Fleet
More Than a Code Editor
Polyglot Programming Is a Thing
There are millions of professional software developers and even more learners and hobbyists who enjoy coding. Dozens of programming languages are in widespread use, many of which come with their own build systems and dependency management tools. Developers have a whole spectrum of tools to choose from, ranging from basic in-terminal text editors to fully-fledged IDEs. And then we have Fleet, yet another editor and IDE all rolled into one. Why?
Software developers speak many languages
Learning programming languages is fun. It’s always hard to resist trying a new programming language – the one everyone is talking about because it’s somewhat fresh-looking, fashionable, performant, safe, and so on. The current market situation is also putting pressure on software developers to acquire new skills, such as additional programming languages.
In the StackOverflow 2023 Developer Survey, there was a question about which programming languages the respondents work with. The report presents numbers of users for every language, but it doesn’t say anything about language combinations. Fortunately, the raw data is available. For example, 22 participants checked every suggested language (and may have been exaggerating their skills in doing so). Even more interestingly, about 12% of participants checked only one language.
What are the most popular language combinations? I’ve come up with the following heatmap to visualize the answer to this very question (many thanks to JetBrains AI Assistant for helping; it would’ve surely taken much longer without it):
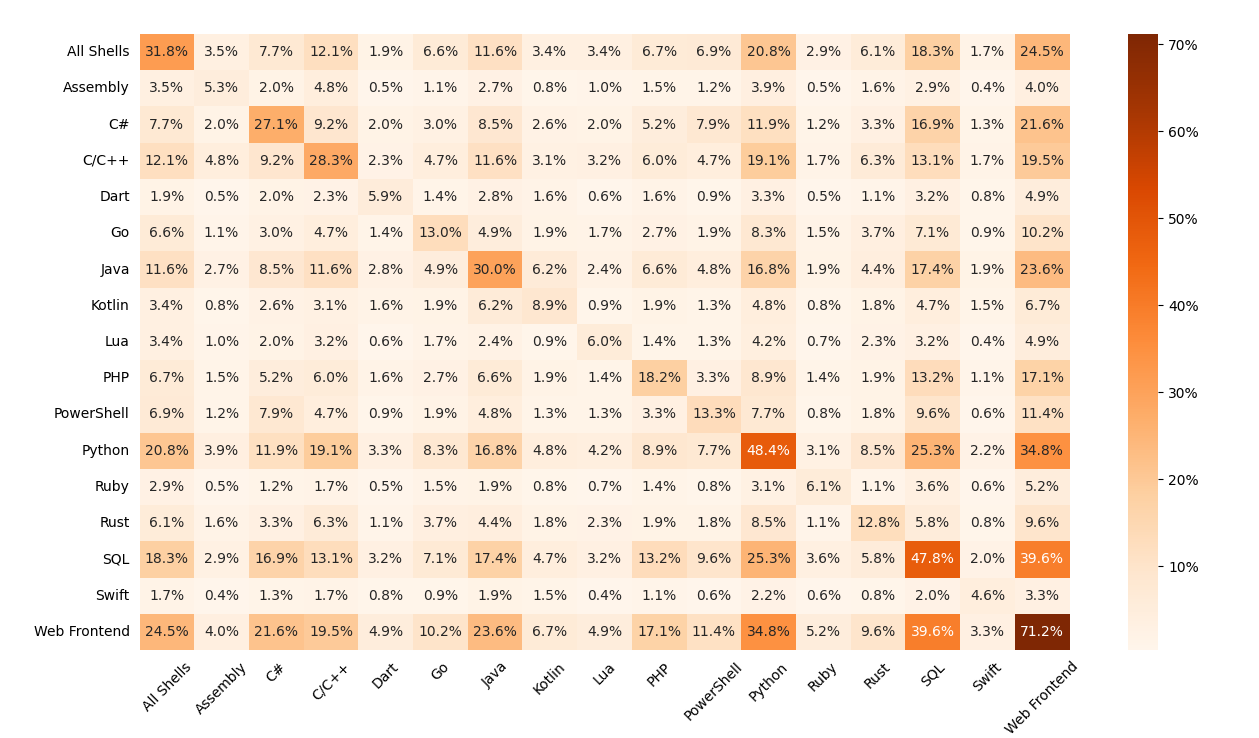
A couple of minor caveats: In creating this heatmap, I merged all frontend development languages into one group called “Web Frontend”. I also counted C and C++ together.
The main diagonal in this diagram shows the number of users for every language, while everything else refers to pairs of languages. For example, 6.7% of the StackOverflow survey respondents mentioned that they work with Kotlin alongside some of the Web Frontend languages. About 17% work with both Python and Java.
Further analysis of the raw data tells us that about 5% of developers work with all of the following languages:
- C or C++
- Java
- Python
- SQL
- Web Frontend
So, software developers these days know and work with more than one programming language – perhaps not simultaneously, but nonetheless the statement stands.
Software is written in many languages
A programming language is chosen at the earliest stages of a new software project. For existing projects, migrating to another language is also an option. These decisions are informed both by the existing knowledge within the team and the availability on the market of certain domain specialists. At the same time, writing or rewriting everything from scratch is rare, as there are useful frameworks to start with and libraries for implementing pieces of required functionality. These frameworks and libraries may belong to different language ecosystems. As a result, there are software projects with different languages coexisting in codebases for years. Over time, language combinations are becoming increasingly intricate and build processes are becoming more and more interwoven.
