup for FastAPI is quite similar to Django.
Open PyCharm, select FastAPI, and specify the project name.
PyCharm will then create a boilerplate. ?
Click on the play icon to start the server.
Effortlessly initiate/terminate/reload the FastAPI server at your convenience.
Tutorials
If you’re eager to delve deeper into Django and FastAPI, we’ve curated some tutorials for you.
Create a Django App in PyCharm
The tutorial covers creating a simple Django application that displays your local air temperature and allows you to explore weather conditions in random locations.
How To Use Materialized Views in Django
This post covers materialized views in Django. After reading it, you’ll know how to create and integrate materialized views into Django models, refresh them as required with the DROP statement, and utilize unique indexes to enhance performance.
Build Django Apps on Google Cloud With PyCharm and Cloud Code
This tutorial guides you through building and running applications in both local Kubernetes clusters and Google Kubernetes Engine using Cloud Code and PyCharm.
Developing FastAPI Application using K8s & AWS
This tutorial will exclusively concentrate on FastAPI while also exploring powerful platforms such as Kubernetes and Amazon Web Services.