PhpStorm 2022.2 Is Now Available: Mockery Support, Rector Support, Improved Generics, and More!
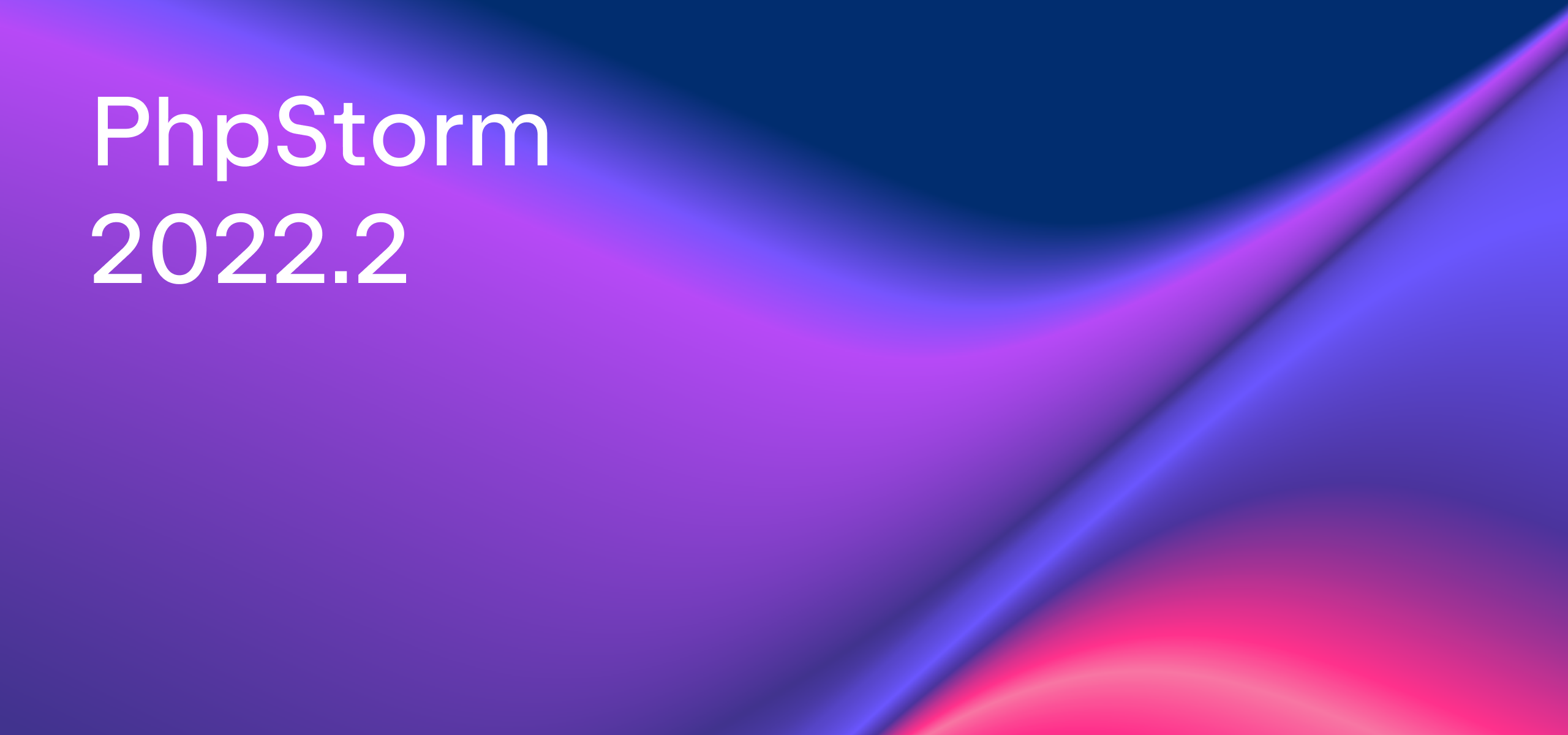
PhpStorm 2022.2 is a major update that brings support for Mockery and Rector, enhanced support for generics and enums, improvements to our debugger and HTTP client, and more.
You can download PhpStorm 2022.2 here and read through this post to learn about all the new features and improvements inside. Let’s dive in!
Rector support
Rector helps you with automatic PHP upgrades and code refactorings in bulk. It’s an amazing tool, and we’re happy to provide built-in support in PhpStorm for it.
In order to use PhpStorm’s Rector support, you first need to install Rector and configure it.
Next, you should create a new run configuration specifically for Rector. PhpStorm can do this automatically for you – simply right-click the folder you want Rector to fix and then select Run | Rector:
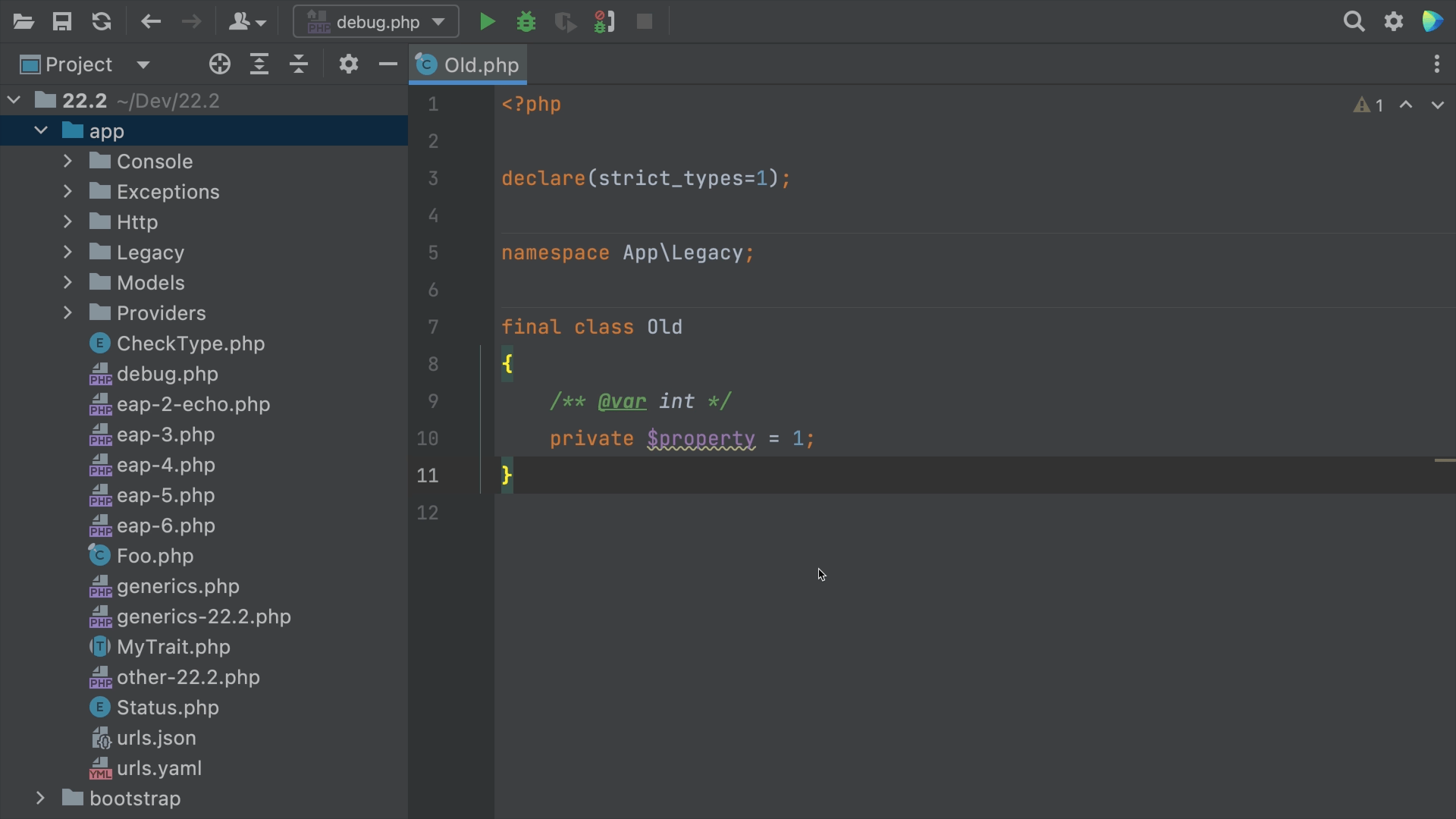
Note that you can also run Rector straight from the rector.php config file with a handy gutter icon:
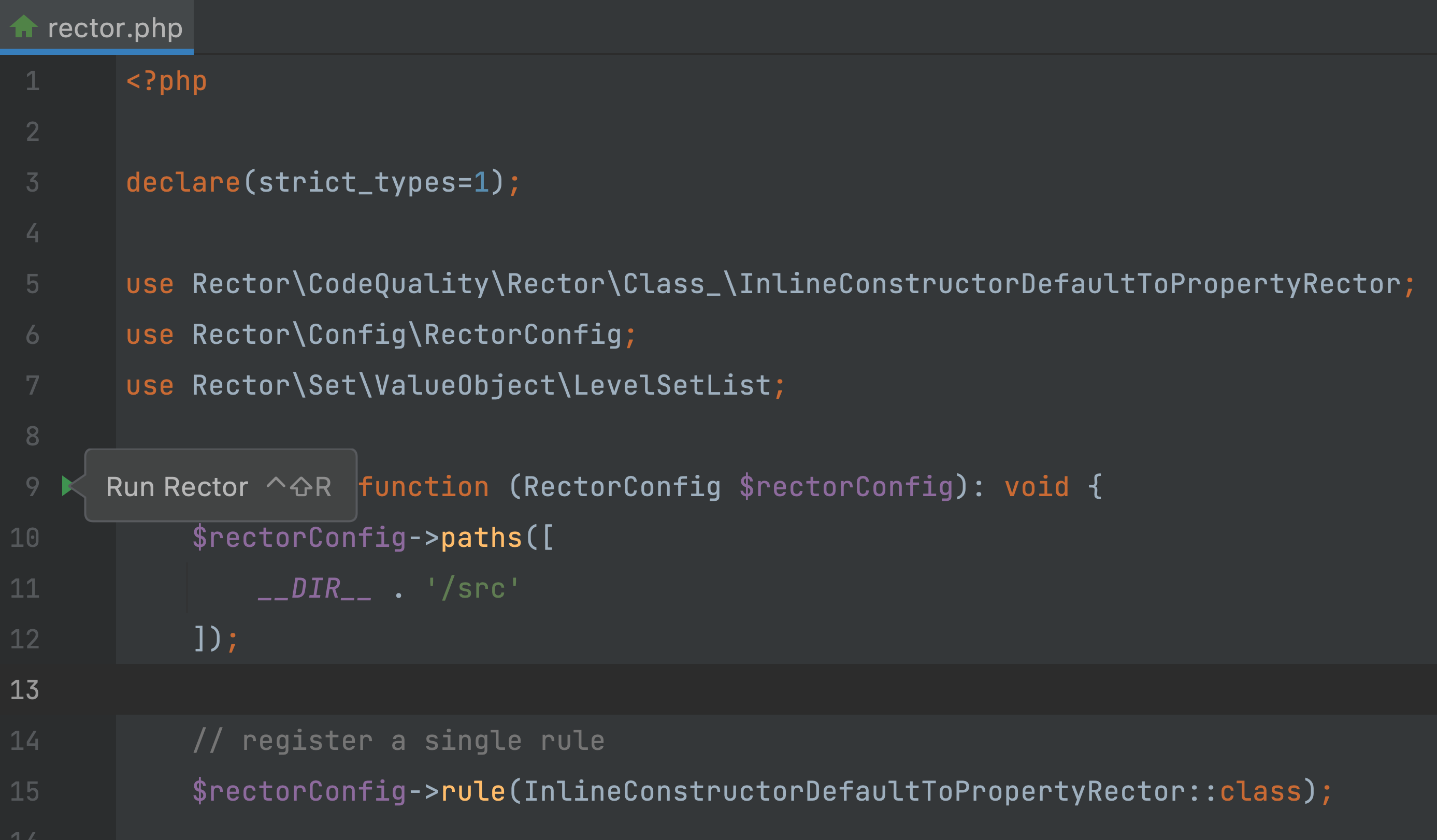
When Rector is done scanning, PhpStorm will give you a list of files that it wants to change. You can then review changes in PhpStorm, select individual files and folders, and finally apply all of the selected changes.
Since Rector is a run configuration, you have the option to configure it, as well. Either go to Run | Edit Configurations or choose Edit Configurations from the Actions menu:
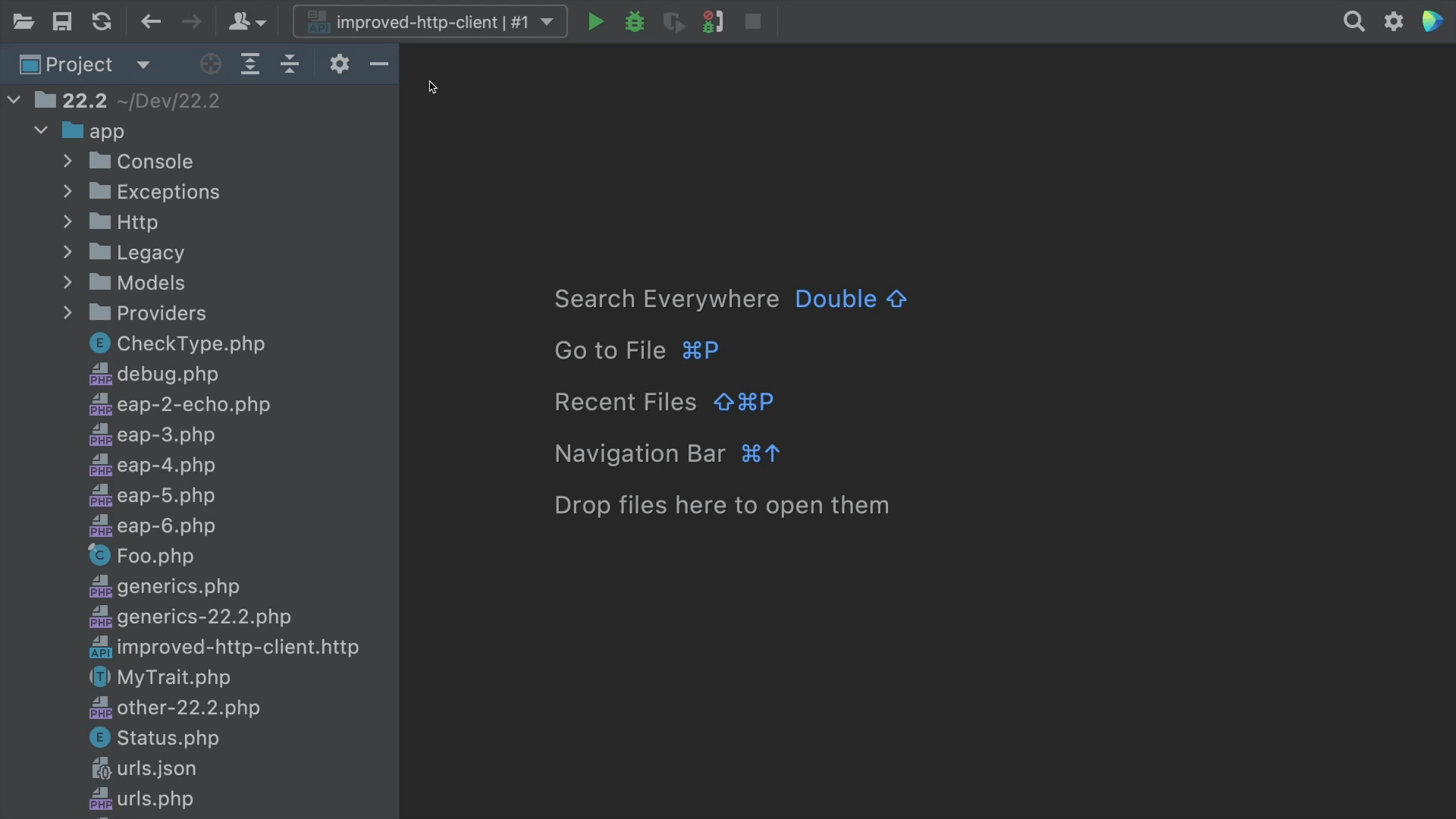
Mockery support
We’ve been working on implementing support for Mockery, one of the most popular mocking frameworks for unit testing in PHP. We’ve added support for autocompletion and code insights in many places, as well as some useful inspections.
