What is the Django Web Framework?
In this blog post, we’ll introduce you to web frameworks and explore what they are and why they’re vital if you want to develop apps rapidly without compromising on security. More importantly, we’ll explain exactly what the Django web framework is – an open-source web framework for building web applications using Python. Whether you’re a beginner or an experienced developer or migrating from a different framework, join us to dive into the details of the Django framework.
What is a web framework?
According to Wikipedia, “a web framework (WF) is a software framework designed to support web application development, including web services, web resources, and web APIs.”
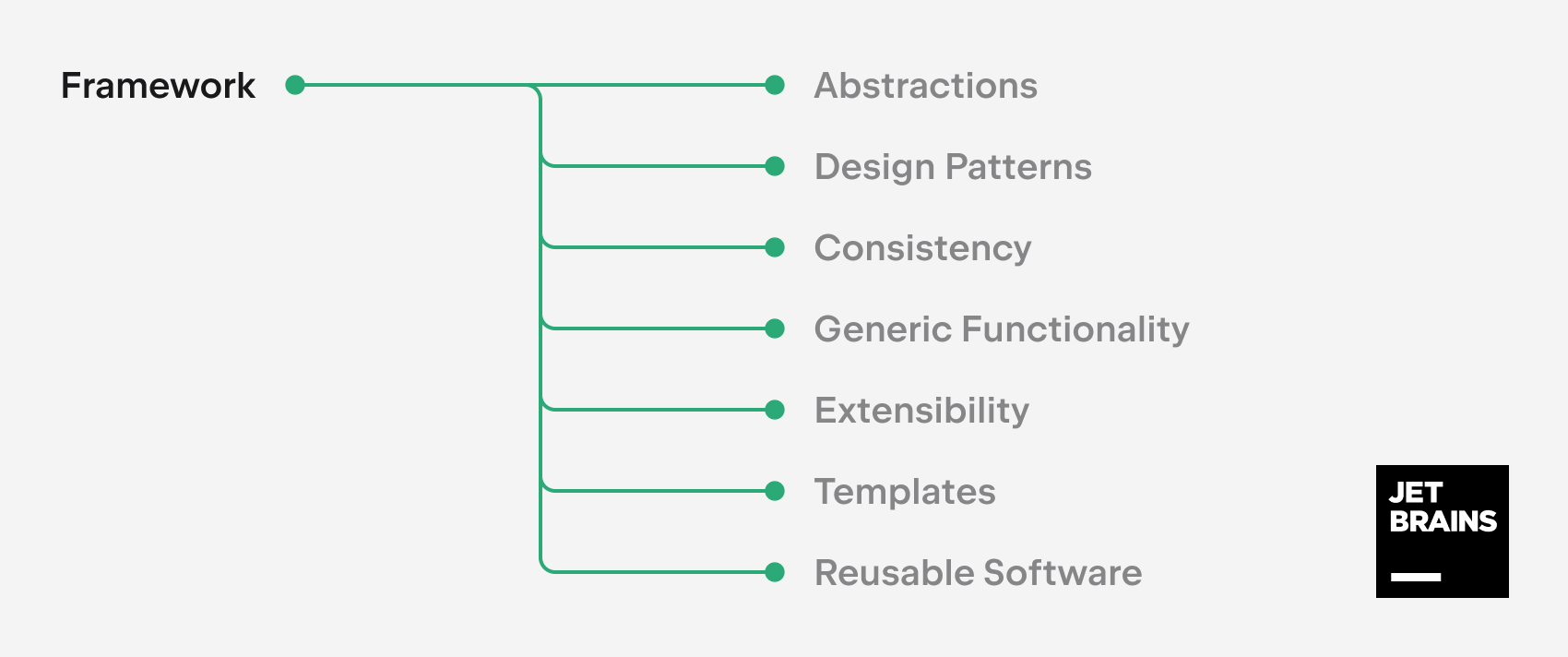
We often refer to frameworks as packages that come complete with established best practices, tools, testing resources, and libraries, greatly simplifying the process of creating web applications or services.
Using a web framework helps you build websites more easily because it provides a defined structure. Even if you switch between different web frameworks, most of them use a common design pattern called MVC (Model-View-Controller), making it easier for you to adapt and learn new ones.
