Kotlin
A concise multiplatform language developed by JetBrains
Kotlin 1.1 Released With JavaScript Support, Coroutines, and More
Today we’re releasing Kotlin 1.1. It is a big step forward that enables the use of Kotlin in many new scenarios, and we hope you’ll enjoy it.
Our vision for Kotlin is to enable the use of a single expressive, performant, strongly typed language across all components of a modern application. Kotlin 1.1 makes two major steps toward this goal.
First, the JavaScript target is no longer experimental, and supports all Kotlin language features, a large part of the standard library, as well as JavaScript interoperability. This allows you to migrate the browser frontend of your applications to Kotlin, while continuing to use modern JavaScript development frameworks such as React.
Second, we’re introducing support for coroutines. As a lightweight alternative to threads, coroutines enable much more scalable application backends, supporting massive workloads on a single JVM instance. In addition to that, coroutines are a very expressive tool for implementing asynchronous behavior, which is important for building responsive user interfaces on all platforms.
Below we describe these two changes further. In other news: we’ve added type aliases, bound callable references, destructuring in lambdas and more. See the details in our What’s new page (check out the runnable examples!).
Coroutines
Coroutines in Kotlin make non-blocking asynchronous code as straightforward as plain synchronous code.
Asynchronous programming is taking over the world, and the only thing that is holding us back is that non-blocking code adds considerable complexity to our systems. Kotlin now offers a means to tame this complexity by making coroutines first-class citizens in the language through a single primitive: suspending functions. Such a function (or lambda) represents a computation that can be suspended (without blocking any threads) and resumed later.
Technically, coroutines are a light-weight way to cooperatively multi-task (very similar to fibers). In other words, they are just much better threads: almost free to start and keep around, extremely cheap to suspend (suspension is to coroutines what blocking is to threads), and very easy to compose and customize.
We designed coroutines for maximum flexibility: very little is fixed in the language, and very much can be done as a library. The kotlinx.coroutines project features libraries on top of Rx, CompletableFuture, NIO, JavaFx, and Swing. Similar libraries can be written for Android and JavaScript. Even many built-in constructs available in other languages can now be expressed as Kotlin libraries. This includes generators/yield from Python, channels/select from Go, and async/await from C#:
// runs the code in the background thread pool fun asyncOverlay() = async(CommonPool) { // start two async operations val original = asyncLoadImage("original") val overlay = asyncLoadImage("overlay") // and then apply overlay to both results applyOverlay(original.await(), overlay.await()) } // launches new coroutine in UI context launch(UI) { // wait for async overlay to complete val image = asyncOverlay().await() // and then show it in UI showImage(image) }
Read more in our docs.
An important note. With all the benefits that they bring, Kotlin coroutines are a fairly new design that needs extensive battle-testing before we can be sure it’s 100% right and complete. This is why we will release it under an “experimental” opt-in flag. We do not expect the language rules to change, but APIs may require some adjustments in Kotlin 1.2.
JavaScript support
As mentioned above, all language features in Kotlin 1.1, including coroutines, work on both JVM/Android and JavaScript. (Reflection for JavaScript is not available, but we’re looking into it.) This means that a web application can be written entirely in Kotlin, and we already have some experience doing that inside JetBrains. We will publish tutorials and other materials about this soon.
Kotlin for JavaScript has dynamic types to interoperate with “native” JavaScript code. To use well-known libraries through typed APIs, you can use the ts2kt converter together with headers from DefinitelyTyped.
We support both Node.js and the browser. The Kotlin Standard Library is available for use through npm
.
Read more in our docs.
Tooling
Kotlin 1.1 is not a major release for Kotlin tooling: we prefer shipping tooling features that do not affect the language itself as soon as they are ready, so you’ve seen many such improvements in Kotlin 1.0.x versions. To highlight a few:
- Kotlin plugins for all the major IDEs: IntelliJ IDEA, Android Studio, Eclipse, and NetBeans.
- Incremental compilation in both IntelliJ IDEA and Gradle.
- Compiler plugins for Spring, JPA and Mockito (making classses open and generating no-arg constructors).
- kapt for annotation processing.
- Lint support for Android projects.
- Numerous IDE intentions, inspections, quick-fixes, refactorings, and code completion improvements.
We’ll continue working to make our tooling even better and deliver the updates in 1.1.x versions.