Autocompletion for mocks
Proper autocompletion is now available for mocked objects. Both the mocked object’s methods and properties are available in the autocompletion menu, along with the functionality that Mockery provides through its mock objects.
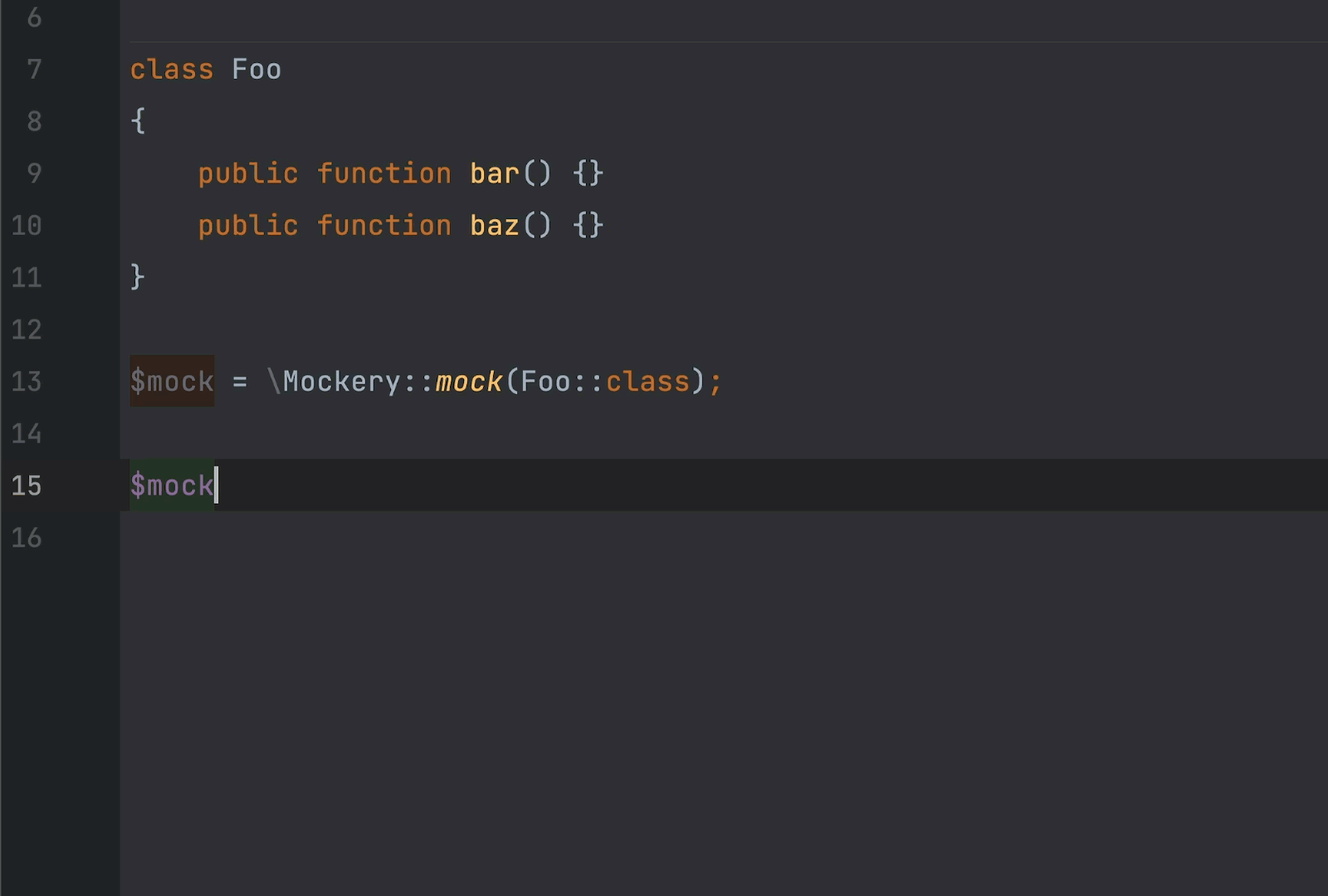
Support for class names as strings
It’s also possible to pass class names by string in the mock()
function. PhpStorm will suggest the right class name for you, autocomplete it, and offer the same insights into the mock object that you would see if you used the real class name.
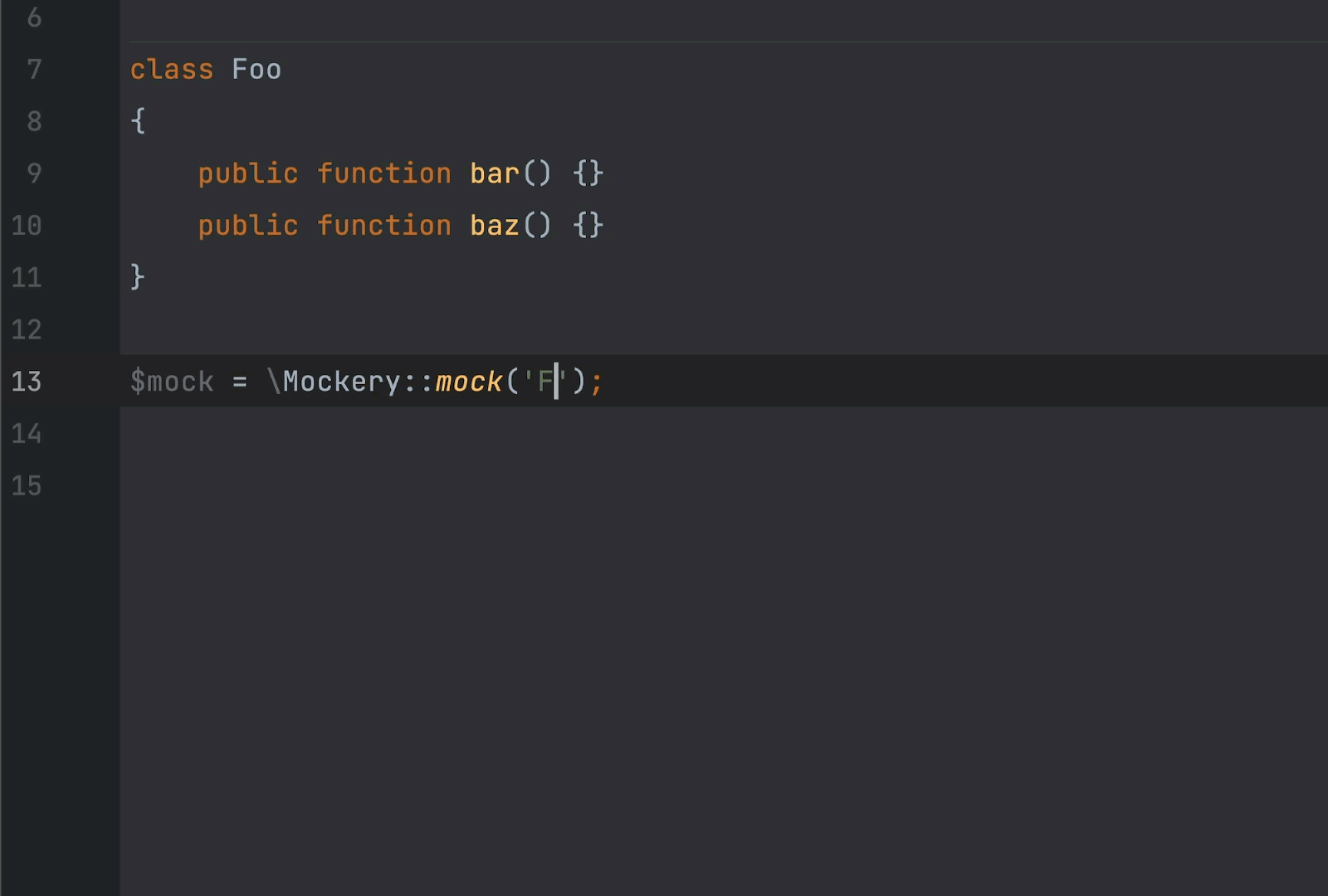
Support for mocking protected methods
PhpStorm will detect when you’re trying to use a protected method and will offer to add shouldAllowMockingProtectedMethods()
for you.
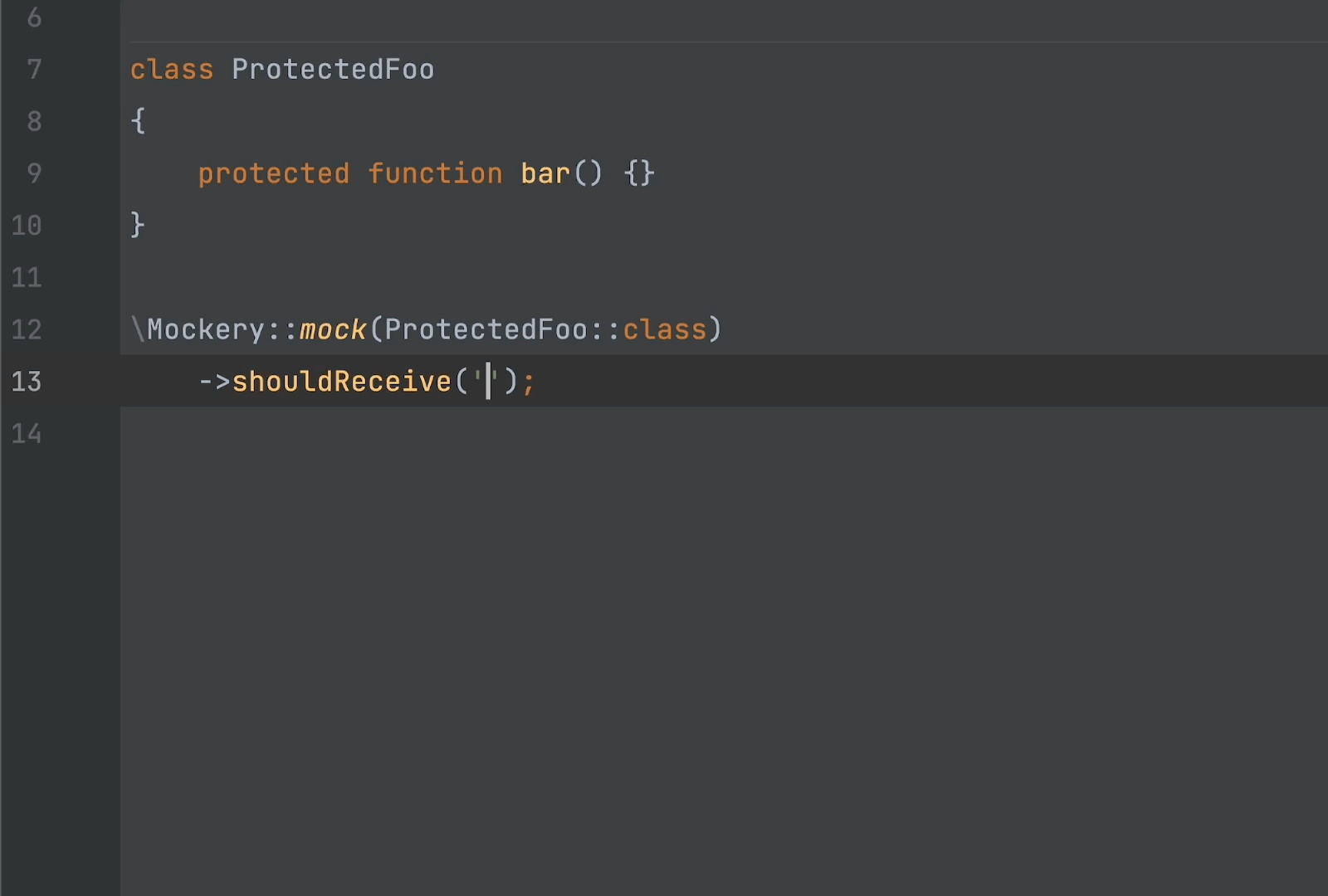
Mocking interfaces
PhpStorm will properly detect mocked interfaces for a given class and will be able to make suggestions based on the interface’s definition.