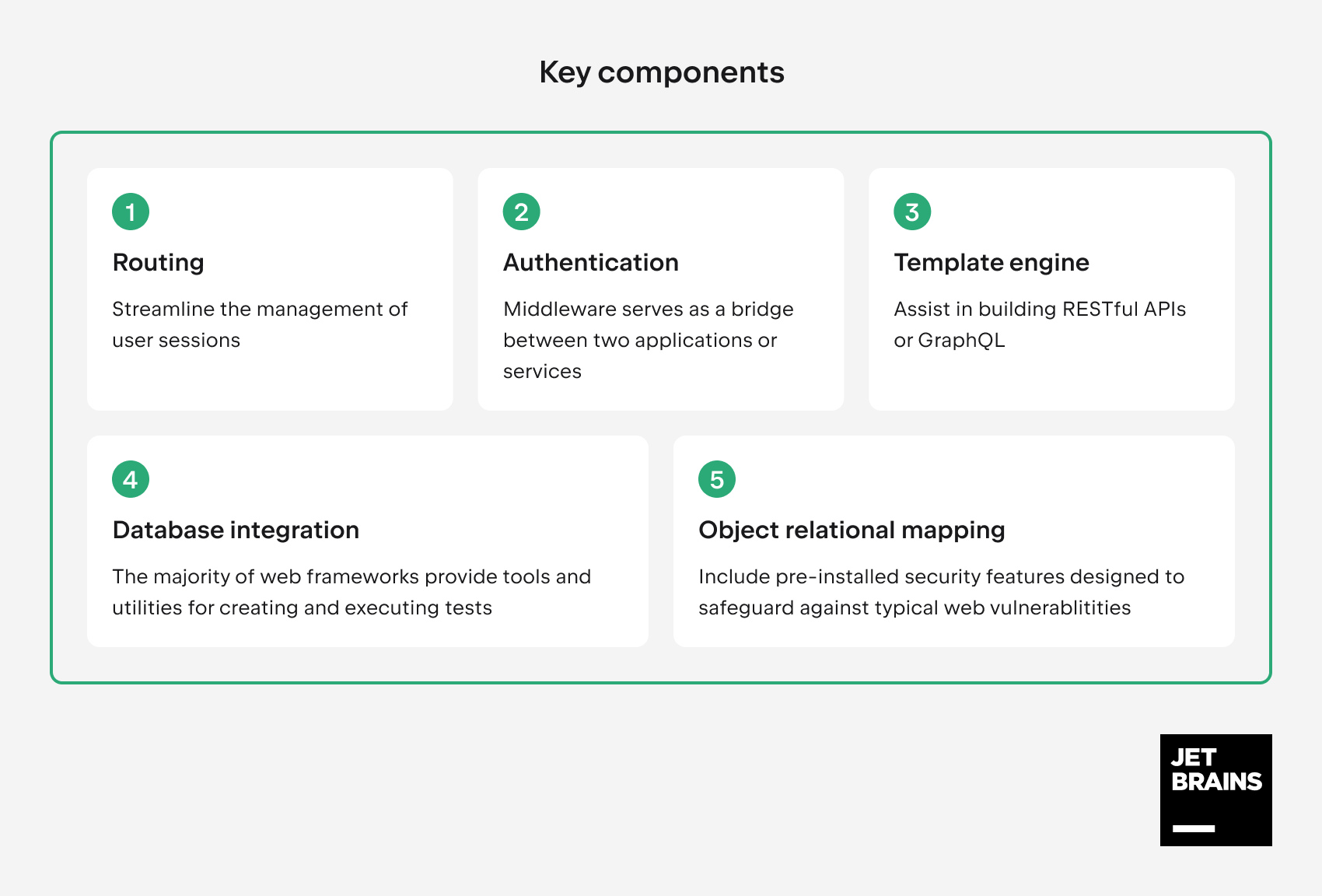
The key components and features of a web framework typically include:
1. Routing: A routing system is a core component of web frameworks that associates URLs with specific code or controller functions, simplifying the process of specifying how various segments of a web application react to incoming requests.
2. Request and response handling: This system is common to many frameworks and streamlines the management of HTTP requests and responses, enabling developers to concentrate on application logic rather than getting bogged down in low-level network tasks.
3. Template engine: Many frameworks come with a templating engine to segregate the presentation layer (HTML) from the application logic, aiding in the upkeep of a neat and well-structured codebase.
4. Database integration: This common framework feature provides tools and libraries for connecting with various types of databases (both relational and non-relational) and executing database operations, like querying, updating, and deleting data.
5. Authentication and authorization: These are key security capabilities present in many frameworks that authenticate users and control their authorizations, simplifying the implementation of user administration and access management using RBAC (Role Based Access Controls).
