.NET Tools
Essential productivity kit for .NET and game developers
Escape Character, Extract Common Code, Params Modifier, Out Vars – C# Language Support in 2024.2
Our release for ReSharper and Rider 2024.2 is just around the corner, and we have lots of exciting features shipping for the new C# 13 and current C# and VB.NET! Since there are so many, we will split them into multiple blog posts. So make sure to check those posts out as well!
In this series, we will cover features around:
- Escape Character, Extract Common Code, Params Modifier, Out Vars
- Equality Analysis, Ref Structs, Culture Previews, Using Directives
- Cast Expressions, Primary Constructors, Collection Expressions, List Patterns
Make sure to download the latest version of ReSharper or Rider to follow along:
Escape Character Support
With C# 13, we are getting a new control sequence for the ESC character. Previously, you had to use the hexadecimal escape sequence \0x1B
to express this character, while now, you can simply use \e
. This is especially attractive for virtual terminal sequences, which you can use to apply text formatting to console applications (including colored, bold, and blinking text).
In 2024.2, we are adding support for the new escape character and allowing you to easily migrate your codebase. As with some other features in this blog post, we’ve been looking more closely at what other areas in our language support could be improved along the way. So with the \e
support, we generalized the simplification inspection to identify other escaping shortcuts, such as \t
or \n
, to keep your string literals in the most “canonical” form:
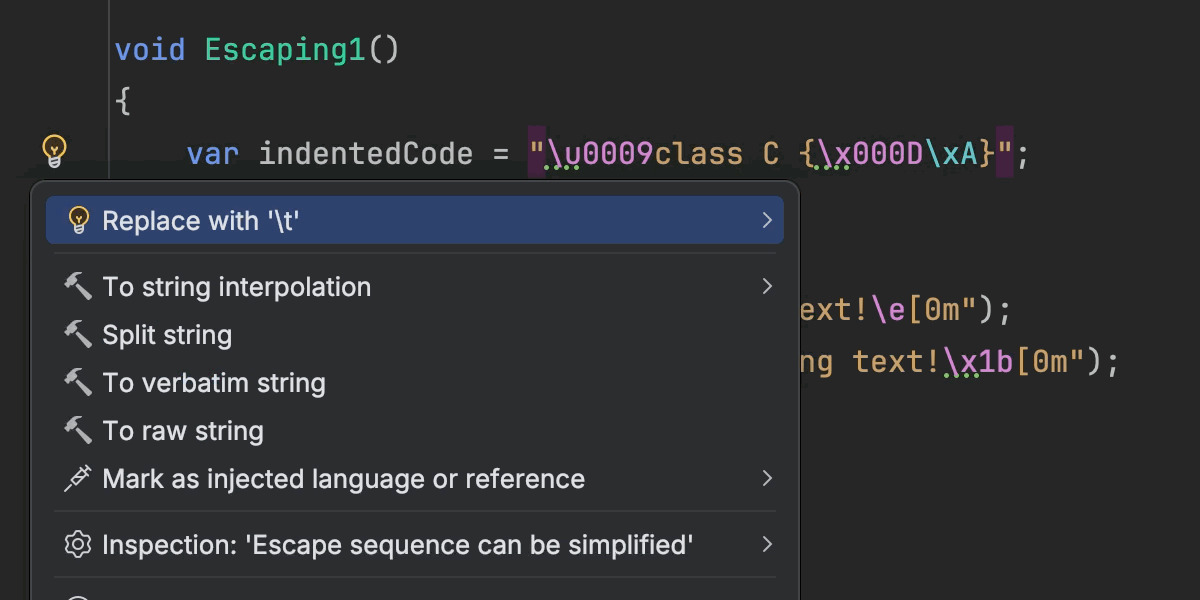
To enhance the handling of escape sequences in C#, we’ve added a whole set of context actions that allow you to switch between different representations, such as plain text, ASCII text (where non-ASCII characters are replaced with escape sequences), or a sequence of \u
and \x
Unicode codes. These actions can be applied to escape sequences, entire string literals, or selected character sequences. For instance, if you want to include a non-ASCII symbol in a string literal while maintaining ASCII encoding in the source code, you can use the new Convert to ASCII text action:
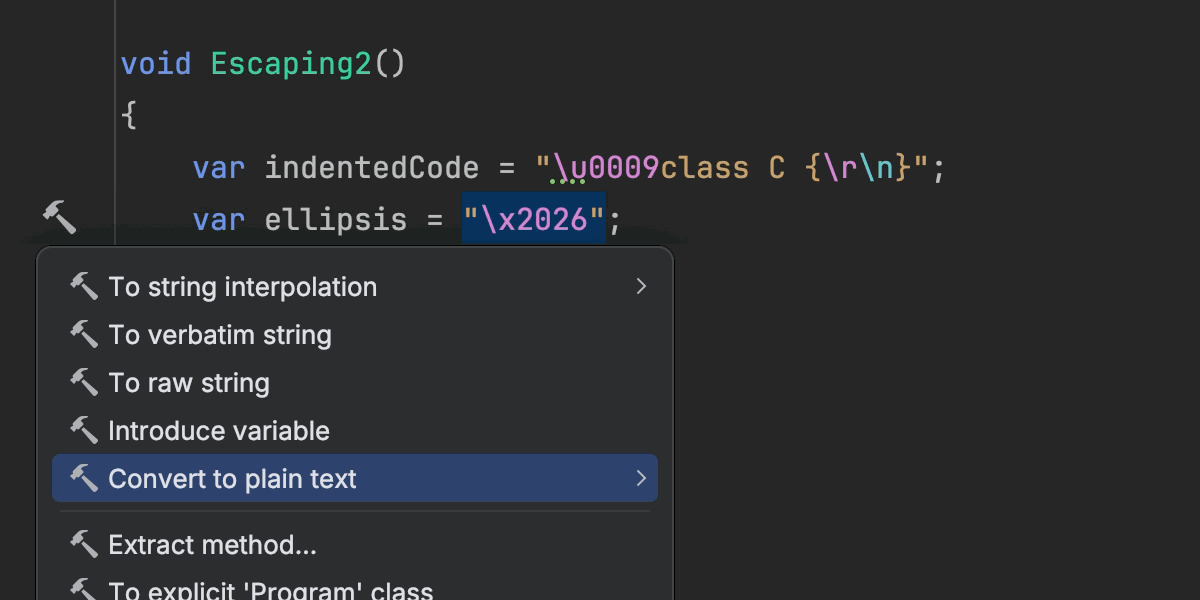
Those actions are also useful for uncovering the code points of individual characters. For example, the following two string literals are rendered equally in the editor, but are actually represented by different code sequences:
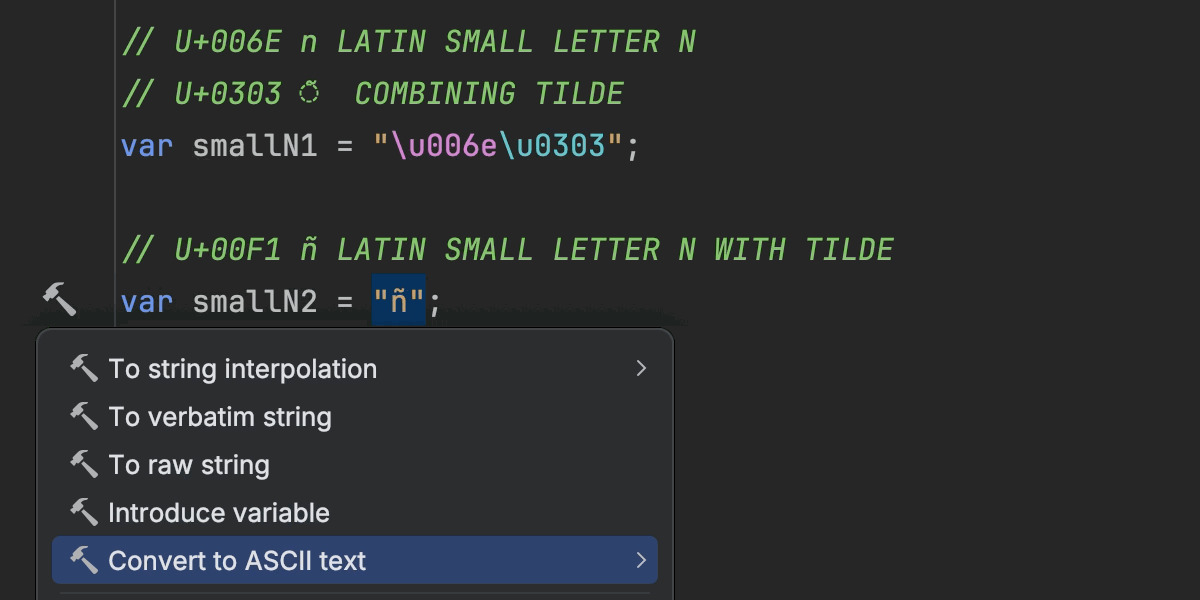
Furthermore, we are now warning about ordinary \x
escape sequences followed by ASCII letters. Unlike in other programming languages, the \x