A simple GitHub search proves that multi-language projects are widespread. Some time ago, I looked at the 1,000 most popular (by number of stargazers) projects on GitHub with Rust as a primary language, and I wondered what other languages lived alongside Rust in those repositories. Every fifth project contains code in C/C++ as well, while slightly fewer contain Python code. At the same time, more than 60% of those repositories contain files related to Web Frontend languages. Here’s a quick visualization of the data:
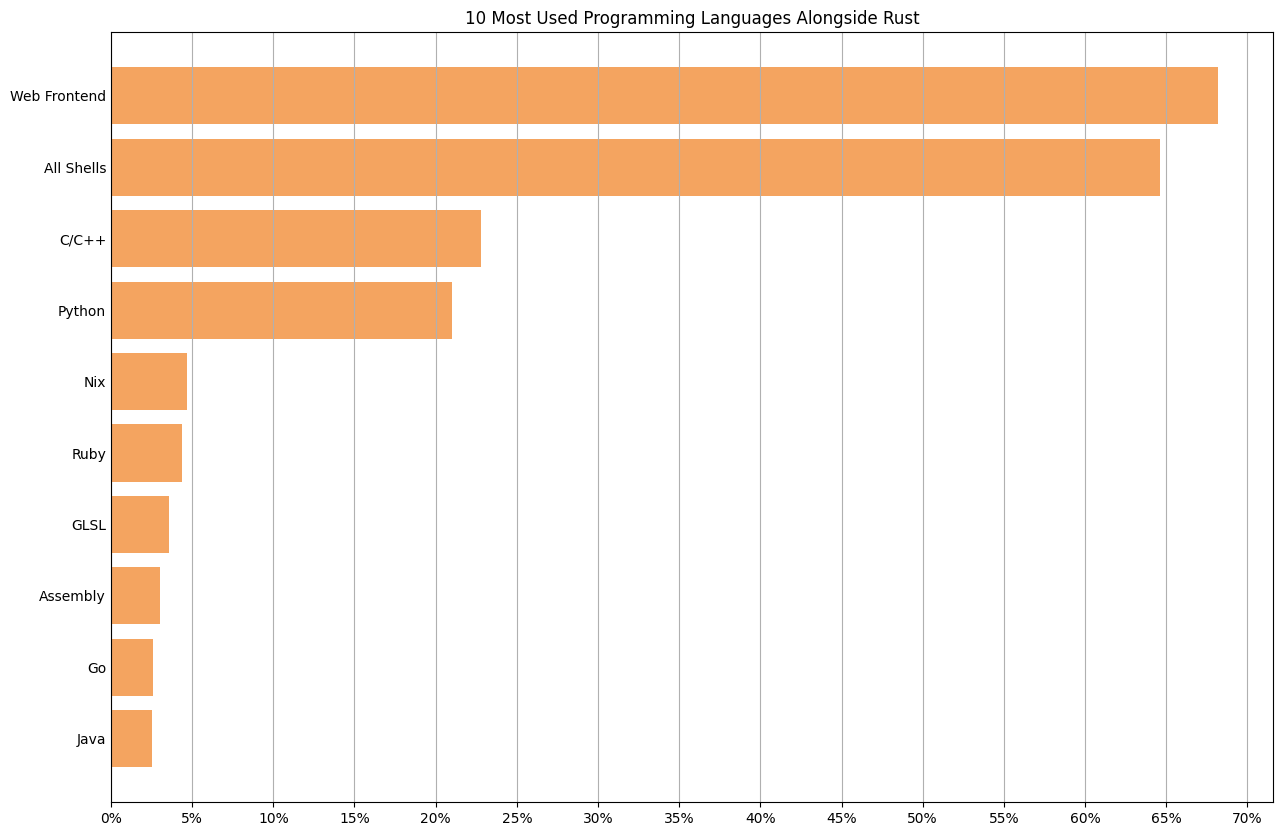
Of course, this is far from rigorous scientific research. I didn’t even look at the repositories with primary languages other than Rust. Still, all the information I was able to acquire from my peers indicates that multi-language software projects are ubiquitous.
One IDE to code them all – without the hassle
Let’s say you have a multi-language project. How long does it take to get everything up and running? It’s not unlikely that you’ll have to spend hours figuring out which add-ons you need, then installing and configuring them, and finally integrating them.
Luckily, there’s a faster way. Fleet already supports a dozen popular programming languages out of the box. In most cases, you don’t need to configure anything at all. Just open your project folder in Fleet and start coding immediately, or enable Smart mode to receive IDE-level coding support. That’s it!
One of the key ideas behind Fleet is to provide a unified experience for every supported programming language. This concept makes it easy to switch between languages as needed and is future-proof to boot.
But what exactly should a unified experience entail? For example, Go To Definition can theoretically be supported for any code in existence, from the level of an assembly to a quantum computer program. This is also true for renaming or syntax highlighting. At the same time, we realize that the specifics of implementing these features is heavily dependent on the particular language.
Let’s abstract a little bit. How do we work with code in general? These are some common scenarios:
- Exploration: We’re exploring a project’s codebase for the first time. We need to read a lot of code. Hence, we require some syntax highlighting and brace matching functionality. This might include the option to inspect a file’s structure, code folding (I personally use that a lot!), the ability to query information about code elements (Hey, what’s the type of this thing again?), and a means of navigating through code and documentation. Quick access to a VCS history also helps a lot.
- Fixing a bug: We’re tasked with fixing some particular issue. In addition to all of the above, we’d certainly appreciate code completion, debugging, and the ability to run code and tests. Using external tools, such as Docker, is not uncommon within this scenario. Once we finish coding, we may want to run a code formatter, commit, and push our changes.
- Refactoring: We’re refactoring existing code to make it more readable, less error-prone, and easier to maintain. Automatic refactorings such as renaming, extracting, or inlining variables or methods are indispensable in this case. We’d also appreciate being able to run tests.
- Writing new code: We’re writing new code (to implement a new feature, for example). Streamlined typing, which includes indenting on typing, inserting paired braces, and live templates (see, for example, the list of live templates for Java), and powerful code completion are must-have tools here. AI assistance is also a handy feature for these kinds of tasks.
In all these scenarios, it helps to have a feature-rich IDE at our fingertips. If we look at the feature matrix for Fleet, we see that it matches our expectations for what an IDE provides: a unified experience in various languages. But we can also see some room for improvement. Some languages have better support than others, although the lesser-supported languages are still entirely usable.
Let’s look at a small, artificial, and extreme example of a multi-language software project and make sure that we can use Fleet to work with it.
Distributed calculator: polyglossia in the extreme
My distributed calculator demo project is an adaptation of the distributed-calculator tutorial from the beautiful collection of Dapr quickstarts and tutorials. I’ve expanded upon the original project by adding more programming languages that power the same basic arithmetic operations:
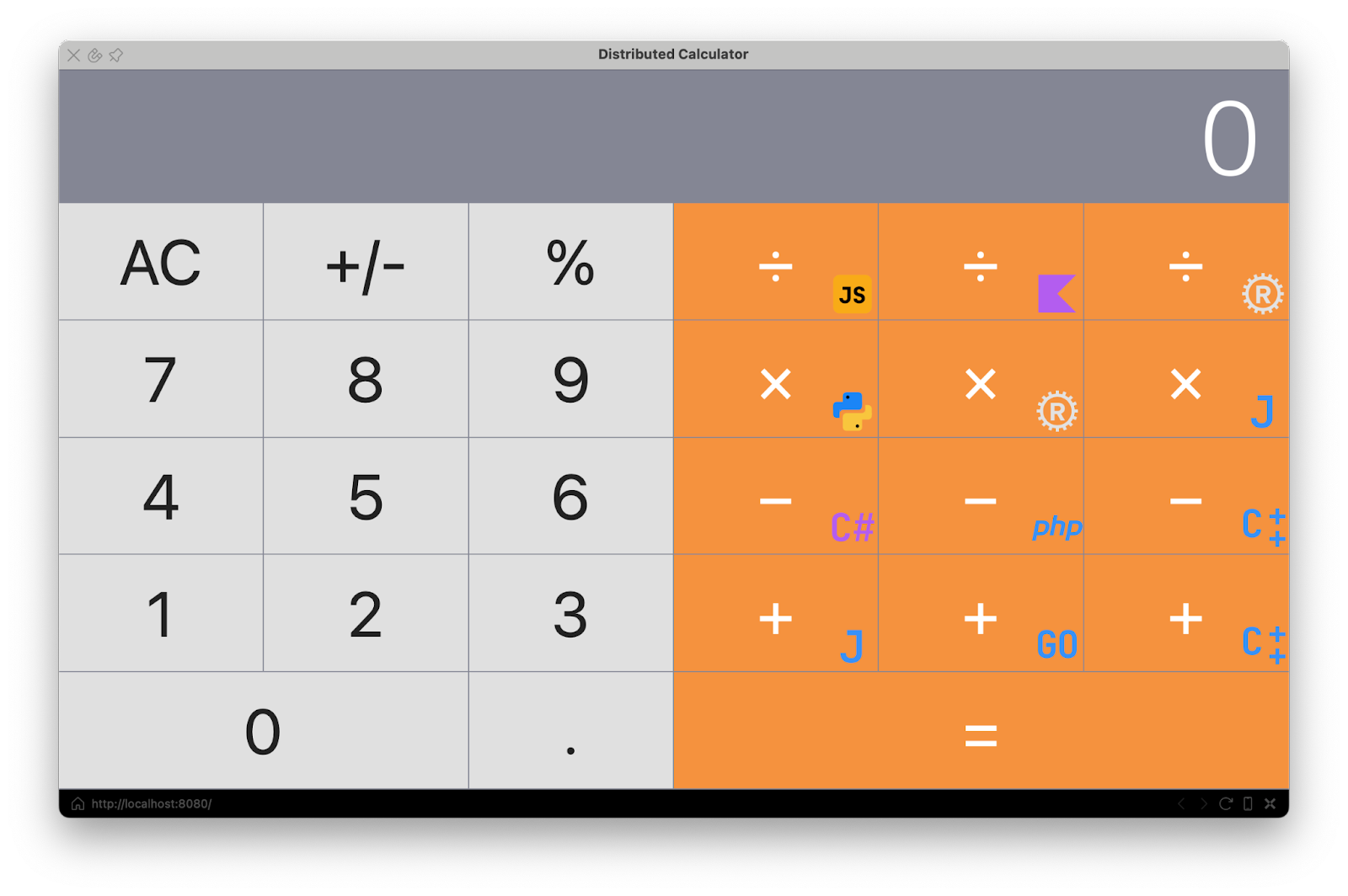
Every operation here is backed by several microservices written in different programming languages. Dapr itself is responsible for orchestrating those services and supporting their communication with the frontend application.