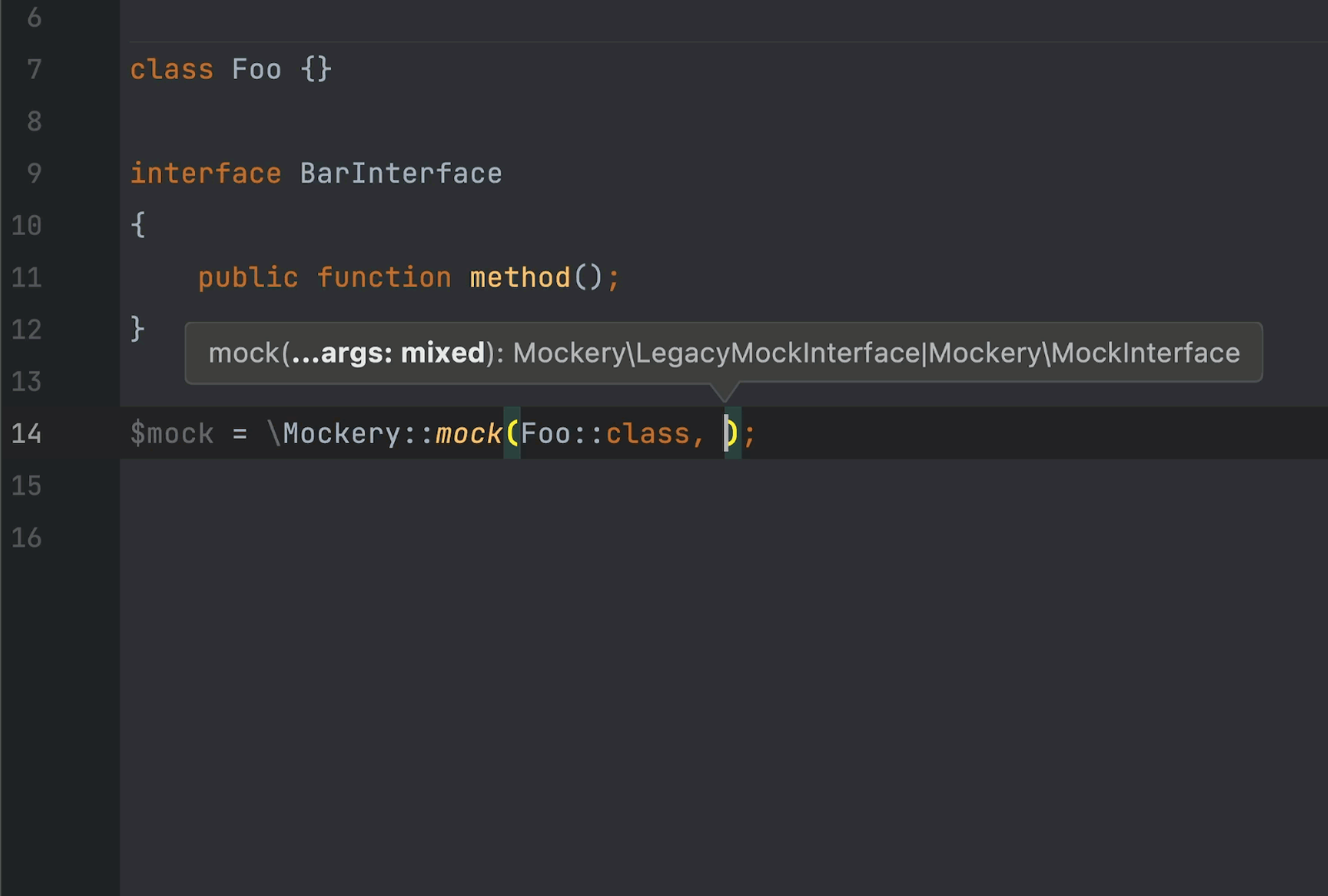
Partial mock support
Insights into mock objects will work even for partial mocks.
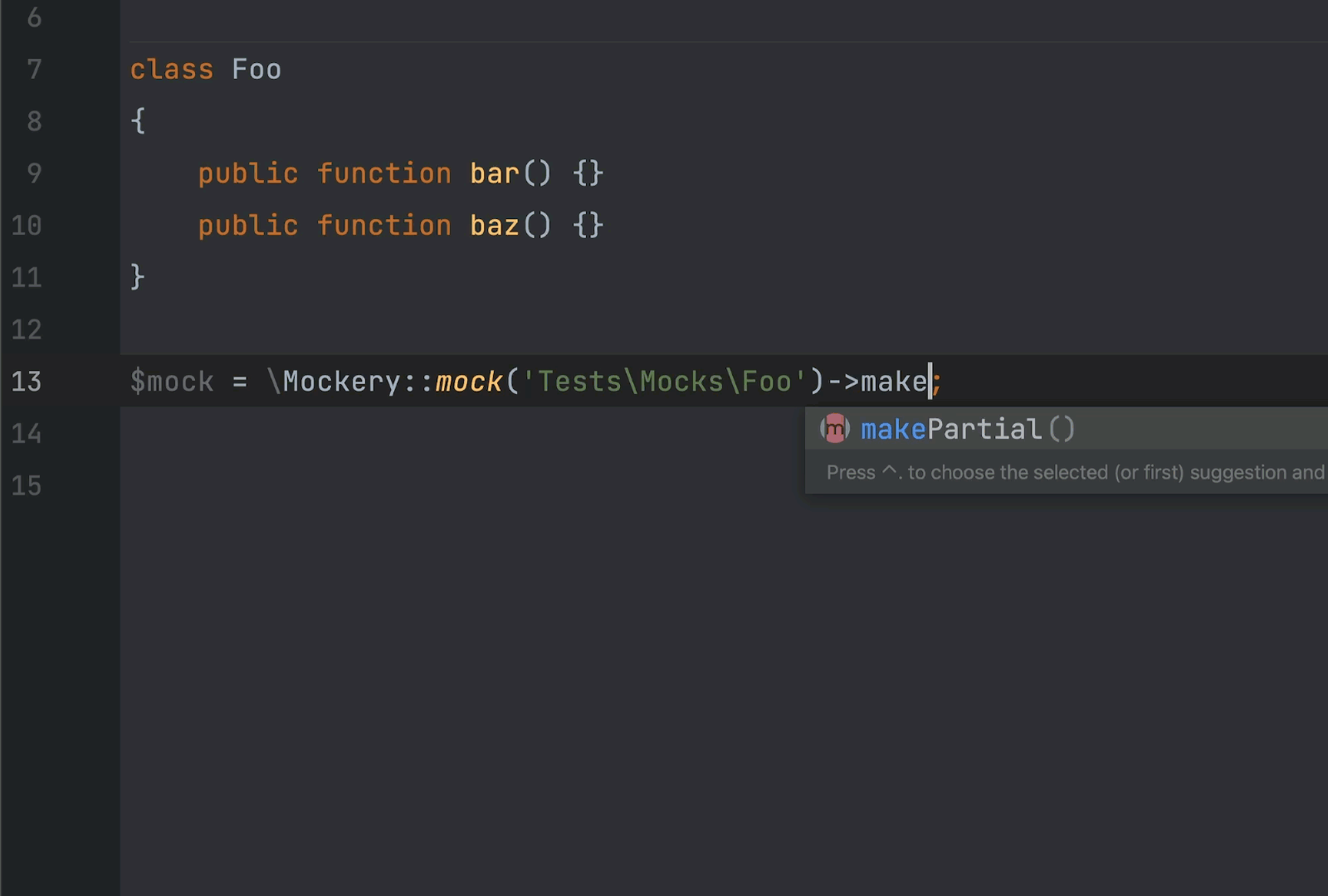
Highlight failed test assertions
Whenever one of your test assertions fail, PhpStorm will highlight the exact line where it failed and allow you to rerun the test from the failed line, thanks to a new context menu suggestion. Just press Alt+Enter
on the failed line to rerun that specific test.
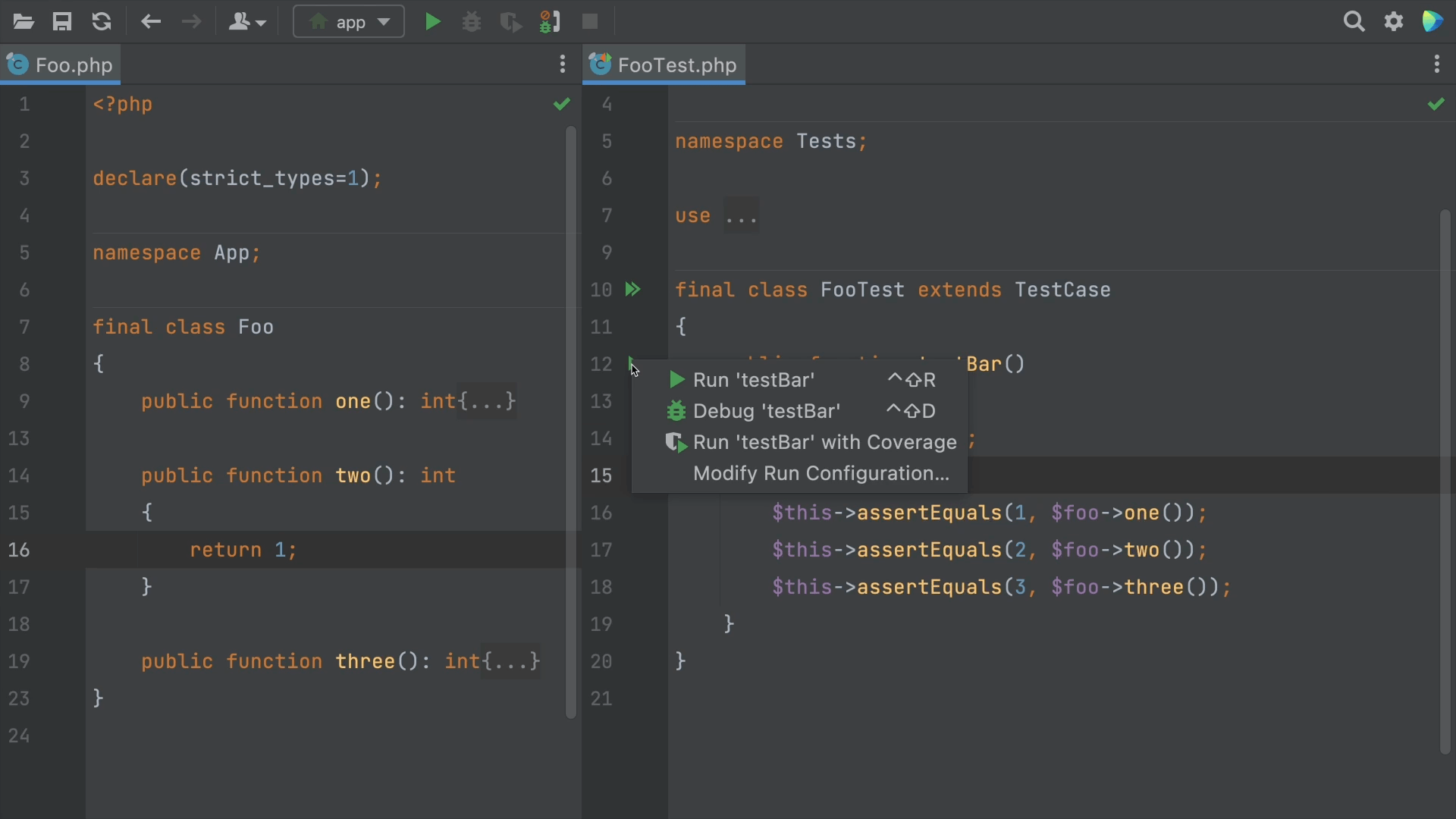
New inspections
Every major PhpStorm release brings a bunch of new inspections. Let’s take a look.
Combining multiple isset calls into one
Since PHP allows you to pass multiple arguments into one isset()
call, it makes sense for PhpStorm to tell you about possible refactorings to clean up your code.
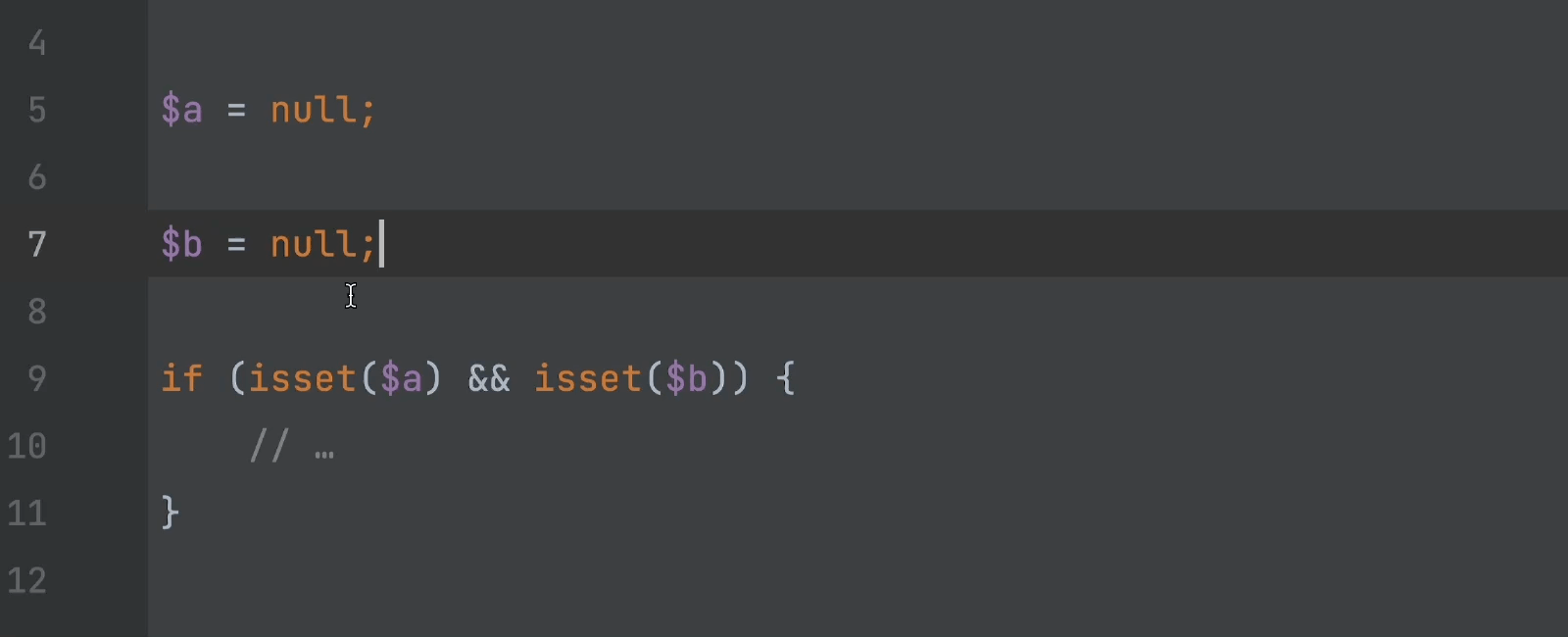
Use in_array() and array_key_exists() when possible
PhpStorm will be able to detect for loops that can be optimized to in_array()
or array_key_exists()
calls.