First year of Kotlin: Adoption and the Community
In short, Kotlin is growing. We’ve seen over 160,000 people using it during the last year. OSS projects on Github grew by more than 300%, from 2.4M to 10M lines of Kotlin code. Our Slack community has grown from 1,400 to over 5,700 people (also quadrupled). Numerous meetups and user groups are organized by the community around the world. And we are seeing more and more Kotlin books and online courses published.
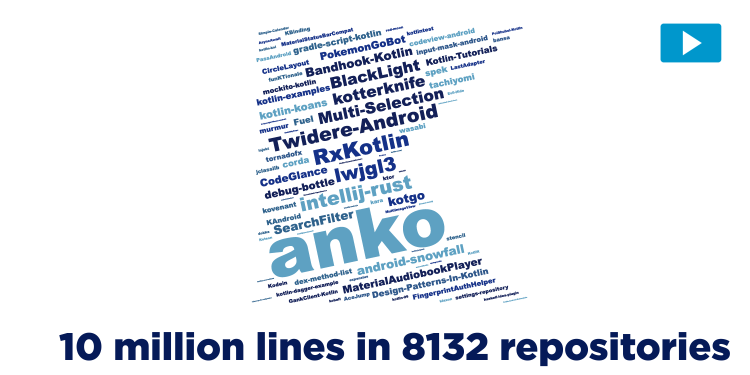
Kotlin is equally strong with server-side and Android developers (roughly a 50/50 divide). Spring Framework 5.0 has introduced Kotlin support, so did vert.x 3.4. Gradle and TeamCity are using Kotlin for build scripts. More projects using Kotlin can be found at kotlin.link.
Many well-known companies are using Kotlin: Pinterest, Coursera, Netflix, Uber, Square, Trello, Basecamp, amongst others. Corda, a distributed ledger developed by a consortium of well-known banks (such as Goldman Sachs, Wells Fargo, J.P. Morgan, Deutsche Bank, UBS, HSBC, BNP Paribas, and Société Générale), has over 90% Kotlin in its codebase.
We are grateful to all our users, contributors, and advocates in all parts of the world. Your support is very important to us!
Organize your own Kotlin 1.1 event
Kotlin 1.1 is a good reason to meet up with your local user group and friends. We have prepared some materials to help you organize such an event. On March 23 we’ll stream live sessions featuring the Kotlin team members, plus there’s an organizers pack that includes some swag and a Future Features Survey. Get more info and register your event here.
What’s next
To make Kotlin a truly full-stack language, we are going to provide tooling and language support for compiling the same code for multiple platforms. This will facilitate sharing modules between client and server. We will keep working on improving the JavaScript tooling and library support. Among other things, incremental compilation for the JavaScript platform is in the works already. Stay tuned for 1.1.x updates.
Java 9 is coming soon, and we will provide support for its new features before it ships.
We expect a lot of feedback on coroutines in the upcoming months, and improving this area of the language (in terms of both performance and functionality) is among our priorities.
Apart from this, our next release will be mostly focused on maintenance, performance improvements, infrastructure and bug-fixing.
P.S. Running on multiple platforms is a strategic direction for Kotlin. With 1.1 we can run on servers, desktops, and Android devices and browsers, but in the future we are going to compile Kotlin to native code and run on many more platforms (including, for example, iOS and embedded devices). A great team here at JetBrains is working on this project, and we are expecting to show something interesting fairly soon. This does not target any particular release yet, though.
Installation instructions
As always, you can try Kotlin online at try.kotlinlang.org.
In Maven/Gradle: Use 1.1.0
as the version number for the compiler and the standard library. See the docs here.
In IntelliJ IDEA: 2017.1 has Kotlin 1.1 bundled; in earlier versions, please install or update the Kotlin plugin to version 1.1.
In Android Studio: please install or update the plugin through Plugin Manager.
In Eclipse: install the plugin using Marketplace.
The command-line compiler can be downloaded from the Github release page.
Compatibility. In Kotlin 1.1 the language and the standard library are backwards compatible (bugs notwithstanding): if something compiled and ran in 1.0, it will keep working in 1.1. To aid big teams that update gradually, we provide a compiler switch that disables new features. This document covers the possible pitfalls.
Have a nice Kotlin!
P.S. See the discussions on Reddit and Hacker News