escaping sequence in C# allows a variable number of hexadecimal characters to follow the prefix (only \u
requires exactly 4 hex numbers). That means you could accidentally attach characters to the sequence. The new Avoid mixing variable-length escape sequences and text inspection helps you spot such cases:
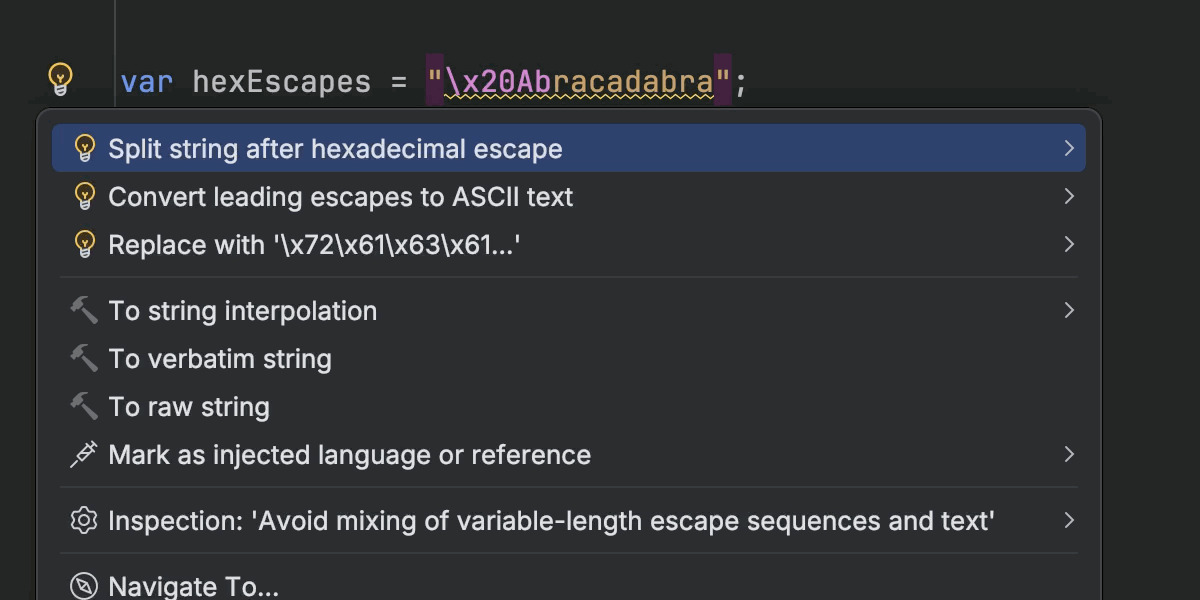
Extract Common Code
As you hack your way through codebases, it’s possible that you end up with small to large code duplications. Wouldn’t it be nice if your IDE told you about them?
var sb = new StringBuilder();
var version = NuGetVersion.Parse("1.3.3.7").Version;
if (version.Major >= 5)
{
sb.Append("?view=net-");
// duplicated from here
sb.Append(version.Major).Append(".").Append(version.Minor);
}
else
{
sb.Append("?view=netcore-");
// duplicated from here
sb.Append(version.Major).Append(".").Append(version.Minor);
}
In 2024.2, we are introducing a new code inspection Extract Common Code to analyze code inside branching constructs, more specifically if
and switch
statements. This new inspection can detect same code in the beginning and ending of all branching execution paths:
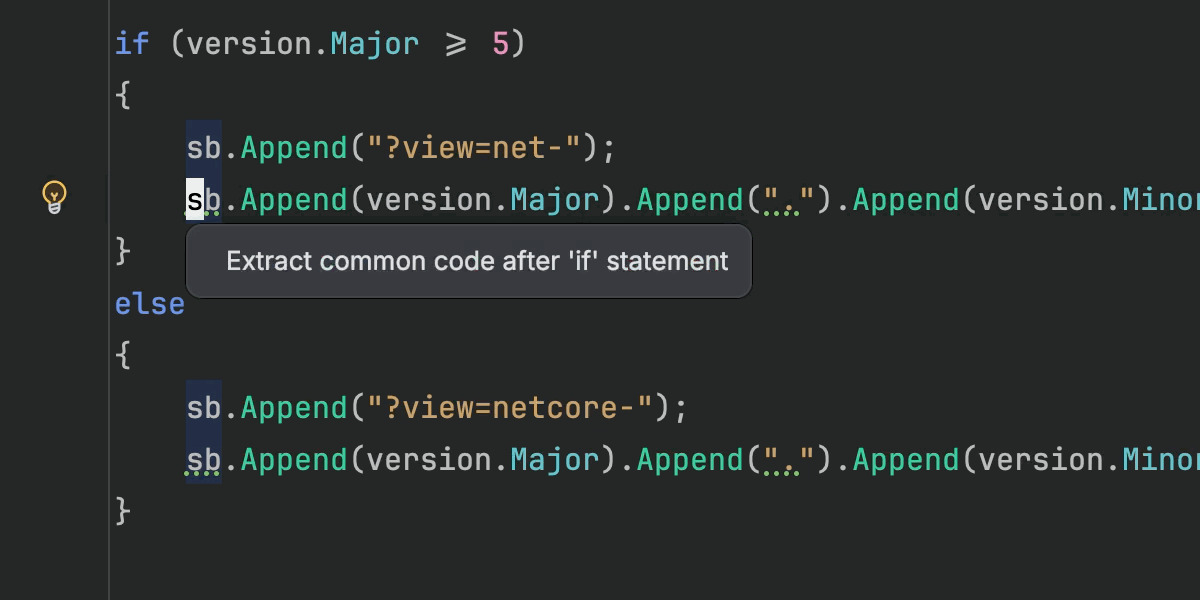