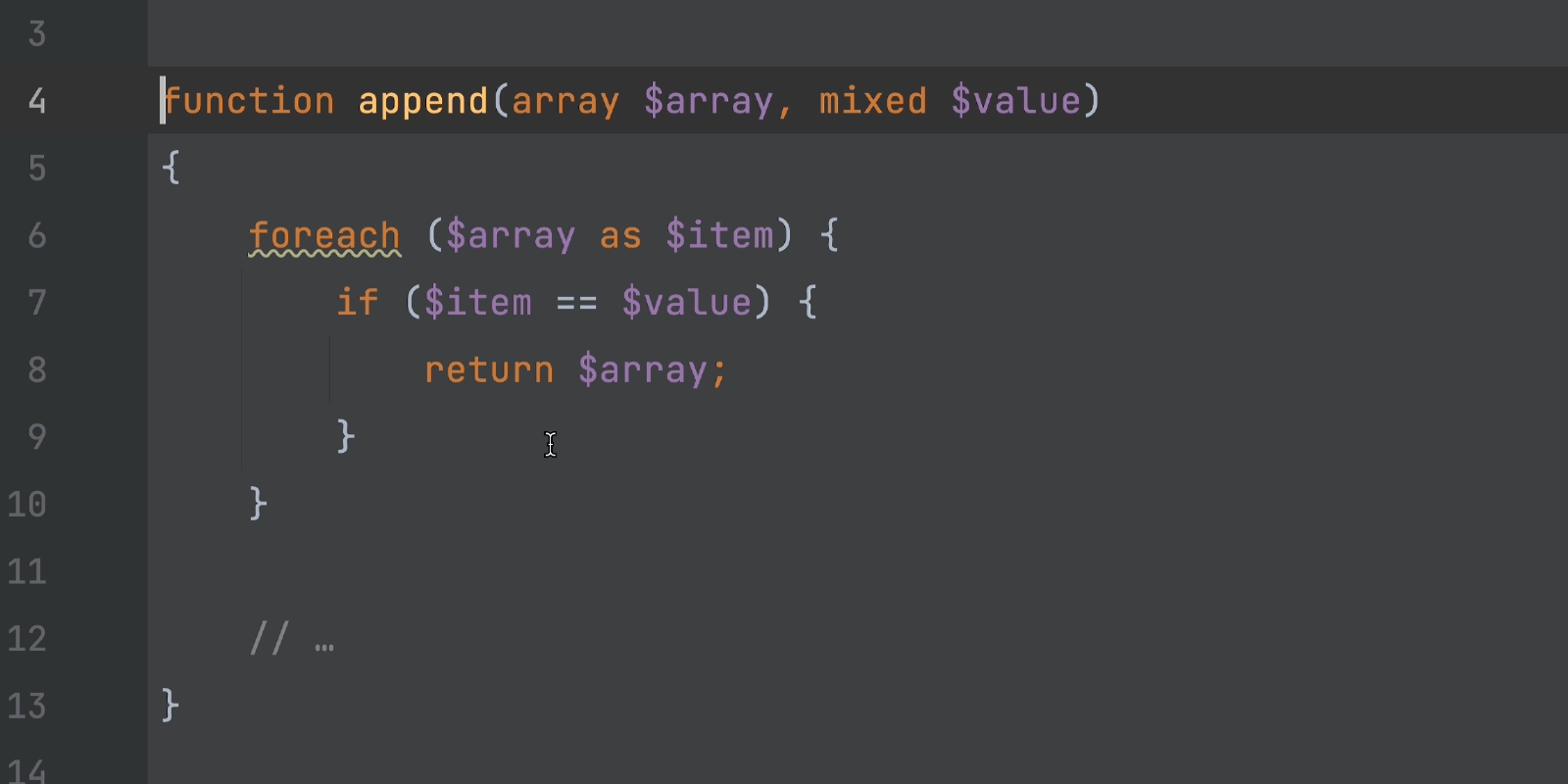
Add variable names to @var and @param docblocks when ambiguous
Sometimes PhpStorm can’t determine which variable a @var
or @param
docblock refers to, so we’ve added an inspection to make it easier for you to point to the right variable.

Convert array|Traversable to iterable
In PHP, the array|Traversable
union is equal to the built-in iterable
type. PhpStorm will now suggest replacing the former with the latter, resulting in cleaner code.
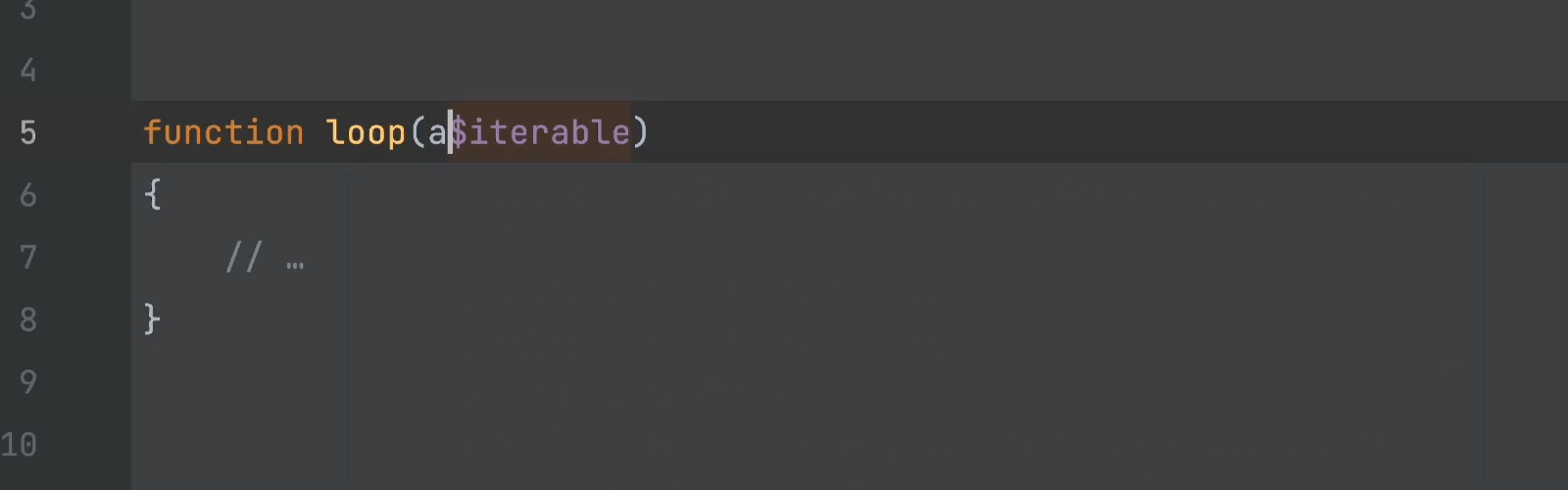
Convert <?php echo …; ?> to <?= and vice versa
PhpStorm now allows you to switch between <?php echo …; ?>
and <?=
within whole files with a single press of a button.
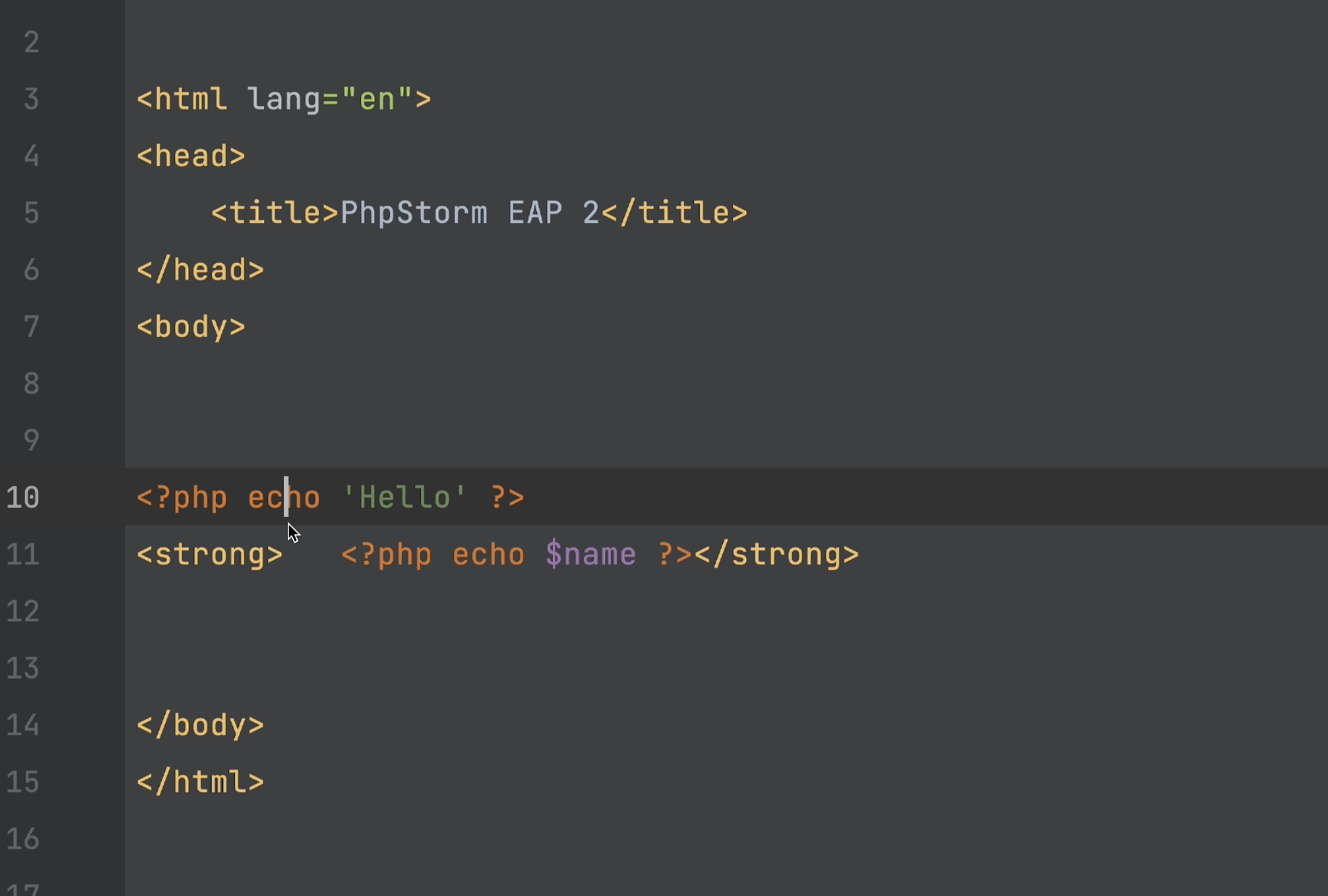
Replace simple preg_split() calls with explode()
PhpStorm will now detect simple preg_split()
calls and suggest using explode()
instead.
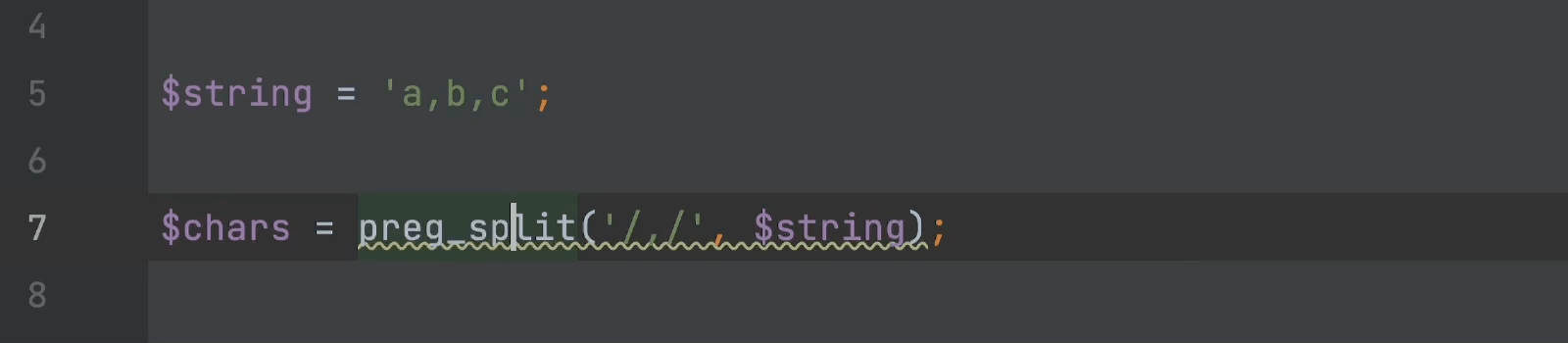
Enhanced configuration for highlighting inspections
We’ve improved the way you configure your preferences for highlighting inspections. When you want to change how an inspection appears in the editor, you can configure it using the new Highlighting in editor drop-down menu, which conveniently shows all available highlighting styles.
Faster access to code completion settings
You can now jump to your code completion settings right from the code completion popup in the editor whenever you need to change how autocompletion works.