6. Middleware: Middleware serves as a bridge between two applications or services, facilitating their communication. Middleware components empower developers to incorporate functionality into the request/response process, encompassing tasks like logging, authentication, and more.
7. ORM (Object-Relational Mapping): Certain frameworks offer Object-Relational Mapping (ORM) tools that abstract database interactions, enabling developers to interact with databases through object-oriented code rather than relying on raw SQL queries, which allows you to develop faster while writing less code compared to raw SQL queries.
8. Test capabilities: The majority of web frameworks provide tools and utilities for creating and executing tests to guarantee the dependability and performance of the application.
9. Management of user sessions: Web frameworks frequently streamline the management of user sessions and states by offering built-in mechanisms for these tasks.
10. Security features: Many web frameworks include pre-installed security features designed to safeguard against typical web vulnerabilities, including cross-site scripting (XSS) and SQL injection.
11. RESTful API/GraphQL support: Many modern web frameworks provide valuable assistance in constructing RESTful APIs or GraphQL endpoints, simplifying the process of development and maintenance.
Developers choose web frameworks based on their programming language preferences, project requirements, and the specific features and conventions provided by the framework. These frameworks can save development time, enhance code maintainability, and promote best practices in web application development.
What is the Django framework?

Django is an open-source, free Python-based web framework that was launched in 2005 and is presently under the management of the Django Software Foundation (DSF).
Django has a proven track record of stability over eighteen years, which means you can rest assured that it will continue to be supported in the future.
Django simplifies the process of creating web applications with optimized code and fewer bugs.
Django’s primary goal is rapid web application development with clean, maintainable code. It follows the Don’t Repeat Yourself (DRY) principle, enhances security, and offers scalability. Its built-in admin interface simplifies data management. Extensible and well-documented, Django fosters a strong developer community, making it an efficient, secure, and popular web framework.
The core design philosophies of Django:
- Loose coupling
- Less code
- Quick development
- Don’t Repeat Yourself (DRY)
- Explicit is better than implicit
- Consistency
Loose coupling
Django emphasizes loose coupling, which means its components operate independently, with minimal knowledge of each other. This promotes modular, maintainable code.
Less code
Django apps should be concise, avoiding unnecessary boilerplate code. Django leverages Python’s dynamic features, like introspection, to optimize development.
Quick development
A web framework like Django helps speed up the routine parts of web development, making it much quicker.
Don’t Repeat Yourself (DRY)
Each piece of data should exist in just one place. Repeating things is not good, while keeping your code organized is. The framework should do more with less.
Explicit is better than implicit
This is a core Python principle listed in PEP 20 that states that Django shouldn’t be made to do too much work behind the scenes unless this helps maximize convenience without confusing new developers.
Consistency
Django aims for consistency, from the way we write Python code to how it feels to use the framework.
It demonstrates exceptional flexibility across various project types and industries, with companies such as Instagram, Disqus, Spotify, and more utilizing it at the production level.
Utilizing a framework can greatly accelerate the development process, enhance code quality, and decrease the chance of errors.
Take a look at this YouTube video from as far back as 2008, where the primary contributors are engaged in a discussion about Django’s technical design.
DjangoCon 2008 Panel: Django Technical Design
In it, the participants discussed various aspects of Django, its technical design, and potential improvements or changes to the framework. Some key points of discussion included:
1. The introduction of new contribs: There was a debate about whether certain Django applications should be categorized as “contrib” or placed elsewhere in the hierarchy, addressing concerns about making Django more accessible and flexible for different use cases.
2. Syndication framework limitations: Concerns were raised about the syndication framework’s flexibility when it comes to handling complex use cases, with several participants suggesting the need for improvements.
3. Timestamp fields: The panelists discussed the relevance and usefulness of timestamp fields in Django models and whether they should be included in the framework.
4. Django search integration: There was a conversation about incorporating the Django search functionality into Django contrib.
These are some of the topics discussed during the panel, and the conversation highlighted various technical and design considerations within the Django framework and its ecosystem.
The origins
Django originated in 2005, when a web development team initially tasked with building and maintaining newspaper websites embarked on a journey. As they constructed several websites, they found themselves identifying and refining numerous shared code snippets and design principles. Over time, this collective wisdom coalesced into a versatile web development framework, and in July 2005, they released it to the public as the “Django” project.
Django’s progression and enhancement have been ongoing ever since. With every new release, it has introduced fresh features and addressed issues, encompassing support for additional database types, template engines, and caching mechanisms.