More importantly, the inspection can also help you catch bugs! Often the equivalent code in branching constructs is the result of copy & pasting code around. The inspection will bring that up to your attention, hinting that the code was supposed to be different. For an easy win, you can hit Alt-Enter
and look for the Run Inspection By Name action and find all occurrences in your codebase.
Params Modifier Refactoring
While we recently investigated support for collection expressions, we’ve also revisited related language features such as the params
modifier, which has been available in C# from day 1. When writing an API, you may not immediately know that you can take advantage of the params
modifier. On the other hand, once you know it, plenty of code may already have been written. A short example:
void M(string text, Type[] types)
{
M("plain array", new[] { typeof(int) });
M("collection expression", [ typeof(int) ]);
}
With 2024.2, we are introducing the Add/Remove ‘params’ modifier and update usages context actions for C# and VB.NET to improve your API ergonomics and update all usages in one go:
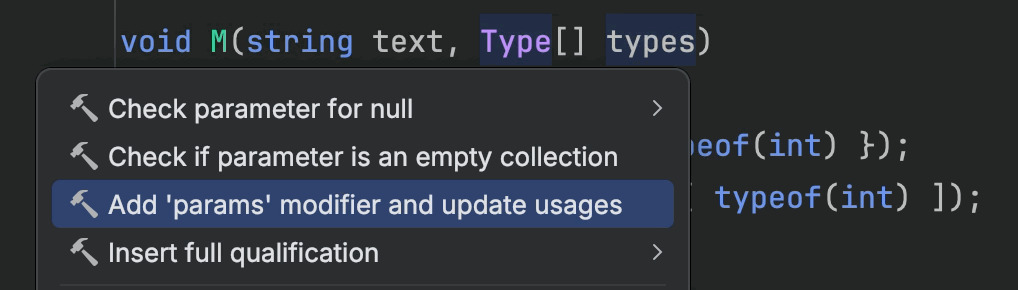
Out Variable Code Completion
Since C# 7, you can use out
variable declarations, which significantly reduce ceremony when calling methods with out
parameters. Not only can you capture values right from the argument, but you can also discard them if only the method return value is of importance.