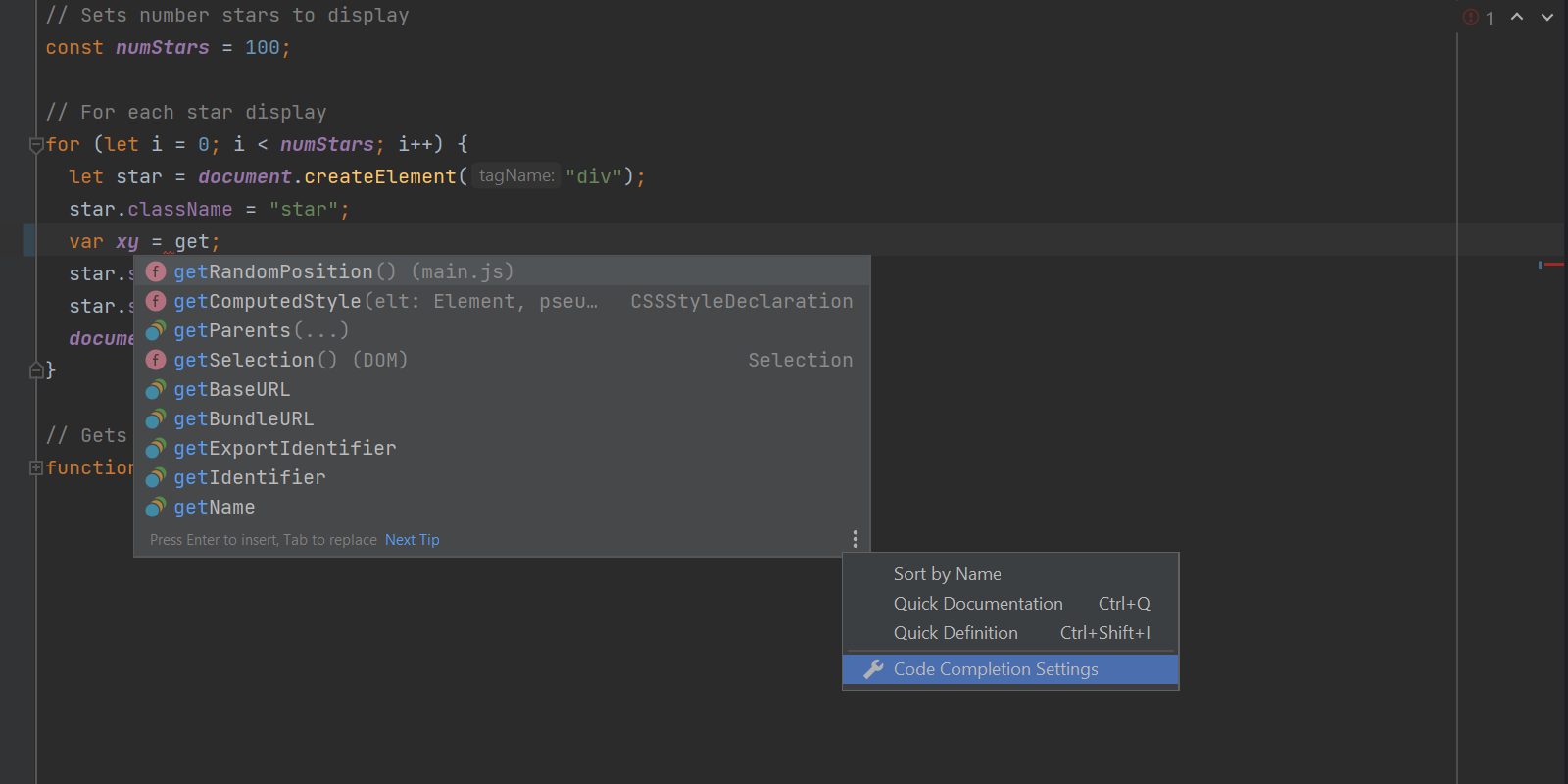
Improvements to our debugger
Besides new inspections, we’ve also made some quality-of-life improvements to our debugger:
Put $this at the top if available during debugging
When debugging in the context of a class, $this
will now always be on top:
Show concrete type and children of an array in the debug variables pane
The debugger’s variables pane now shows the contents of an array:
Display __toString() representation on an object
When an object implements the __toString()
method, that textual representation will also be displayed in the variables pane:
Add enum type to “New PHP Class” dialog
You can quickly create enums using the Create Class dialog. Note that the “Enum” option will only be available in projects that have their PHP level set to 8.1 or higher.
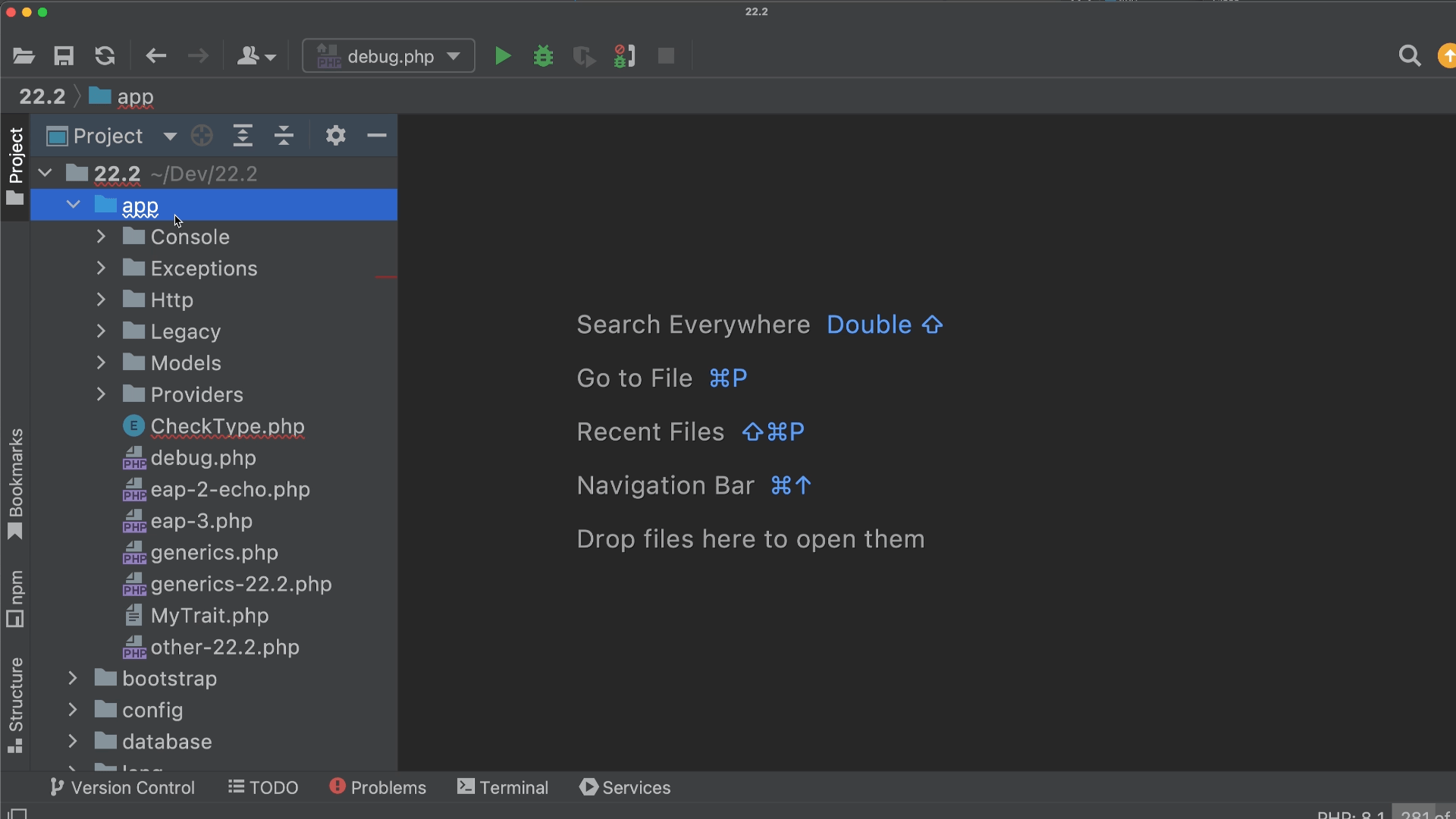
If you want to make changes to the default generated template, you can go to Settings / Preferences | Editor | File and Code Templates | Files | PHP Enum and change the template there.
Generics
When we announced basic support for generics in PhpStorm back in 2021, we knew there was plenty of work to be done in the future. Once again we’ve made lots of updates, so let’s take a look at what’s new with our generics support.
Int<min, max>
This EAP comes with many more improvements to generics. For example, we now support the int<min, max>
type, meaning you can define the minimum and maximum values of an integer for finer control over what can go into a variable.
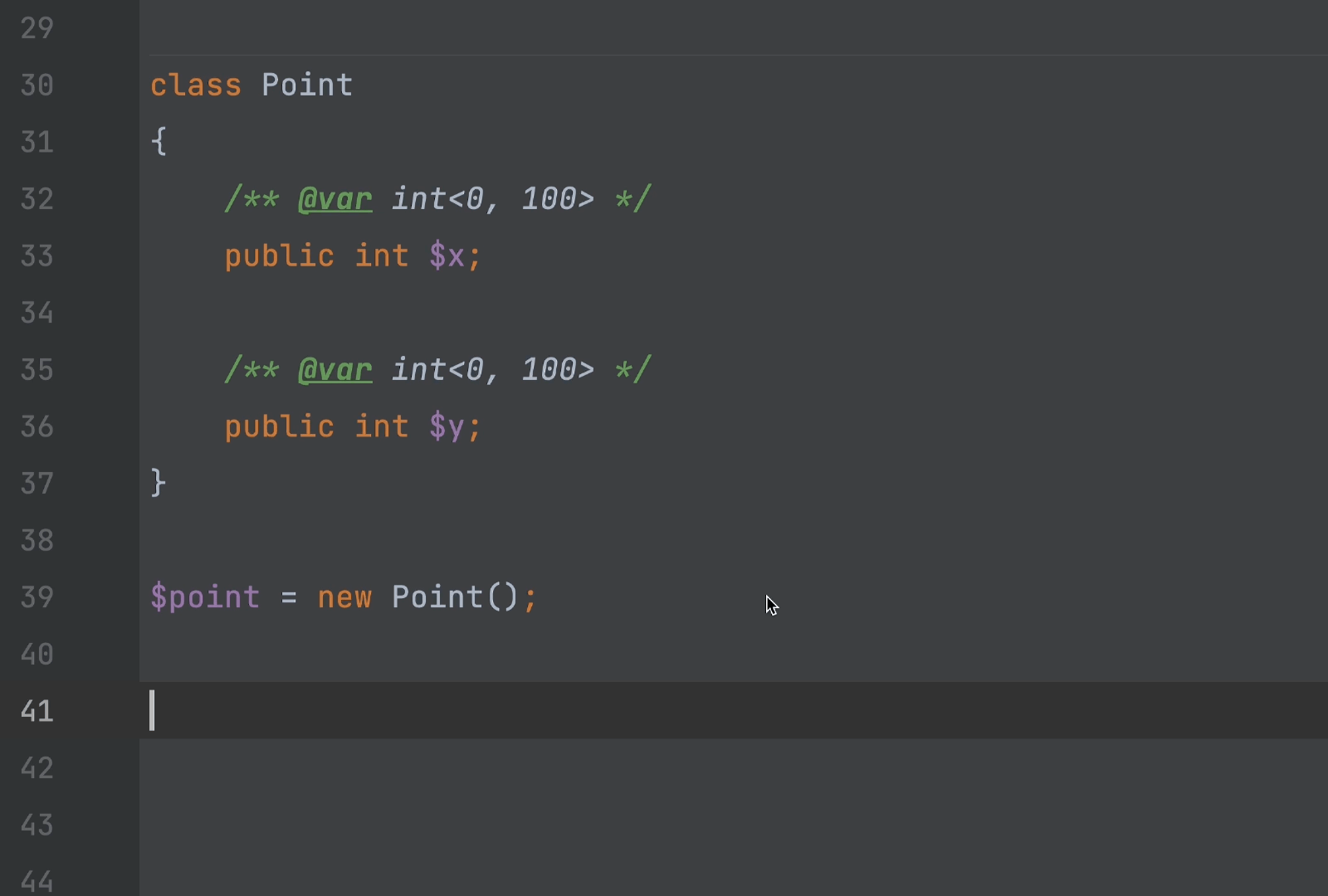
Generic support for iterables
PhpStorm will now also be able to infer types when looping over iterables:
