.NET Tools
Essential productivity kit for .NET and game developers
.NET Annotated Monthly | January 2023
Did you know? Though these terms are often used interchangeably, there is a difference between a parameter and an argument. A parameter is only the definition of something that’s passed into a function and is found in the function’s signature. Parameters usually define the name and type of allowed for the argument to use. The argument is the actual value that’s passed into the function in calling code.
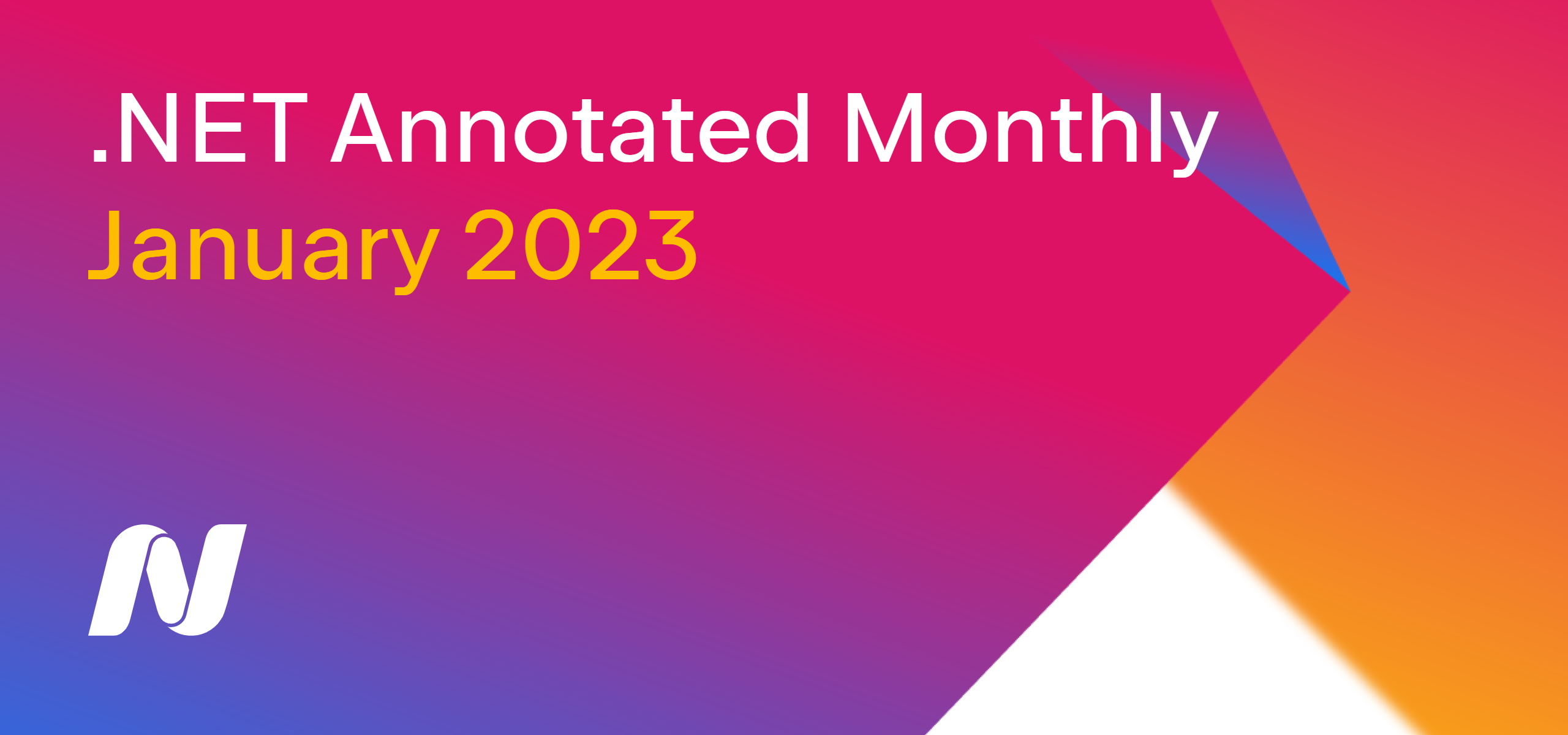
.NET news
Through December most of the .NET folks at Microsoft headquarters take time off for Christmas, New Year, and other winter holidays. There’s been no new releases from Microsoft, but there are a few noteworthy items in their Top 10 Blog Posts of 2022.