Now let’s dig into a few features – run configurations, smart mode, integration with Git and Docker, and AI assistance.
Run configurations
Even the most powerful and knowledgeable IDE won’t ever know how to handle polyglot projects with a variety of build systems in use. In this project, I have an extremely flexible and versatile home-grown shell-script-based system consisting of the start-service.sh and stop-service.sh scripts.
For example, to run the Rust service for multiplication and division, you need to execute the following command:
./start-service.sh rust-calc
That then expands into the somewhat magical:
cd rust && dapr run --app-id rust-calc --app-port 5002 --dapr-http-port 3508 cargo run
Sorry for hard-coding everything in the worst possible way. Of course, no real industry-level project would ever do things this way… would it?
To teach Fleet our project-specific run configurations, we use the .fleet/run.json file:
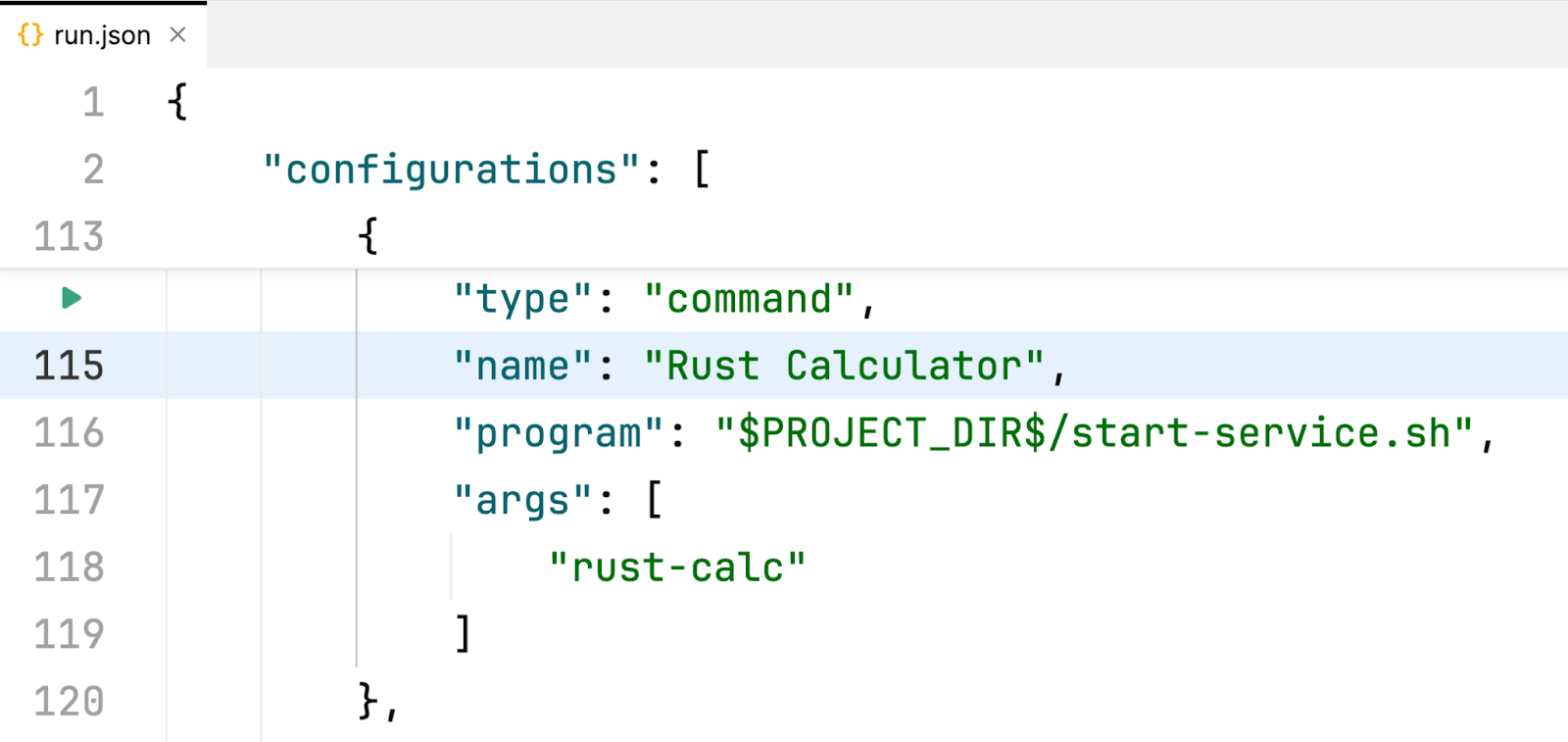