In 2024.2, we decided to finally address this new syntax in our code completion – including smart naming suggestions! The quickest way for you is to use the ovv
shorthand:
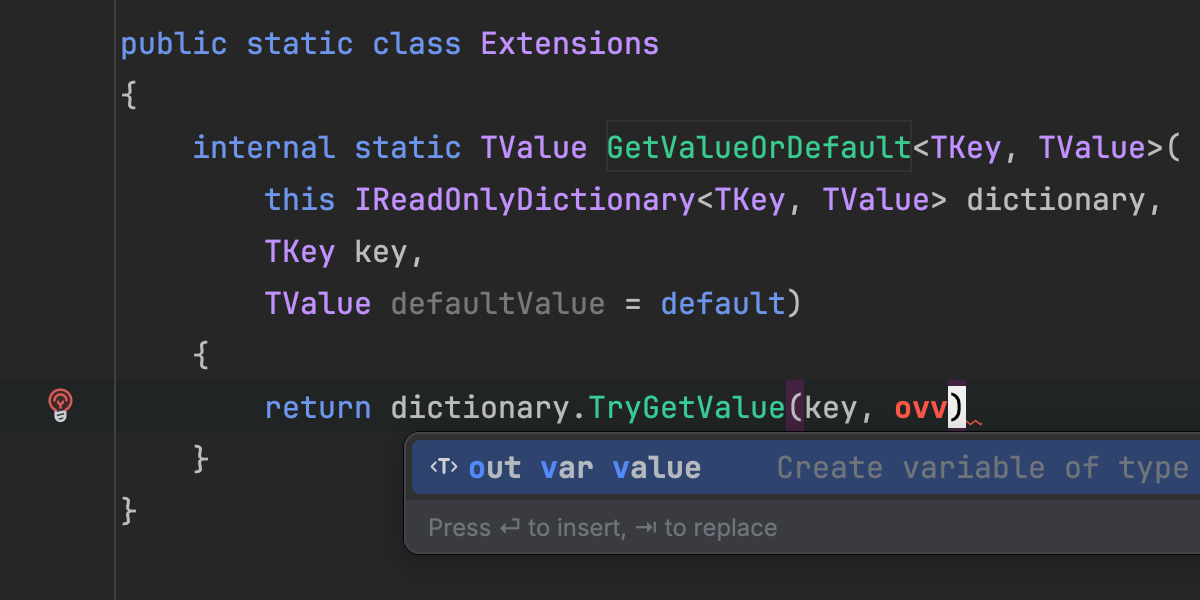
In some cases, the out var
argument must have an explicit type annotation to help with overload resolution. In this case, our code completion will provide you with all the possible types:
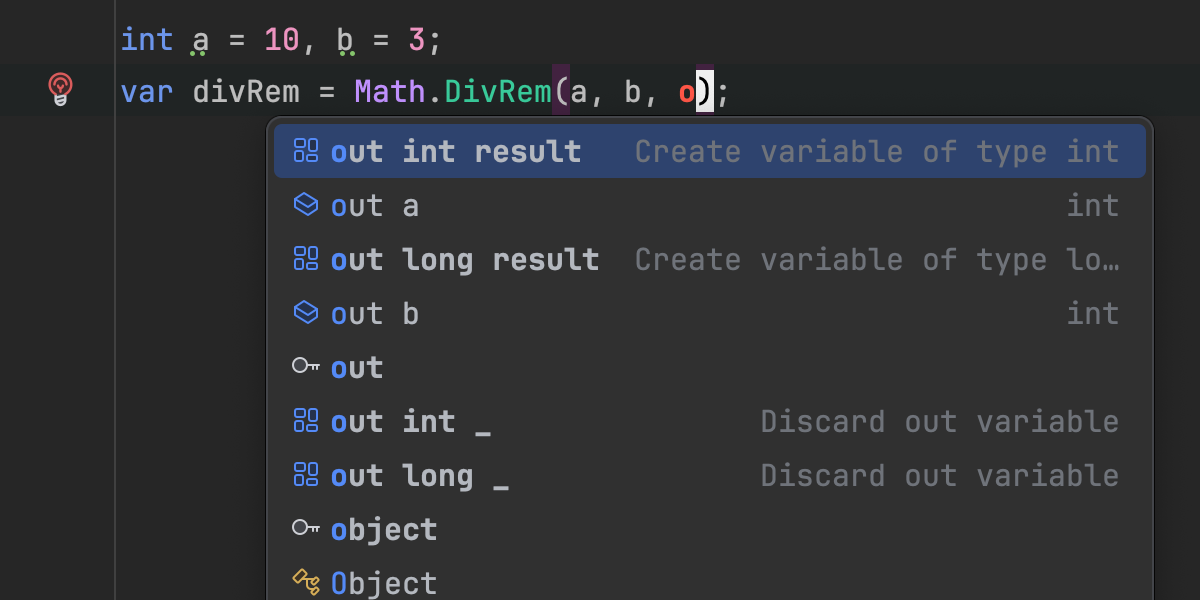
Conclusion
Give it a go with the latest ReSharper 2024.2 and Rider 2024.2, and let us know if you have any questions or suggestions in the comments section below.