Featured content
We’d like to thank Chris Patterson for curating this month’s featured content! It’s story time!
Chris Patterson is an Enterprise Software Architect, the founder of Loosely Coupled, LLC, and the author of MassTransit, an open-source distributed application framework for building message-based applications. Before starting his own company, Chris spent 24 years leading the architecture and development of multiple platforms and services in a Fortune 10 company leveraging a broad range of technologies. Chris is a multi-year Microsoft MVP award recipient and regularly produces software development-related content on YouTube.
Avoid Breaking API Changes
Contract design is critical when building applications, even more so now that microservices are mainstream. Updating distributed applications requires discipline as these contracts define APIs, messages, and persistent data structures used by other services. Maintaining these contracts requires care to avoid breaking changes when releasing updated application versions.
There are numerous guidelines to avoid breaking contract changes, including not changing a property’s name, type, meaning, or cardinality and only adding nullable properties. However, there are less obvious ways that change can break an existing API, and the following is a true story of one such change.
A popular payment provider uses a string to identify customers in their API, and that identifier was always a numeric value. The API transfers data using JSON, which formats most data types as strings, so the customer identifier was assumed to be numeric. With that assumption, the engineering team used a numeric column to identify the customer in the database.
A few months ago, the webhook began receiving alphanumeric customer identifiers that immediately produced errors since the identifier could not be parsed into an integer. The payment provider changing the identifier format was not explicitly a contract change (it’s still a string), but it contradicted an established expectation. Customers treating the identifier as a numeric type were unable to process payments.
The provider reverted the behavior within a few hours.
The moral of this story: don’t change property expectations when developers may infer the content is a specific type. For example, if a text property contains a formatted number, Guid, DateTime, or TimeSpan, changing the format may cause unintended results, in this case breaking order fulfillment for new customers as they were unable to be stored in the database.
Tutorials and tips
.NET tutorials and tips
- Implementing Maps in .NET MAUI and Exploring Christmas UI in .NET MAUI – Keep learning MAUI with these posts by Leomaris Reyes.
- Method-Core Injection: a C# Pattern for Reducing Boilerplate Code – This post by Ann Lewkowicz contains some excellent recommendations for reducing time consuming yet not so high ROI boilerplate code.
- MAUI Sounds Amazing. Is It? – Well? Is it? This article by Lee Richardson details many ways MAUI makes for an excellent technology. What do you think? Will it work for you?
- C# 11.0: Raw String Literals – Strings are data types found in every language and in this post Thomas Claudius Huber is showing off C#’s newest string manipulation features.
- Colorful ASCII Christmas Tree in C# by ChatGPT – More holiday themed posts by Martin Zikmund – this time, fun! It’s a how-to on creating Christmas trees in C#.
- Add Busy Notifications to Your .NET MAUI Application – Give your users status updates in a progress bar or ring when your app is busy. Post by Selva Ganapathy Kathiresan.
- How to automatically generate SRI hash for client libraries in ASP.NET – SRI hashes are security features used to demonstrate that an API has not been tampered with. In this article, Valentin Anghel walks us through how to automatically generate them in our ASP.NET Core apps.
- Applying the CQRS Pattern in an ASP.NET Core Application – Using CQRS and Mediator patterns in .NET can help you organize your message-based systems nicely. In this post, Assis Zang details how to use these patterns in .NET apps.
- Web API Analyzers in ASP.NET Core – Muhammed Saleem writes about how you can use analyzers in your Web API code to generate the appropriate Swagger documentation to go with it.
- Generate YouTube Timestamp Links with C# 11 – This is a super useful post by Khalid Abuhakmeh showing us how to use C# to automate tasks related to YouTube.
- Technical Debt and C# Best Practices – Everyone has tech debt. What do you do with it? Here’s what Xavier Morera found to work the best for him, and some facts about time spent maintaining vs writing code.
- F# Tuple – Is it pronounced Tuple or Tuple? Jin Vincent explains in this article not just how to pronounce it – but he also covers all the important stuff that C# developers should know about Tuples. Or Tuples. Whatever, you’re going to keep pronouncing it the same anyway.
- Implementing Validations in .Net Core API – Baskar Rao has penned a tutorial on how to validate data in .NET Core.