Note that you can run the desired configuration right from the gutter icon. All the run configurations defined in .fleet/run.json are also available in the Run & Debug dialog:
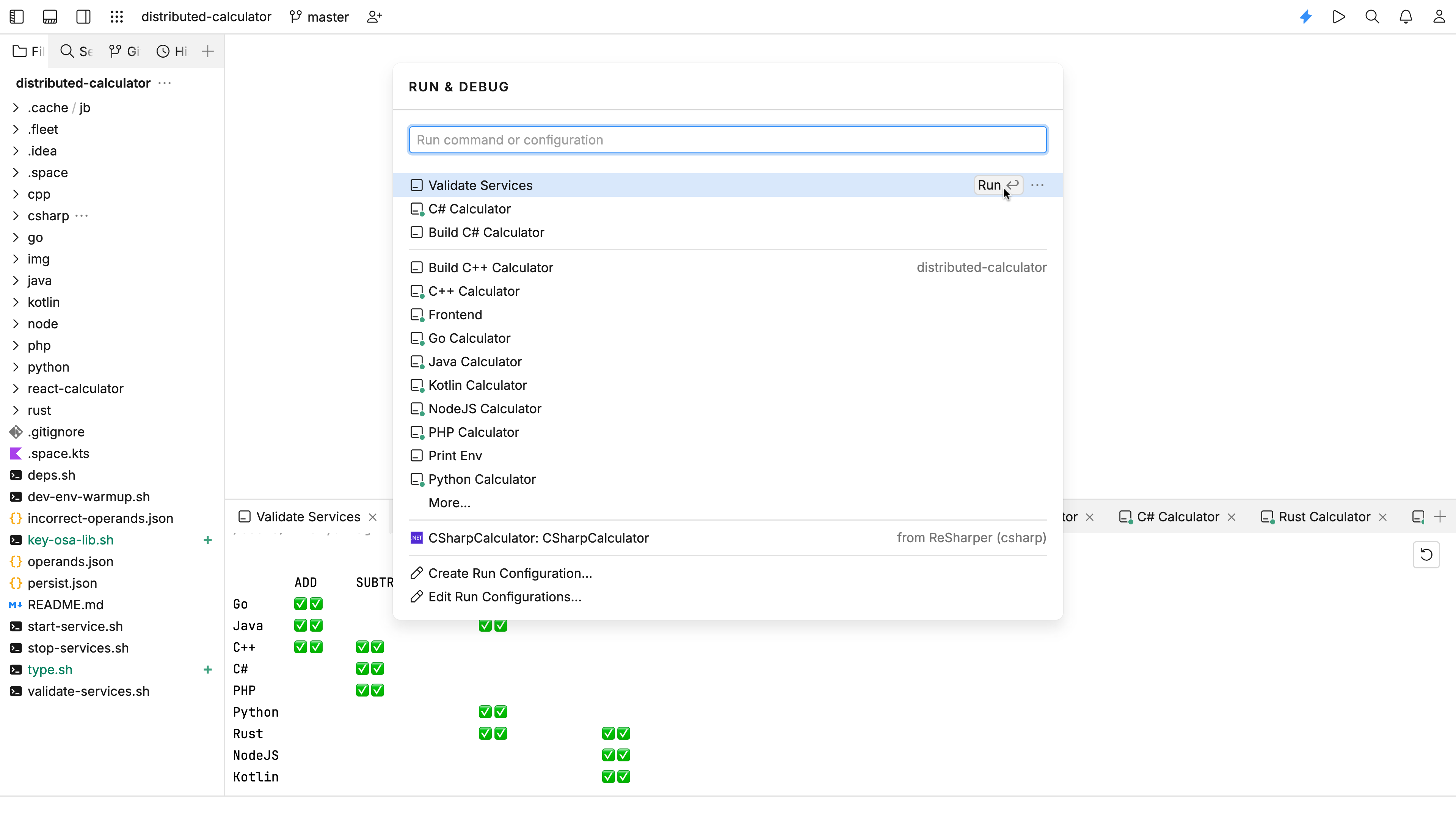
The Frontend service can also be launched via the Run & Debug dialog. Once it’s up and running, it will be available at http://localhost:8080/.
Integration with Docker
Dapr supports storing individual components’ states in Redis by running the corresponding Docker container. We can explore the state directly from Fleet by opening the terminal in the container from Fleet’s Docker dashboard:
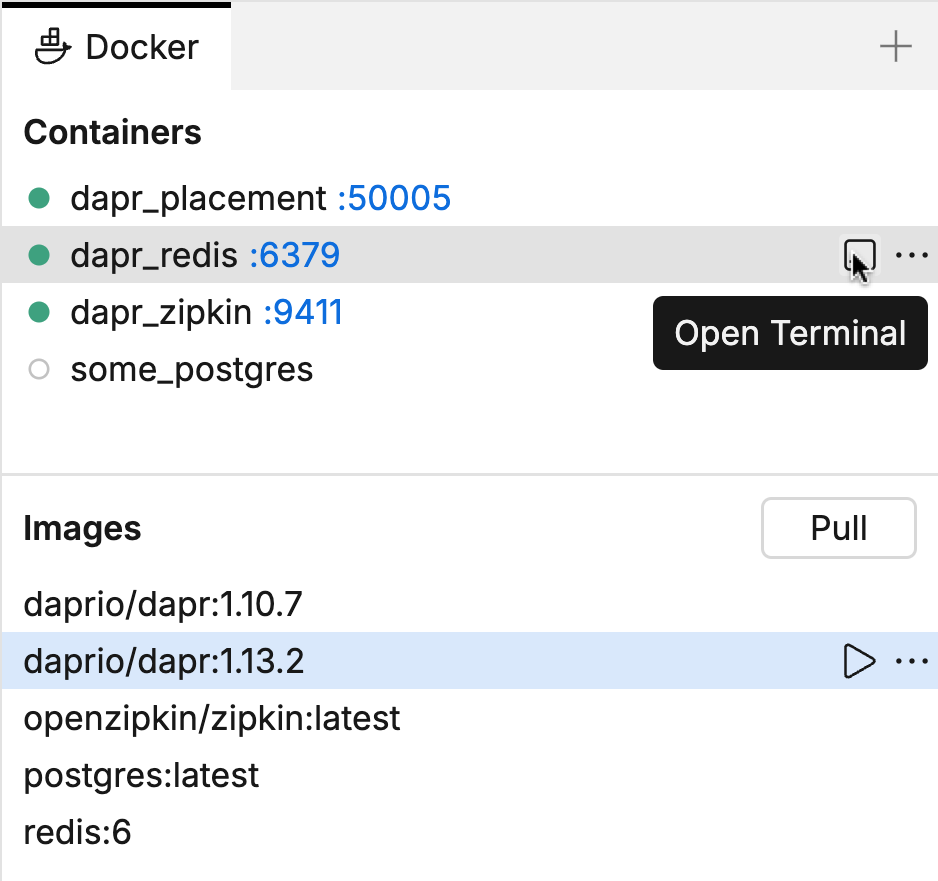
While we are at the container’s terminal, we can launch redis-cli and talk to it:
root@421f310af85b:/data# redis-cli 127.0.0.1:6379> keys * 1) "frontendapp||calculatorState" 127.0.0.1:6379> hgetall "frontendapp||calculatorState" 1) "data" 2) "{\"total\":\"2\",\"next\":\"3\",\"operation\":\"+\",\"service\":\"go\"}" 3) "version" 4) "89" 127.0.0.1:6379>
But of course, Fleet is not a deployment tool. It’s an editor and an IDE, so let’s focus on those aspects.