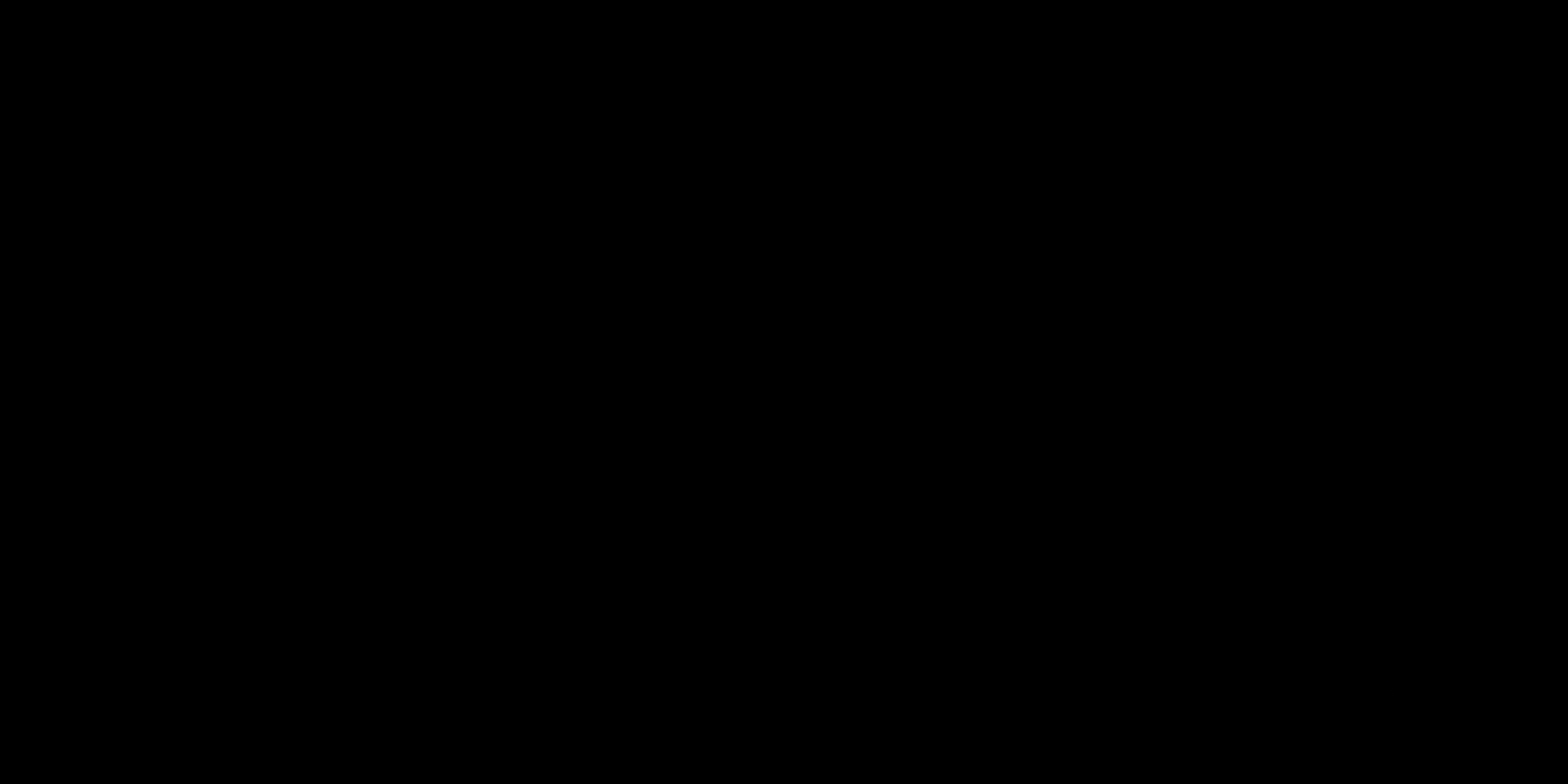
Infer types from closures
PhpStorm is now able to infer generic types from closure return values. This feature is used a lot in Laravel collections where you pass a closure to a collection function like map()
or first()
:
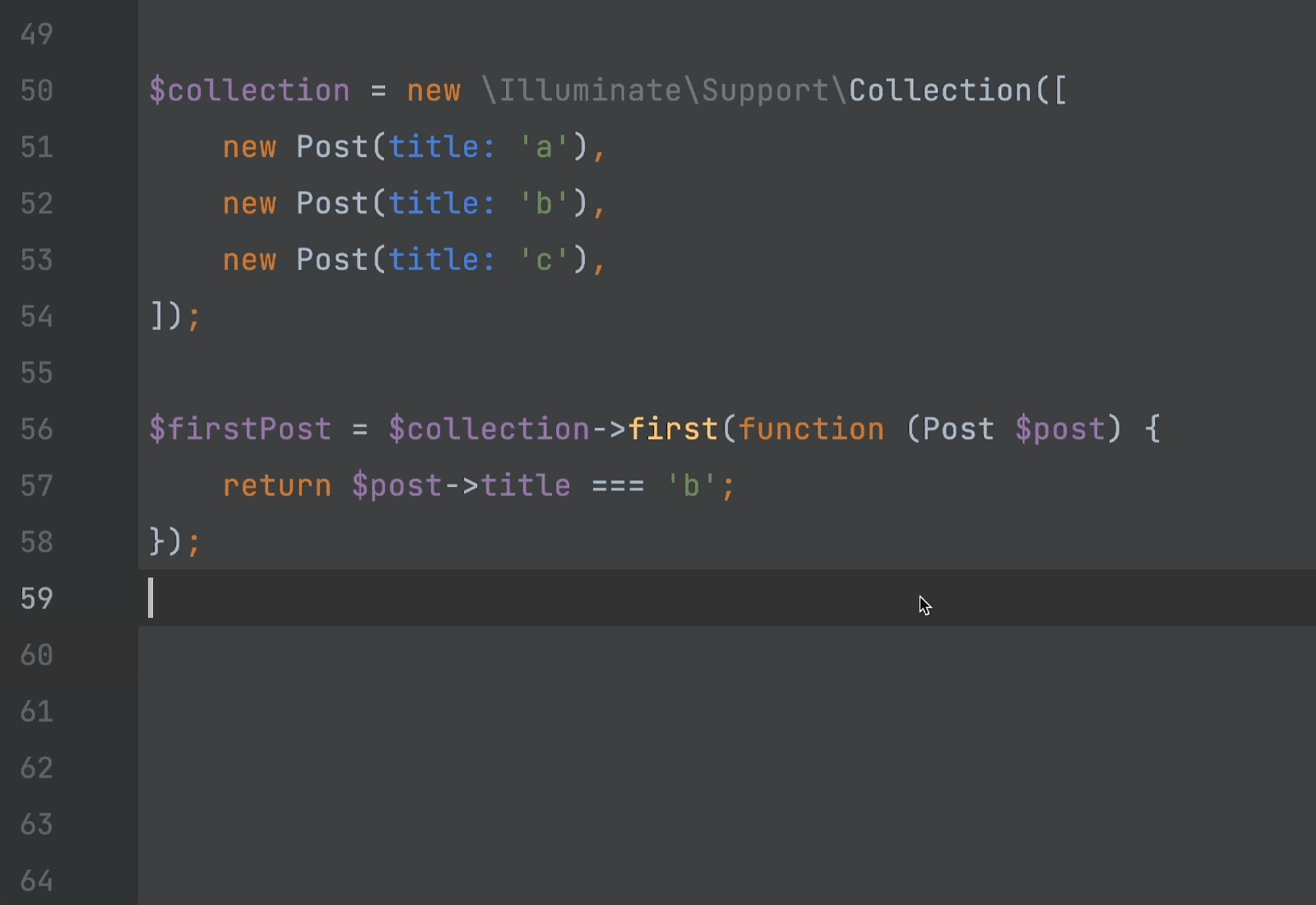
Generics in traits
You can now use generics in combination with traits by using the @use
annotation:
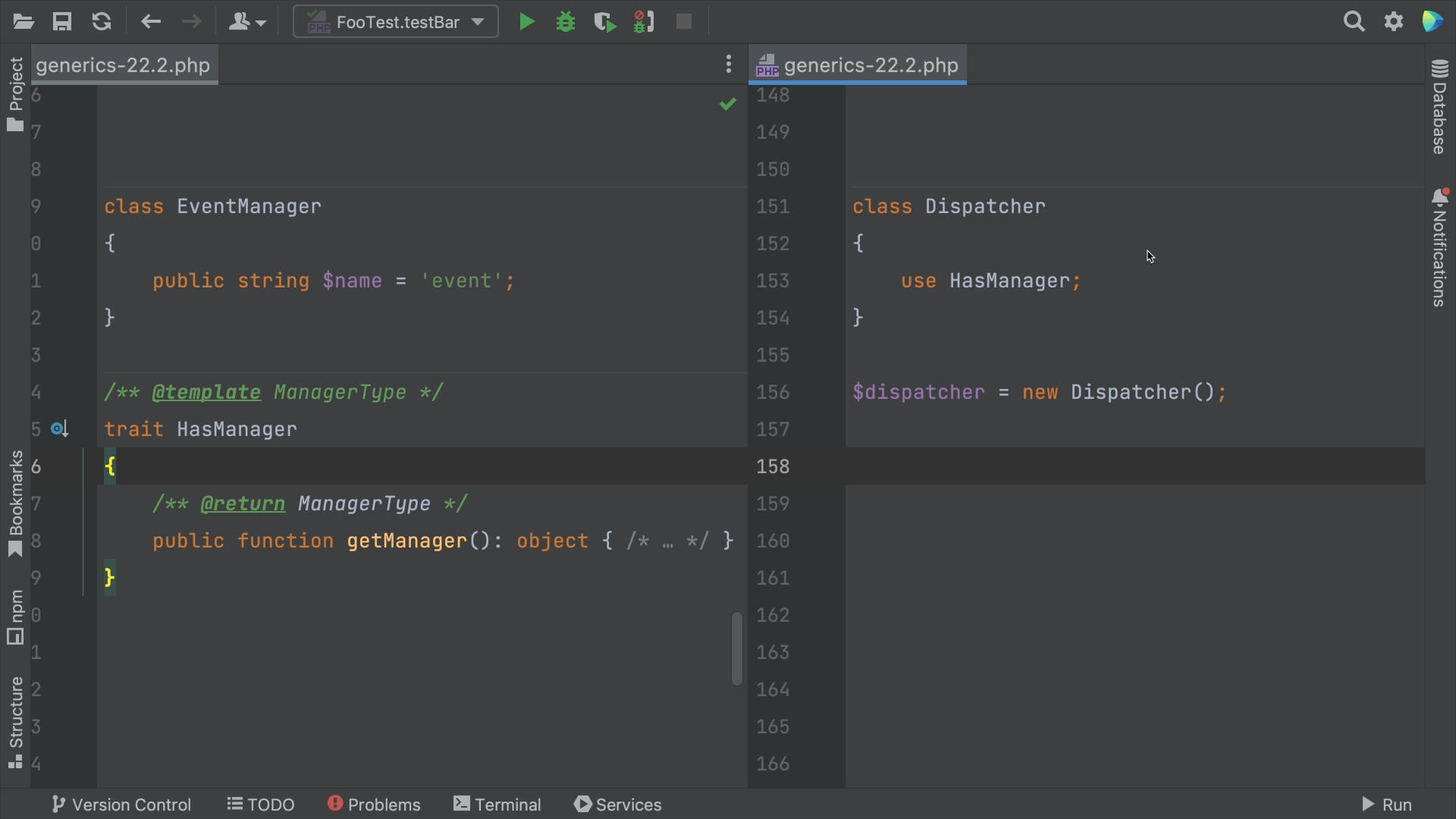
Support for by-passing generic types
PhpStorm now handles methods like Collection::lazy()
better, where generic types are proxied into another object:
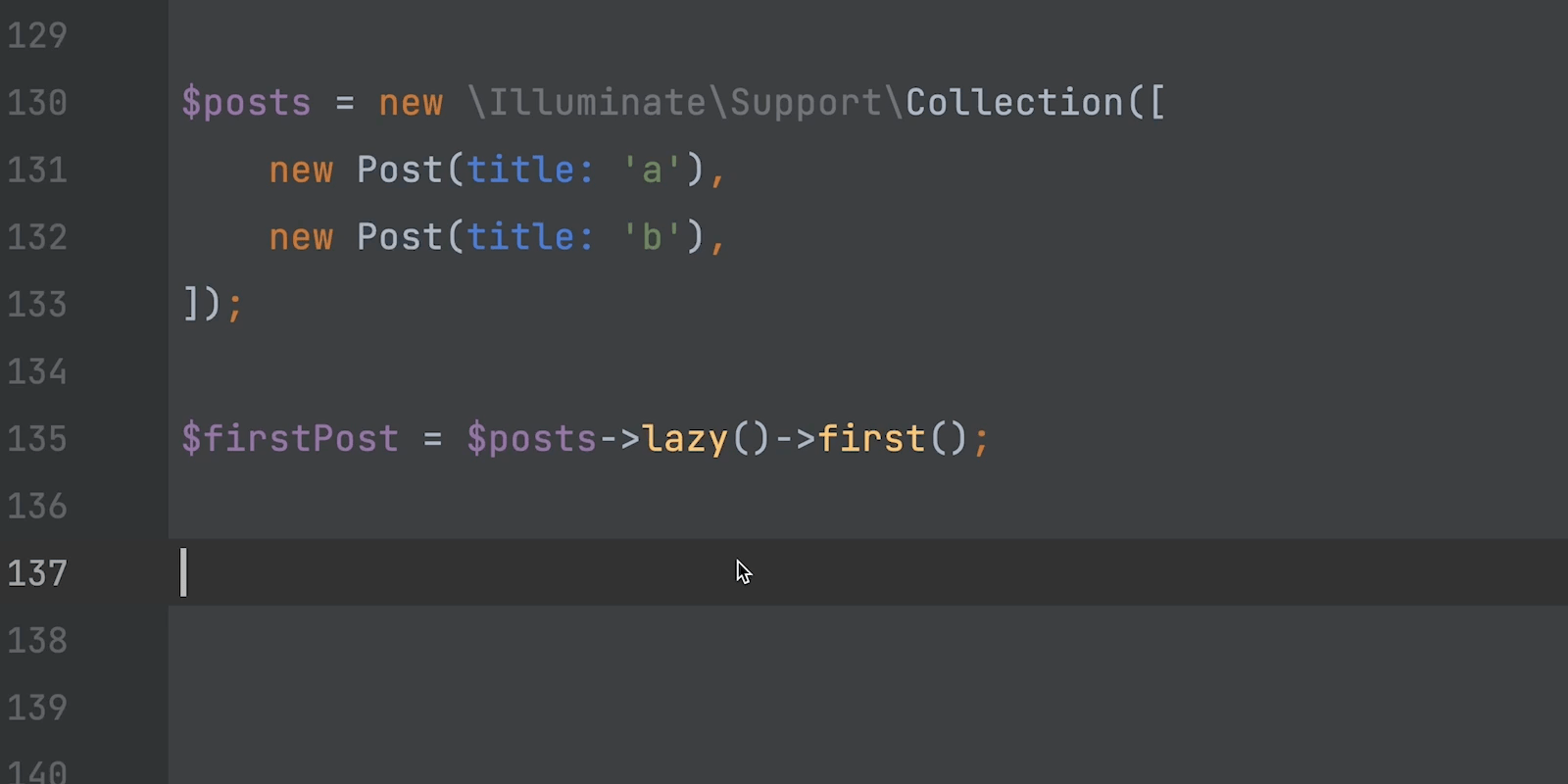
There are a lot more features:
- Improved support for nested generics (WI-66014).
- Flipping generic types (WI-66015).
- Support for generics in iterators –
Iterator<Type>
now works as expected (WI-62323). - Support for generics in iterables –
iterable<KeyType, ValueType>
now works as expected (WI-56037). @extends \SplFixedArray<Token>
now works as expected (WI-65964).- Improved generic type inference (WI-60891).
We’d like to keep improving our generics support, so don’t hesitate to submit an issue when you run into cases that aren’t supported yet.
Anonymous @var in return statements
You can now add an @var
docblock before a return statement to specify the concrete return value of a function without having to introduce a temporary variable.
Clickable URLs in PHP, JSON, YAML, and .properties string values
PHP, JSON, YAML, and .properties files now feature automatically inserted web references inside values that start with http:// and https://. You can easily open these links in a web browser with one click.