- Visual Studio for Mac vs. Visual Studio Code vs. JetBrains Rider for new C# Developers – Here’s a balanced review by Costoda on those popular IDEs – Visual Studio, VS Code, and of course, Rider.
- Prefix your http requests without touching the actual HttpClient code – Josef Ottosson has found some techniques that work with Blazor when you need HTTP prefixes, such as when dealing with JWTs.
- Filtering in C# – How to Filter a List with Code Examples – Filtering lists is a basic function of programming. Get back to basics with Edeh Israel Chidera’s post.
- C# Advent Calendar 2022 – Matthew Groves has hosted several contributions to his yearly C# Advent Calendar. There’s lots of great “stocking stuffers” in here, go check them out.
- Error-accumulating composable assertions in C# – Mark Seemann shows some great ways to work with errors so that they don’t run amok.
- Refactoring – Fixing huge methods – “I like big methods and I cannot lie…” sang no developer, ever. So Henrique Dalcin Dalmas has penned this nice piece on tackling those huge blocks of code. Pro tip: Rider and R# are great tools to help cut method sizes.
- Array, List, Collection, Set, ReadOnlyList – what? A comprehensive and exhaustive list of collection-like types – Nice! Finally a nice rundown about different collections and similar objects in .NET. Know which is which and when to use them. Post by Steven Giesel.
- Logging and global error handling in .NET 7 WPF applications – Yep yep, we have to add logging so users can send error logs to us. Thomas Ardal demonstrates how to do this in .NET 7 WPF apps.
I occasionally see something like this when developers create SQL tables and use the string value “null” for nulls. Unfortunately, there are many negative side effects of this practice, including what’s in Joe’s tweet. Use nulls in a database the way the database creators intended. It will work better.
Related programming tutorials and tips:
- What are microservices? The pros, cons, and how they work – Anna Monus demonstrates the important things you need to know about microservices.
- Continuous Regression Testing—Pros, Cons & How It Works – Regression testing is a thing we often leave to QA, without much more thought about it. But it will add to the quality of your products if you know what’s gong on. Let Amy Reichert show you around the testing world.
- Don’t Panic: A Developer’s Guide to Building Secure GraphQL APIs – Melinda Gutermuth demonstrates how to build using GraphQL.
- Building Better UI Designs With Layout Grids – Grids, grids, grids. Grids are a great way to make a neat and clean UI that is organized and useful to customers. Nick Babich shows us how to make them better.
- .NET Core Best Practices – Vishal Shah writes about how TatvaSoft has found these familiar techniques that work as best practices for their engineering team. Many of these techniques are great and will work for you too, but you must read to find out.
Interesting and cool stuff
- What Rust Brings to Frontend and Web Development – Wait, Rust? This is a .NET newsletter! So what might .NET devs want to do with Rust? Maybe nothing, but it might be an interesting new option for frontend development. Post by Loraine Lawson.
- The LastPass disclosure of leaked password vaults is being torn apart by security experts – Here we go again. LastPass has been hacked (again), and The Verge is covering the security clown show. Check it out.
And finally, the latest from JetBrains!
Here’s a chance to catch up on JetBrains news that you might have missed:
Watch a recording of our ReSharper & Rider 2022.3 Release Party!
The .NET developer advocates are all on Mastodon now as well. Give them a follow!
Check out our .NET Guide! It’s a learning tool with tons of videos, tips, tricks, and info on a variety of .NET related topics.
Blog posts, webinars, etc..:
- Update on JetBrains’ Statement on Ukraine
- Overview of the 2022.3 Versions of All JetBrains IDEs and .NET Tools
- Rider 2022.3: Support for .NET 7 SDK, the Latest From C#11, Major Performance Improvements, and More!
- ReSharper 2022.3 Released With More C# 11 Features, NuGet Vulnerabilities Detection, and Support for Visual Studio ARM64.
- dotCover, dotMemory, dotPeek, and dotTrace 2022.3 Released!
- Introduction to MongoDB: Zero To Document Hero – Webinar Recording
- JetBrains Three Webinars on Game Development – Live Streams
- The Future of .NET with WASM
- Blazor Best Practices Borrowed From ReactJS – Webinar Recording
Don’t miss this fantastic offer! CODE Magazine is offering a free subscription to JetBrains customers. Get your copy today!
Sharing is caring! So share content that you find useful with other readers. Don’t keep it to yourself! Send us an email with your suggestions for publication in future newsletters!