Fleet as an editor for code
There are plenty of features available in Fleet right after opening your project. For example, syntax highlighting is already there for you. Let’s look at available implementations of the addition operation:
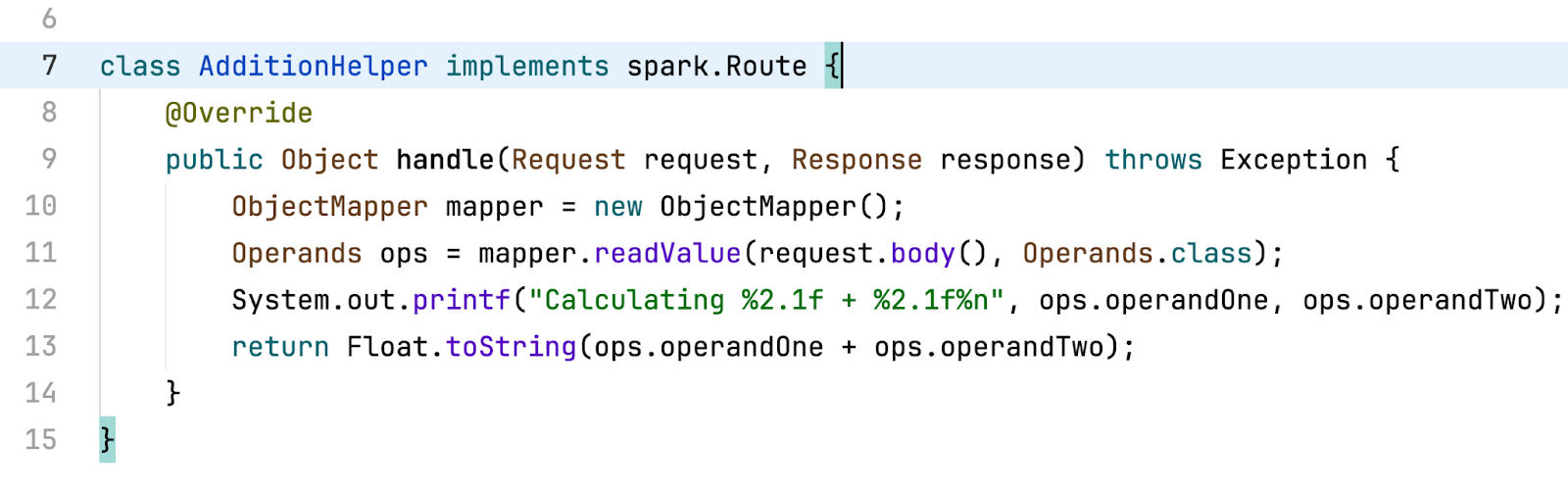
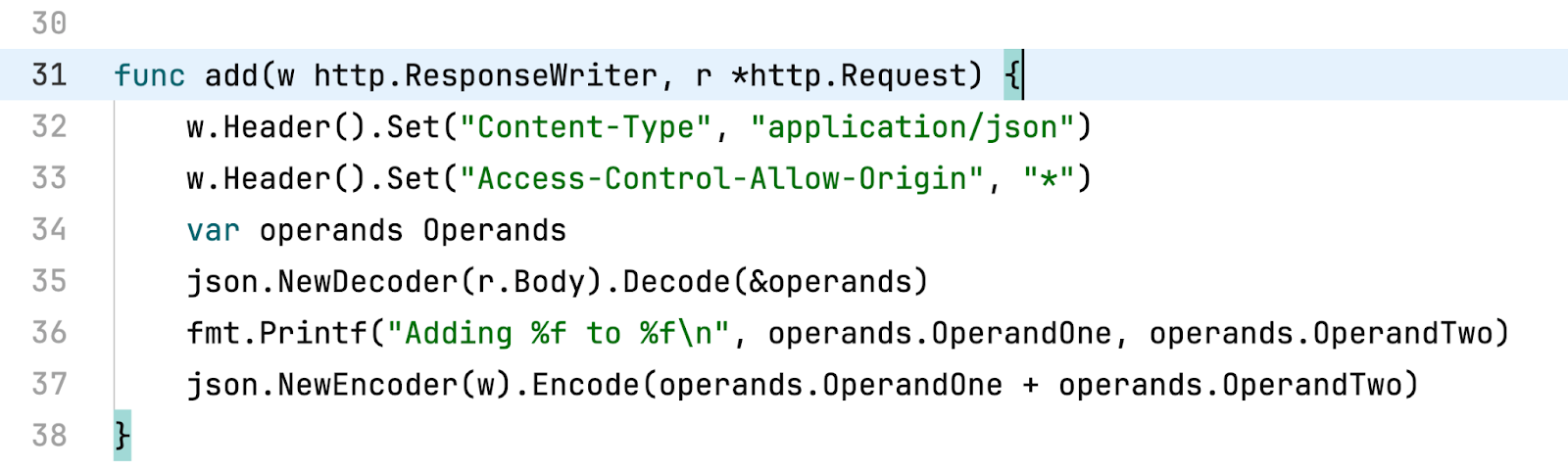
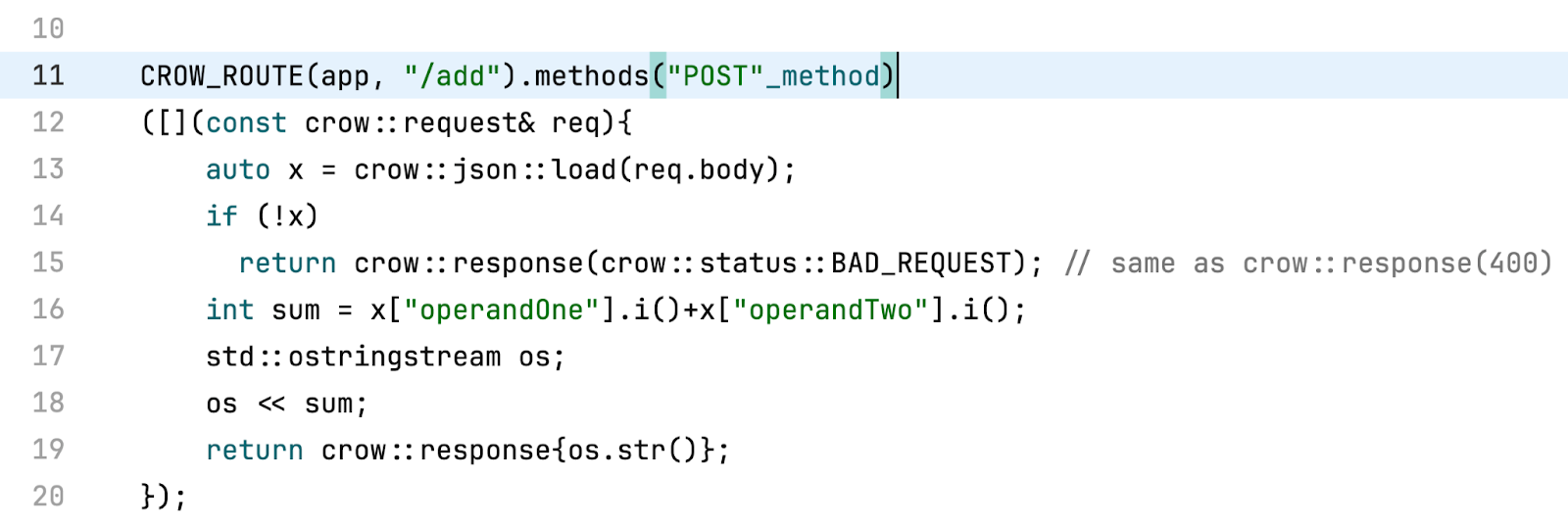
Can you recognize the languages used in these code fragments?