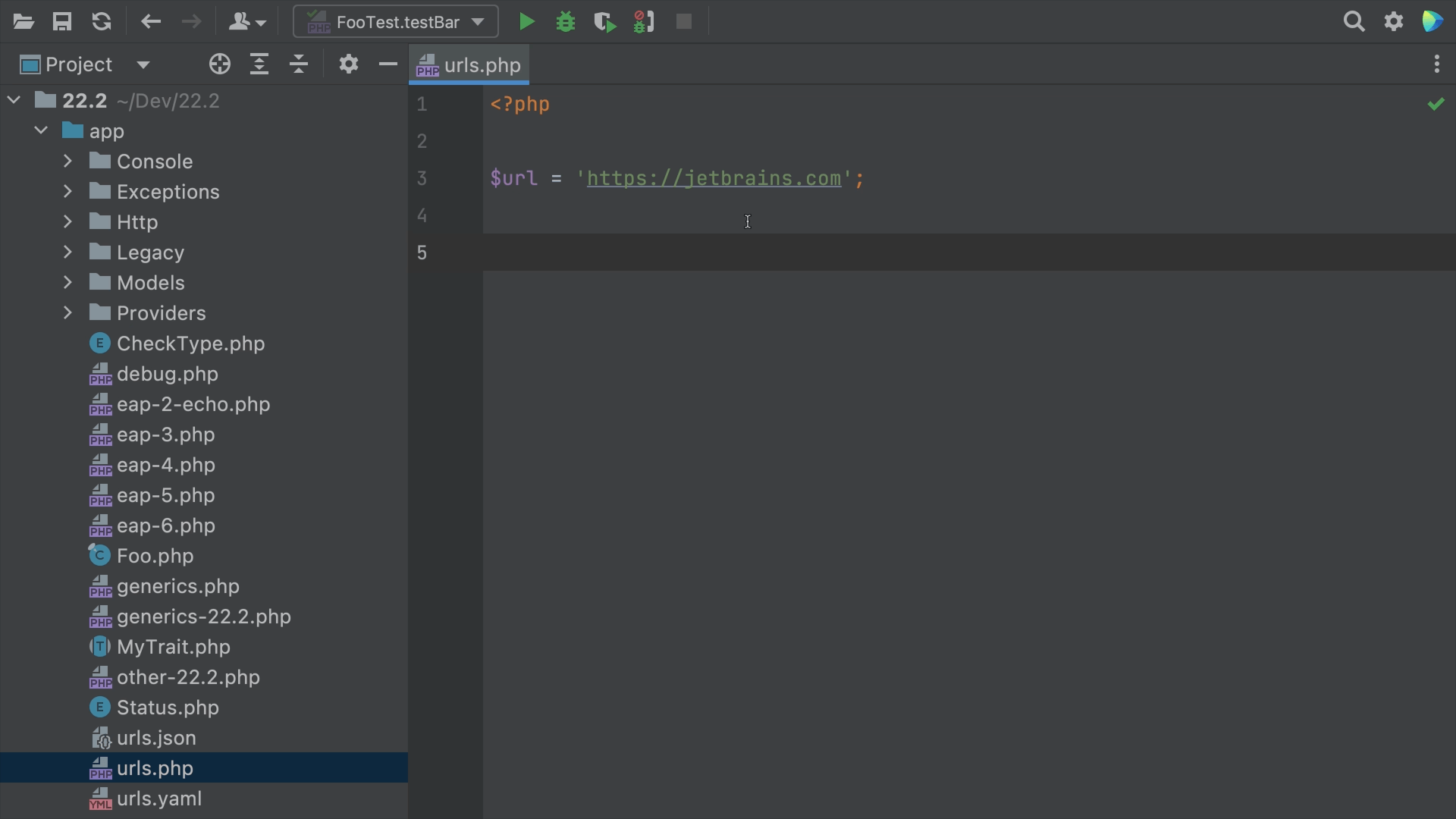
Blade: @props and @aware directives
We now support the @props
and @aware
directives in blade components.
Code Style: trailing commas
You can configure PhpStorm to automatically insert trailing commas in parameter lists, closure use lists, and function calls. Go to Settings / Preferences | Editor | Code Style | PHP | Code Conversion to configure these options.
Improved runtime and quality tools configuration
We’ve made significant improvements to how runtimes and quality tools are configured. You can now specify which PHP binary should be used for individual runtimes.
We also changed the name of the default local PHP runtime to System PHP to make what it’s about a little clearer. It was called Local before, which was a bit confusing.
You can select a different runtime for individual quality tools, and Xdebug will be disabled by default when running those quality tools.
There’s one case where Xdebug isn’t disabled by PhpStorm: when you have System PHP selected as the runtime for any specific quality tool. In that case, PhpStorm will use your system’s PHP installation without any changes to it. So if Xdebug is enabled in your system’s installation, PhpStorm won’t disable it.
Bundled VSCode keymap plugin
The VSCode keymap plugin is now bundled by default, meaning you no longer need to manually install it. Go to Settings / Preferences | Keymap to choose the VSCode keymap.
HTTP client improvements
We made several changes and improvements to our HTTP client – let’s go through them one by one.
WebSocket support
Our HTTP client now supports the WebSocket protocol. Use the WEBSOCKET
keyword to initiate a WebSocket connection.
GraphQL support
We’ve also added support for GraphQL requests in the HTTP client. PhpStorm can now send GraphQL queries over HTTP and WebSockets protocols out of the box.
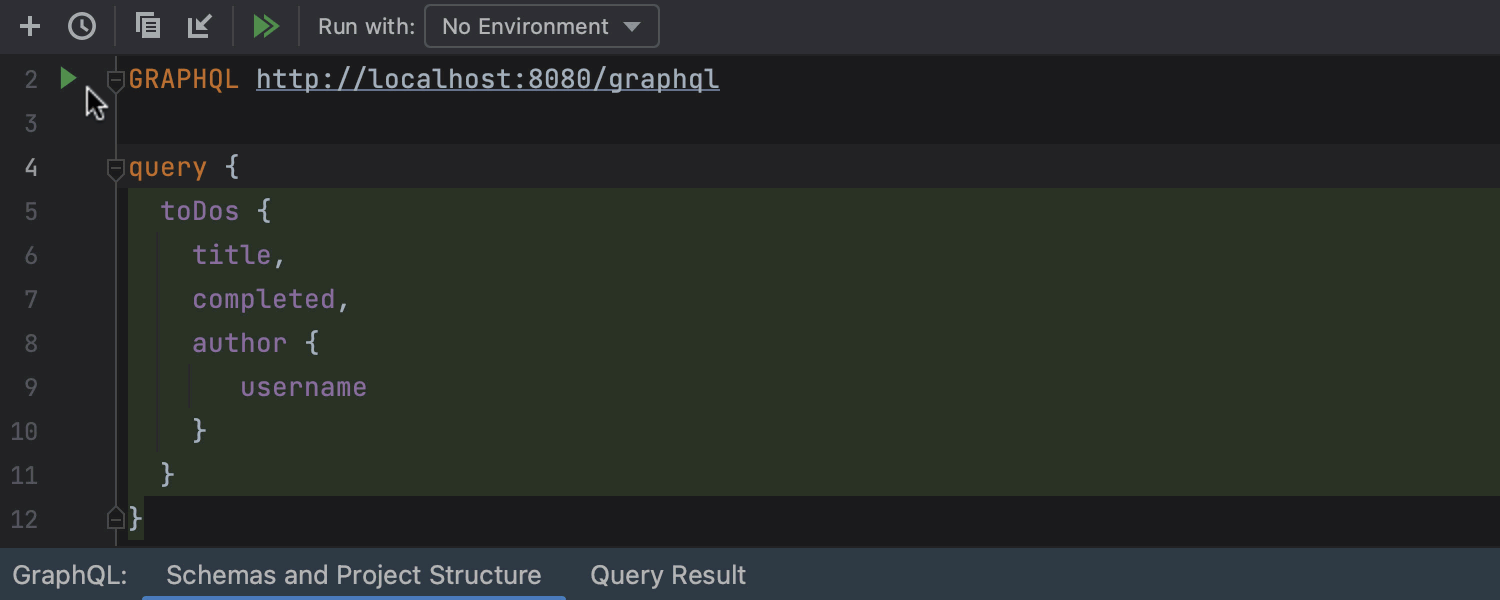
Run environment via the gutter icon
There’s a new convenient way to select a run environment using an icon in the gutter. To enable this feature, choose the Select Environment Before Run option from the Run with combobox.
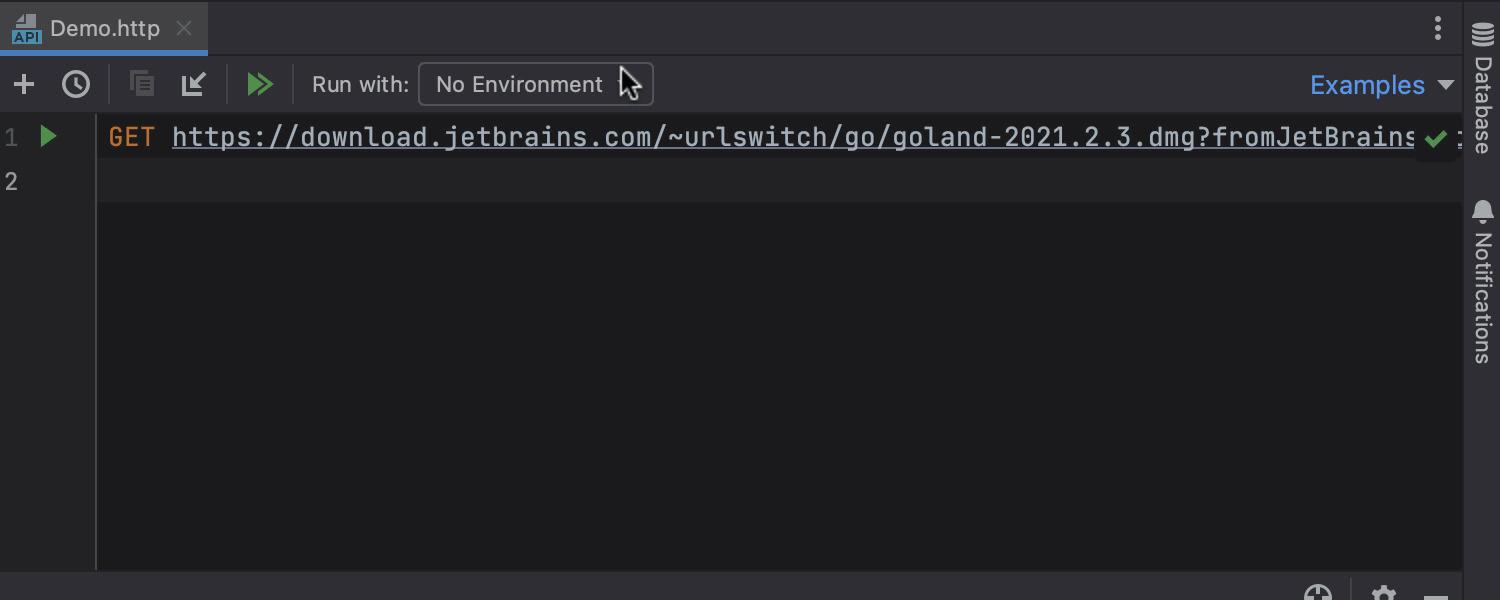
Response view progress bar
We’ve also improved the Response view by adding a progress bar that allows you to track the downloading process.
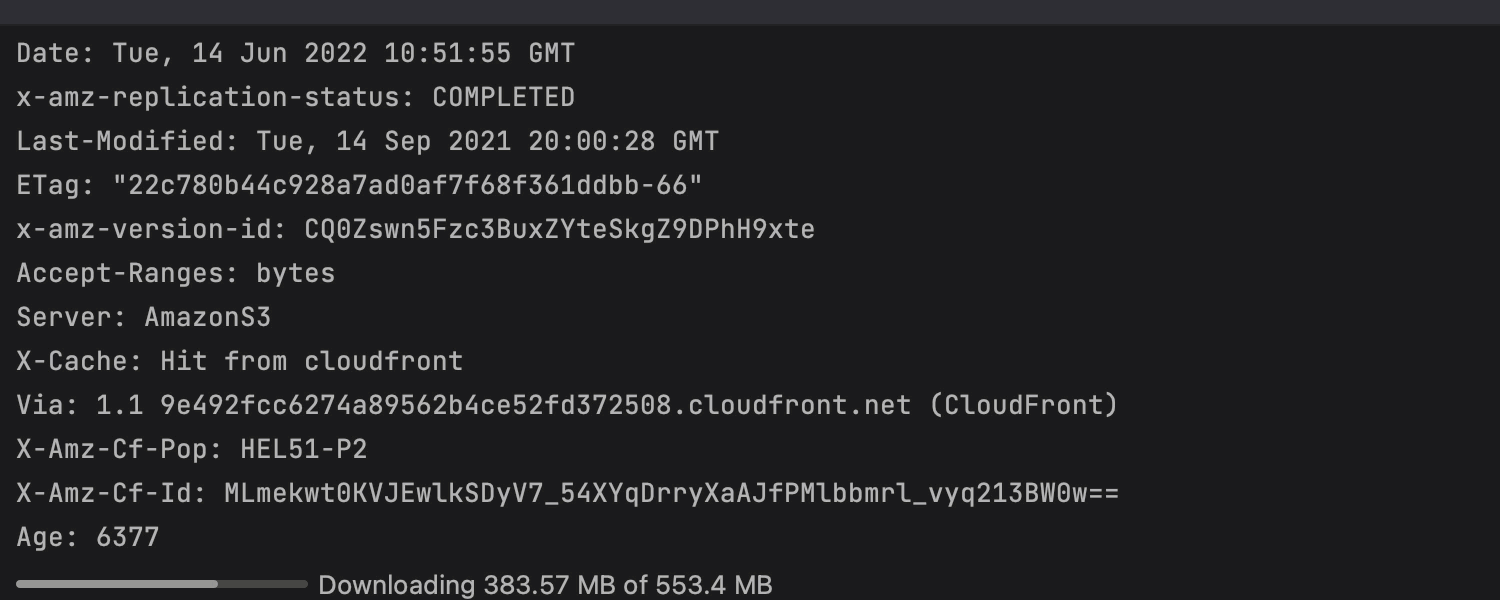
Docker auto-connection on IDE restart
PhpStorm now automatically connects to Docker after you restart the IDE. This new setting is enabled by default in Settings/Preferences | Advanced Settings | Docker.
Docker connection options for additional Docker daemons
PhpStorm is now integrated with Colima and Rancher to support more options for establishing connections to Docker daemons.
Keyboard shortcut to change the font size globally
For this release, we’ve resolved a long-standing feature request by introducing a keyboard shortcut that changes the font size across all tabs. To increase the font size, press Ctrl+Shift+.
/Alt+Shift+.
. To decrease it, press Ctrl+Shift+,
/Alt+Shift+,
.