The Django Software Foundation (DSF)
The Django Software Foundation (DSF) is a non-profit organization that develops and maintains Django, a free, open-source web application framework.
The foundation’s goals are to:
- Support the development of Django by sponsoring sprints, meetups, gatherings, and community events.
- Promote the use of Django among the World Wide Web development community.
- Protect the intellectual property and the framework’s long-term viability.
- Advance the state of the art in web development.
The DSF presents an annual award as a tribute to Malcolm Tredinnick, an early Django contributor. This monetary prize is awarded to the individual who best exemplifies the essence of Malcolm’s contributions – someone who embraces, aids, and encourages newcomers, generously offers guidance and support to fellow members, and contributes to the expansion of the community.
5 benefits of using Django
Django follows the “Batteries included” approach, offering an extensive set of features that developers require right from the beginning. Since all of the necessary components are integrated into a single package, they operate harmoniously and maintain uniform design principles.
Versatile
Django has proven its versatility by serving as the foundation for a wide array of web applications, including but not limited to content management systems, e-commerce platforms, social networks, and multi-tenant applications. It seamlessly integrates with various client-side frameworks.
Offering an extensive array of built-in functionality options, including support for multiple popular databases (MySQL, Postgres, MariaDB, etc.) and templating engines, Django also provides the flexibility to incorporate other components as necessary.
Secure
Django aids developers in steering clear of prevalent security blunders by offering a framework meticulously designed to automatically safeguard websites with best practices.
This framework incorporates robust defenses against prevalent security threats such as XSS (cross-site scripting) and CSRF (cross-site request forgery) attacks, SQL injections, clickjacking, and more.
Scalable
Django’s standout feature lies in its inherent scalability. Unlike other web frameworks, Django is purpose-built to manage substantial traffic and extensive data volumes from the beginning. Whether you’re crafting a modest blog or a sprawling online e-commerce platform, Django empowers your application to expand and adapt to your specific requirements.
Maintainable
Django follows the Don’t Repeat Yourself (DRY) principle, encouraging maintainable and reusable code to reduce code duplication. It also encourages the organization of related features into reusable “applications” and, at a more granular level, it groups related code into modules, following a structure reminiscent of the Model-View-Controller (MVC) design pattern.
Portable
Django grants you the freedom to deploy your applications across various operating systems like Linux, Windows, and macOS without being confined to a specific server platform. Additionally, Django enjoys robust support from numerous web hosting providers who frequently furnish tailored infrastructure and guidance for hosting Django-based websites like Fly.io, AWS LightSail, and others.
Django Tutorial: How to Set Up Your First Django Project
Get Your Django Project Rolling in One Click with PyCharm – a Python-Optimized IDE!
If you’re looking to get your Django project up and running quickly, make PyCharm, a Python-optimized IDE, your go-to tool.
Follow this in-depth tutorial:
Short on time? Kick off your Django journey in no time!
If you’re up for a challenge, here’s a detailed step-by-step guide to setting up your first Django project.
- Create a virtual environment
python3 -m venv <virtual_env_name>
2. Activate the environment
source <virtual_env_name>/bin/activate
3. Install the Django framework
pip install Django
4. Create a new project
django-admin startproject helloworld
Running your Django application
Get inside the helloworld directory and run the following command:
cd helloworld
python manage.py runserver
The application is up and running smoothly. Now, go to your web browser and access http://127.0.0.1:8000.
You should then see Django’s default welcome page. 🚀
PyCharm and Django: Out of the box
If you haven’t yet used PyCharm for Django development, then you definitely should, as it provides out-of-the-box support for Django.
Supercharge your development speed with Django tutorials curated by the PyCharm team!
Django’s core concept is rapid application development, and mastering it significantly shortens the journey from idea to production-ready web app. For even faster development, this tutorial in PyCharm teaches you to create a Django app. The tutorial covers creating a simple Django application that displays your local air temperature and allows you to explore weather conditions in random locations. It includes creating a Django project in PyCharm, writing models, views, and templates, making API calls, processing responses, and connecting to databases in order to populate them.
This post covers materialized views in Django. After reading it, you’ll know how to create and integrate materialized views into Django models, refresh them as required with the DROP statement, and utilize unique indexes to enhance performance.
This tutorial guides you through building and running applications in both local Kubernetes clusters and Google Kubernetes Engine using Cloud Code and PyCharm. It covers setting up a development environment, installing essential tools, creating projects, writing REST APIs with Django, and deploying applications on Google Kubernetes Engine.