You can also enjoy code folding like in this PHP Symfony implementation of the subtraction operation:
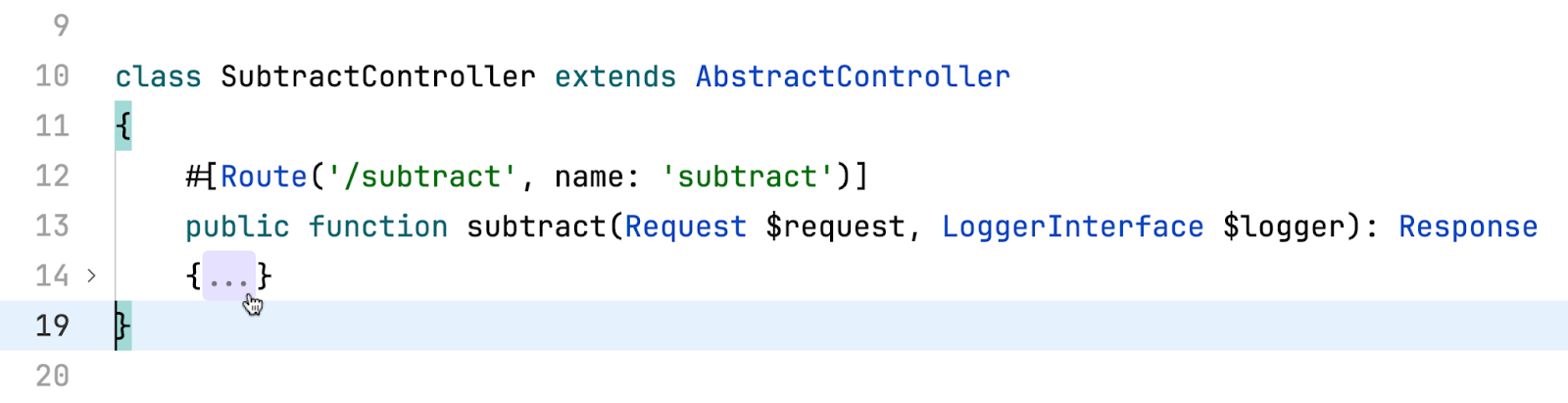
Of course, indentation on typing works out of the box. You can also easily comment out anything you want, even if you don’t remember the syntax for comments in the specific language you happen to be working with. Simply use the Toggle line comment action or fire the corresponding shortcut (⌘/ on macOS or Ctrl + / for other operating systems):
Fleet even knows your files’ structure and helps navigate quickly:
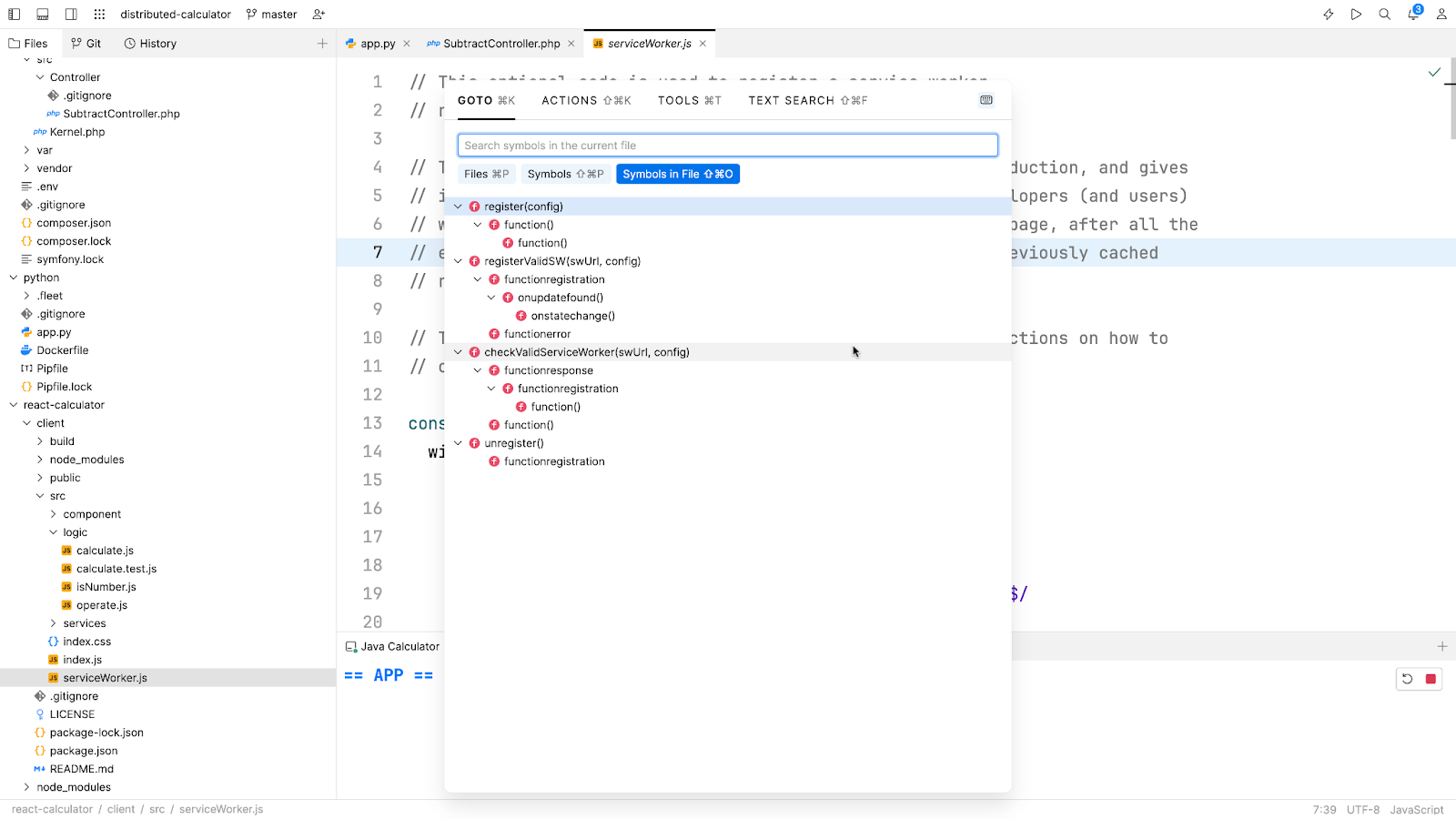
In the unlucky event that Fleet doesn’t speak your programming language, it supports TextMate bundles. You just need to add a bundle for the language you want to use. I use this feature for Haskell and Zig. For example:
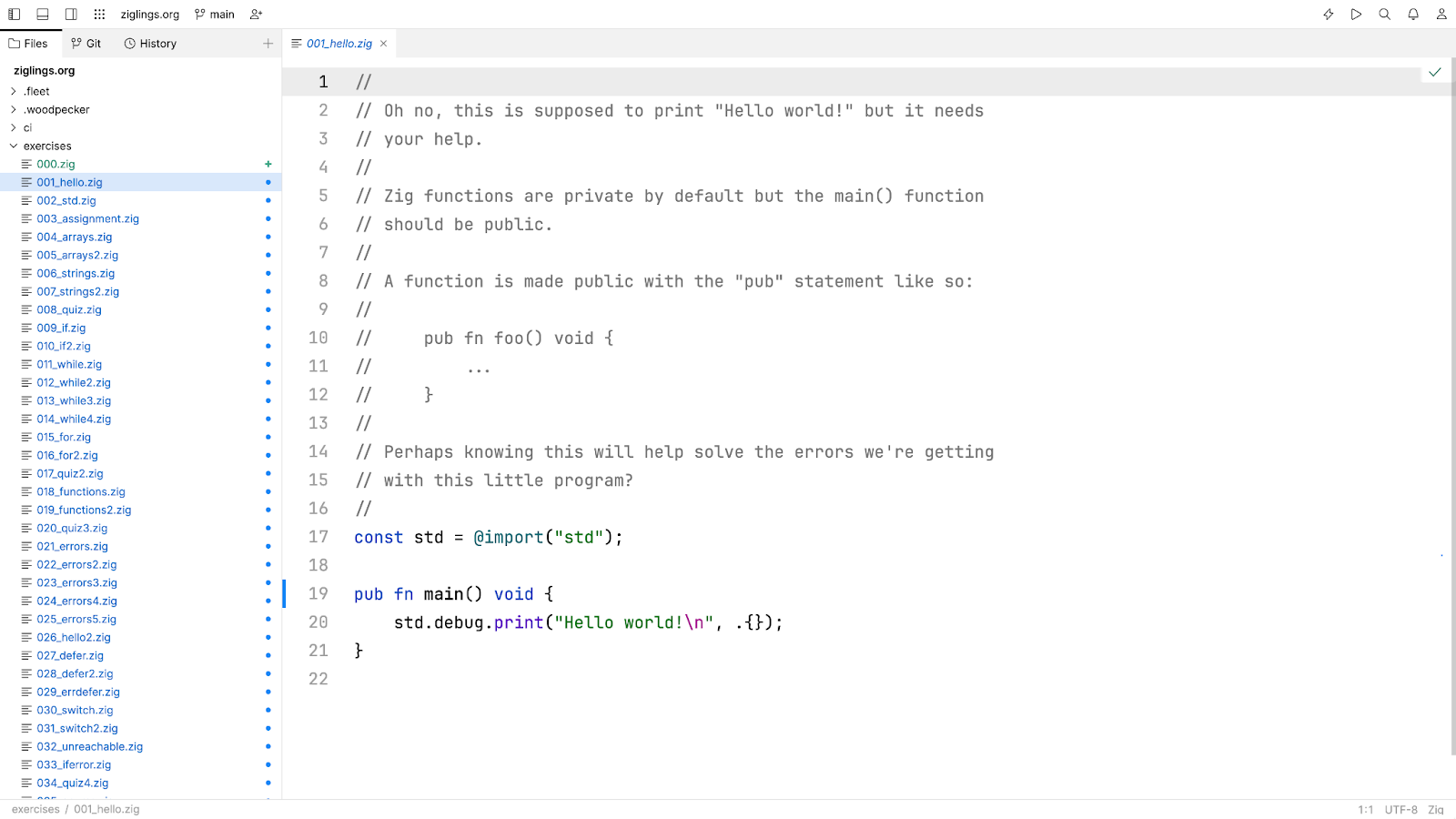
Maybe I need to add these two to the distributed calculator project as well…
A glimpse at Smart mode’s features