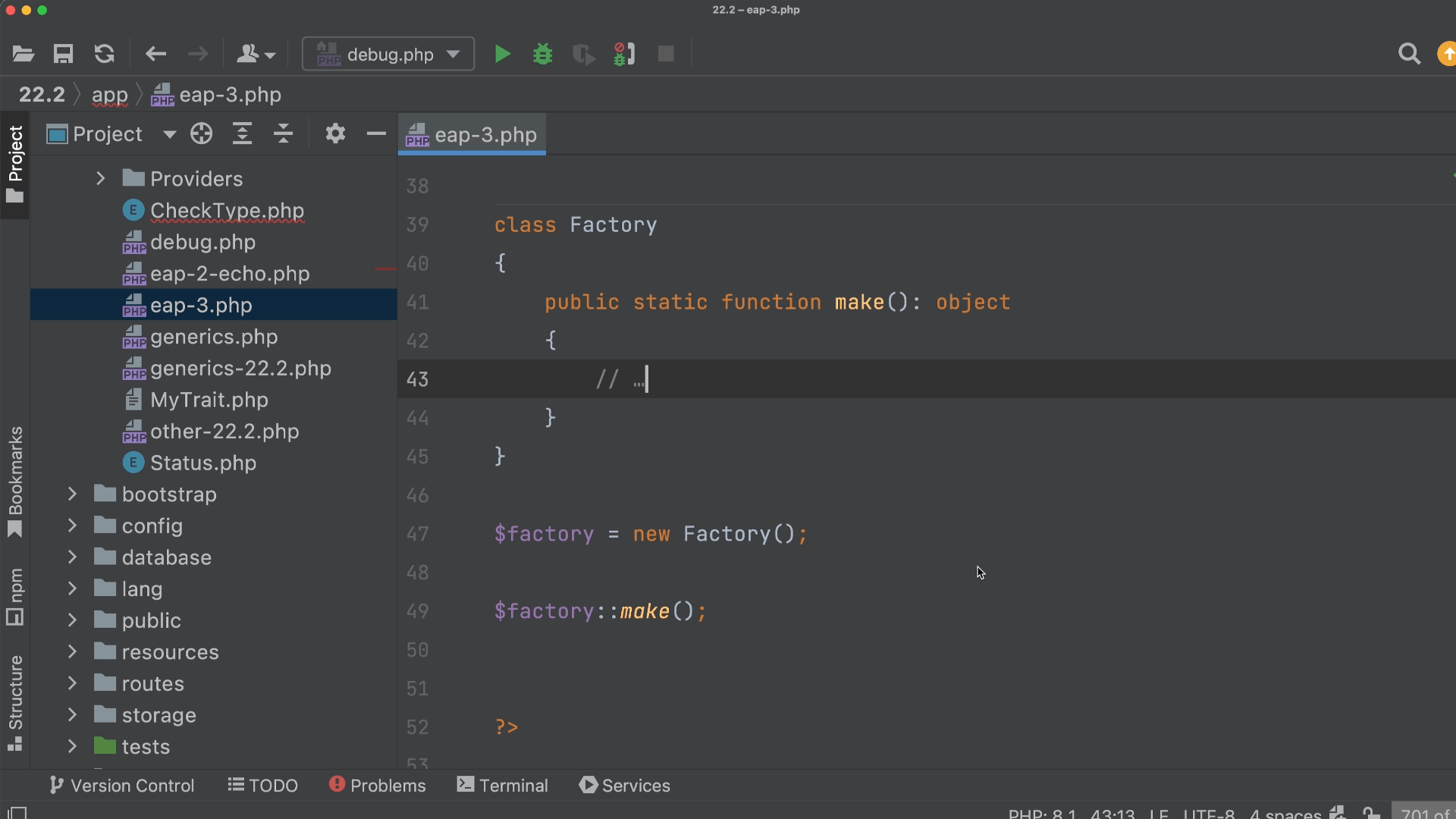
When you zoom into or out of your code within the editor, you will also see an indicator that shows the current font size and the option to revert it back to default.
Merging project windows on Mac
Windows on Mac supports a multi-tab view, and PhpStorm now allows you to merge all of your open project windows into this multi-tab view via Window | Merge All Project Windows:
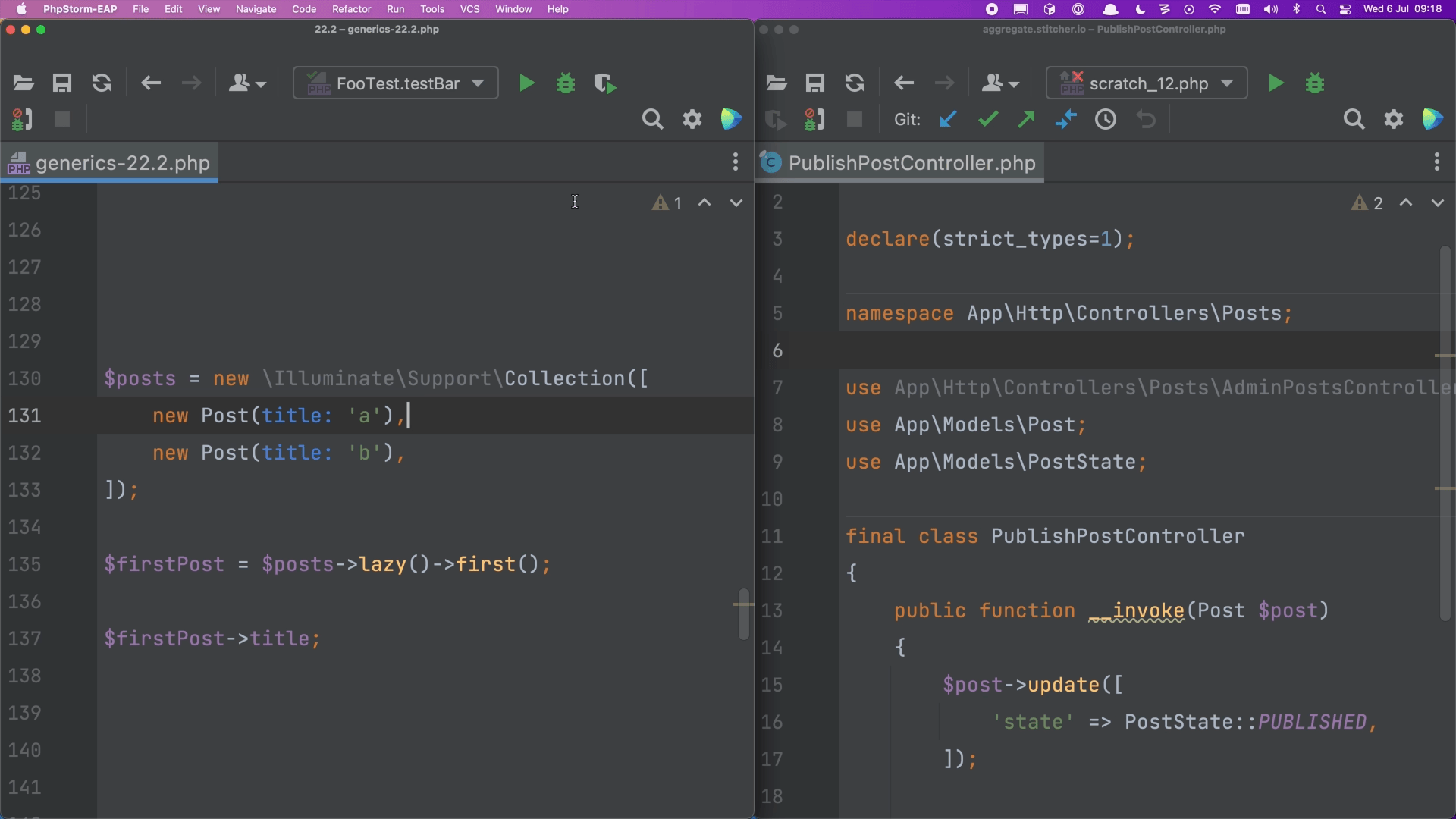
Markdown improvements
We’ve made a couple of improvements to markdown files. Let’s take a look.
Generating a table of contents
We’ve been working on making our Markdown support better. In this release, we’ve added a new Generate Table of Contents action that will make creating a table of contents for your Markdown files much more straightforward. You can use ⌘N
/Alt+Insert
, which brings up the Insert… popup. You can then select Table Of Contents, which will be generated automatically for you.
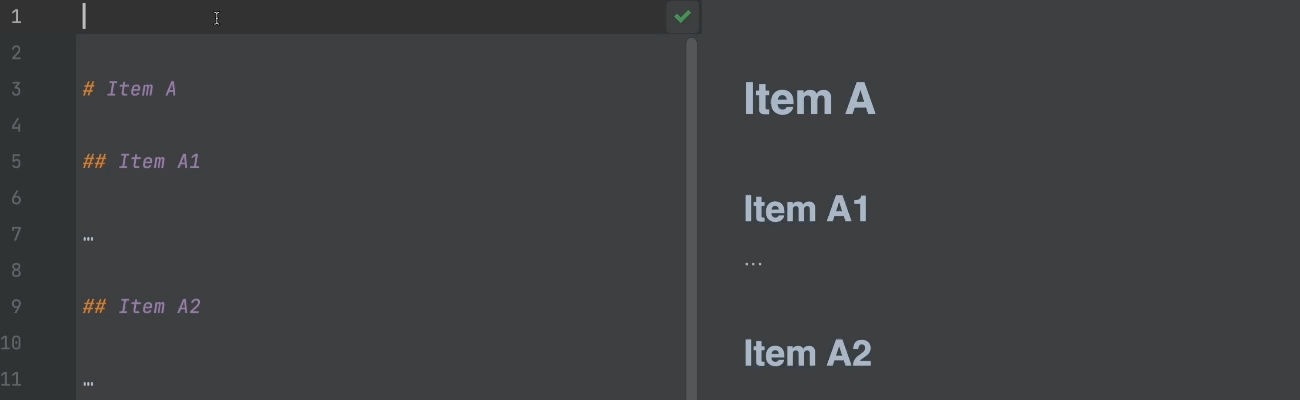
Experimental support for Front Matter in Markdown files
We’ve introduced experimental support for YAML Front Matter headers, which can be enabled via the markdown.experimental.frontmatter.support.enable
registry key.
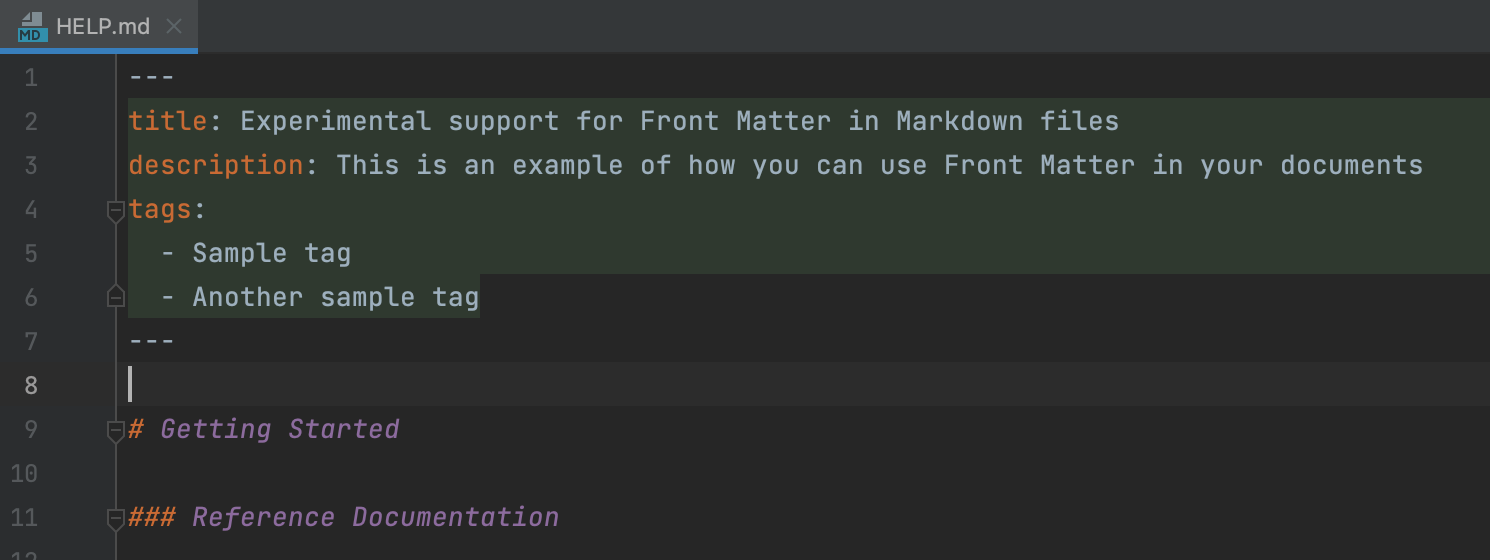
New Description field for mnemonic bookmarks
We’ve integrated a Description field into the Add Mnemonic Bookmark dialog so that you can add an optional description to your bookmark right away.
Improved support for web technologies
Here are a couple of highlights of what’s new when it comes to web technologies, you can take a look at this page to learn more.
Support for Angular standalone components
We’ve been actively working on support for Angular 14 in PhpStorm. The most important addition we’ve made in this release is support for Angular standalone components. PhpStorm now properly recognizes components, directives, and pipes marked as standalone: true
.
Updates for Vue 3
Our support for Vue 3 has lagged behind some of the latest Vue updates. We’ve shipped several improvements to address this, though some, such as Vite support, were already included in the minor updates for v2022.1. PhpStorm 2022.2 also comes with a few fixes, including understanding type narrowing in v-if/else
directives.
TypeScript 4.7 support
PhpStorm 2022.2 comes bundled with TypeScript 4.7, supporting new language features like moduleSuffixes and ESM in Node.js. It will automatically insert the .js extension to the import statement if the module is set to node16 or nodenext in your tsconfig.json file. Additionally, PhpStorm supports the typesVersions field in package.json files.
Faster Runtime
With this release, we are moving from JetBrains Runtime 11 (JBR11) to JetBrains Runtime 17 (JBR17). This will have the following effects:
- A significant performance improvement, allowing faster and smoother IDE operation.
- Better security, as JBR17 is based on the latest OpenJDK LTS.
- Better rendering performance on macOS, as JetBrains Runtime 17 leverages the Metal API.
- Increased accessibility on macOS, as JBR17 features integration with the VoiceOver screen reader.
- Usage of the Vector API, further improving performance.
The full list of changes in PhpStorm 2022.2 is available on the release notes page.
That’s all for today. Thanks for keeping up with the changes! We hope they improve your PhpStorm experience.
- Download PhpStorm.
- Tweet @ us!
- Report bugs to our issue tracker.
Your JetBrains PhpStorm team
The Drive to Develop