With Fleet’s Smart mode, you get much more powerful support. To start, you can refresh your memory using the quick documentation popup:
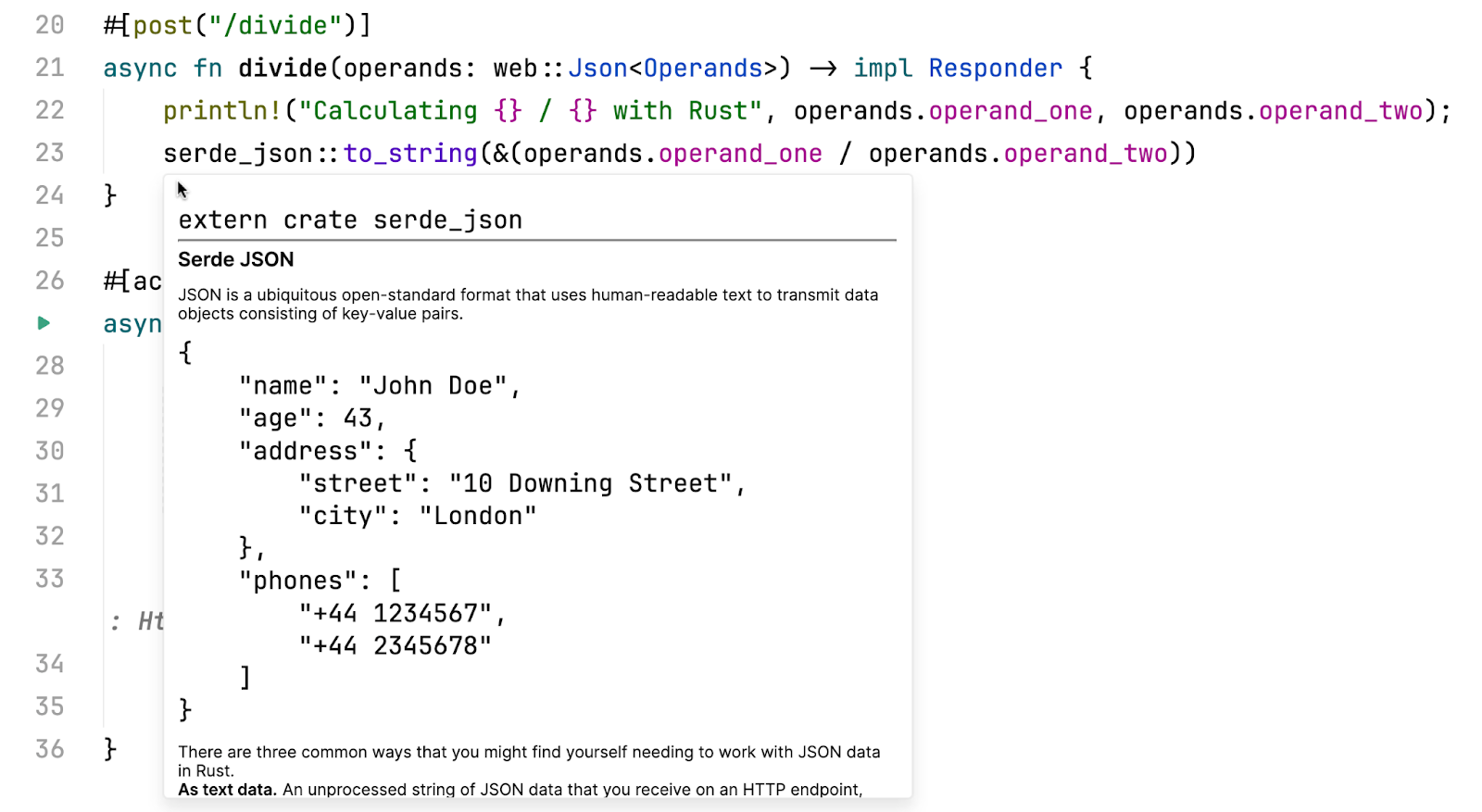
Or, you can quickly navigate to usages or definitions:
Of course, you get code completion as well:
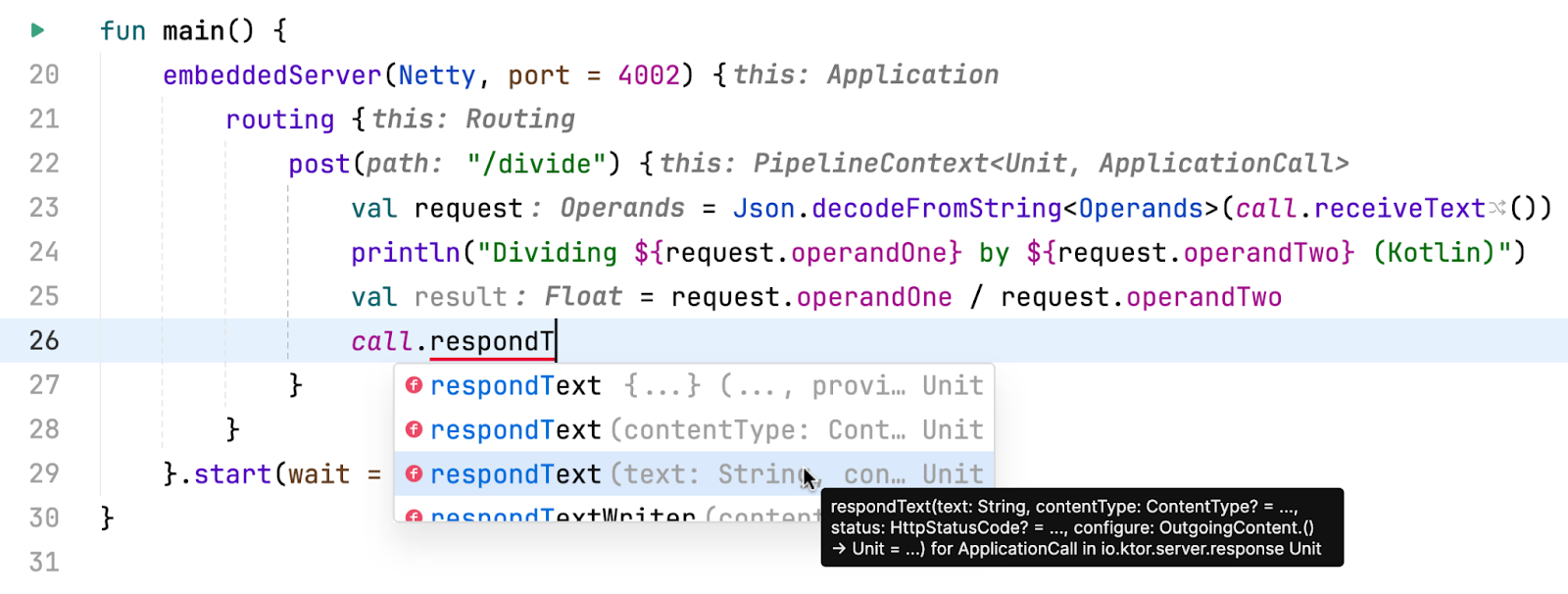
And if a linter is unhappy with your code, we’ve got you covered:
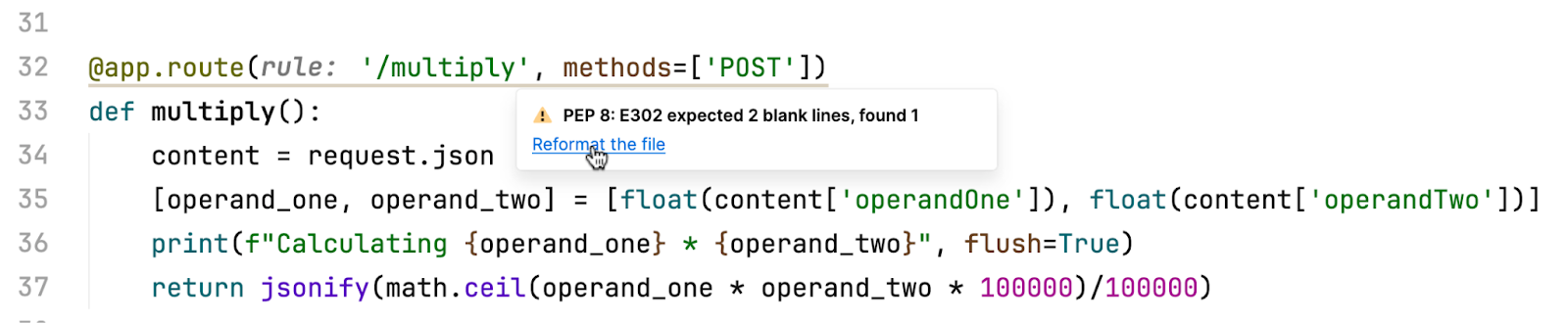
The Rename refactoring is useful to those who care about names (as we all should!):
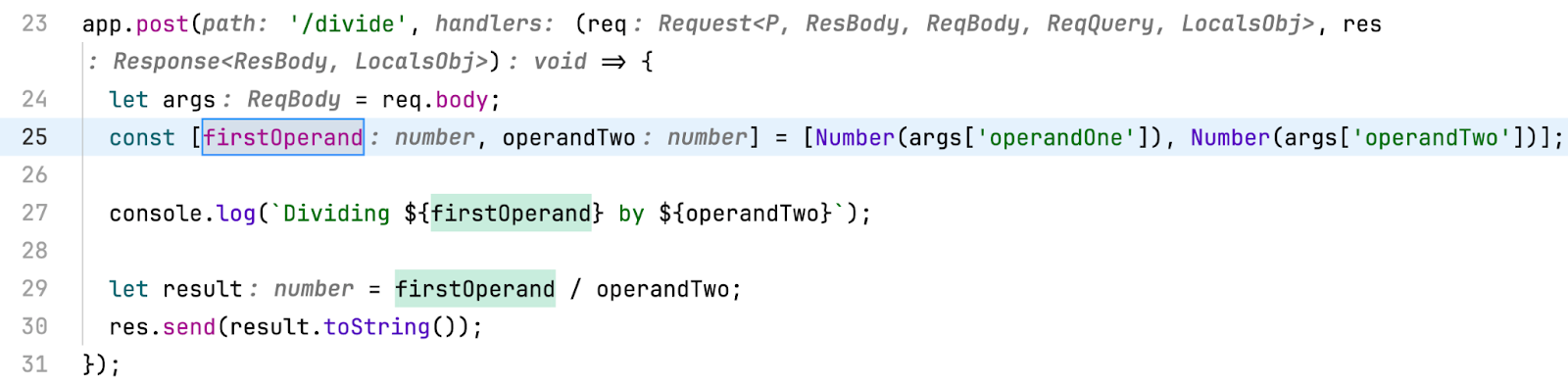
Smart mode in Fleet is powered by a backend engine. This engine might be IntelliJ, Resharper, or some LSP server implementation. Fleet itself is not responsible for implementing the features mentioned above. It’s just communicating them to the user.
And much more!
Fleet bundles AI Assistant for all the languages that Fleet supports. It can explain the code you’re working with (in my experience, this is useful even if you wrote it yourself!) or a commit from the Git history. Beyond this, AI Assistant can suggest refactorings and even generate commit messages, documentation, and, of course, tests and code (but please read the result before committing!).
Fleet has lightweight Git support and features a nice Diff tool window. Editing with multiple carets works like a charm. You can easily connect to a remote server via SSH or to a Docker container and work with the same functionality as on a local setup. Fleet is even getting the long-anticipated Markdown preview feature!
Summary
Many developers work with more than one programming language. Many software projects are implemented in more than one language. Many developers don’t like to spend their time installing add-ons and configuring them. Fleet is the answer, with a dozen programming languages supported out of the box and seamless integration with the most important software development tools, including AI assistance. Try exploring Fleet with your own polyglot software projects!